FPGAs are well suited for precision motor control, and in this project we will create a simple motor control program upon which more complex applications can be built.
Required Hardware
introduce
We can control the motor with a simple 8-bit microcontroller, outputting a simple pulse width modulated waveform. However, when it comes to precision or advanced motor control, nothing beats the determinism and real-time response of an FPGA. The flexibility of the interface also makes it possible to control multiple motors from a single device, providing a more integrated solution.
First, we will learn a little about motor control theory and create a simple example. We all know that we can drive a DC motor and control its speed via a PWM signal. However, driving it efficiently and accurately requires a little more knowledge of motor control theory.
Motor
Believe it or not, one of my favorite subjects at university was Control Theory. In this module, we studied AC and DC motors, understanding both the theory and real-world use cases. There are various types of AC motors that are powered by an AC supply and can be divided into synchronous motors and induction motors. For example, AC motors are commonly used in pumps and compressors.
There are two types of DC motors: brushed and brushless. Of the two types, brushed are the easiest to drive because they require only one power source. In a brushed DC motor, brushes supply current to a commutator that is connected to the rotor and coils. The current induces an electric field in the coils that is repelled by an external magnet (stator). To ensure rotation, the commutator is designed so that the current flows in the opposite direction to ensure continuous rotation.
The second type of DC motor is the brushless motor, which is slightly more complicated to drive because they have no commutator. Instead, the magnets are mounted on the rotor and the coils are wrapped around the stator so that the current in the coils can be controlled and sequenced externally.
The easiest of the two to control is the brushed DC motor, so we'll use that as our example.
Pulse Width Modulation Drive
The theory behind driving a motor with PWM is that you can control the average voltage that the motor gets, and therefore its speed. When the PWM signal has a 100% duty cycle, the motor is at full voltage and runs at full speed. If you provide a 10% duty cycle, the motor will run at 10% of its full speed.
However, to run the motor efficiently, we need to determine the PWM period correctly. A DC motor has a series inductance and series resistance, which means the motor will act as a low pass filter. The frequency is reduced to
where the time constant is given by L/R - we can get these values from the motor datasheet.
Therefore, to ensure a stable speed, we need to choose a PWM frequency that is higher than the motor frequency cutoff to ensure that the DC component is observed.
Therefore, we want to choose a frequency that is at least 5 times the cutoff frequency.
FPGA
To get started with this project, we first create a hardware design targeting an FPGA board.
Start by creating a new project
Name your project
Select RTL project without specifying source
After creating the project, create a new block diagram
Pull the system clock onto the block diagram from the Board tab
Do the same for USB UART
Adding a MicroBlaze processor from the IP library
Run the block automation connection, select the local memory size as 32KB and uncheck the interrupt controller
Add AXI timer
Run Connection Automation
Open the Clock Wizard and deselect the Reset Input
Adding GPIO
Re-customize GPIO to 1 bit wide, output only
Select GPIO output and AXI timer PWM and set them to output
It should look like this when you are finished.
Once synthesis is complete, we can open the synthesis view and assign the IO to GPIO and timer output - for GPIO the pin is J1 and for PWM the pin is L2
Build the bitstream and export the platform
Vitis Design
Open Vitis, create a new application project and select the XSA you just exported.
Enter the project name
Choose independence
Create a new hello world application
The application software is very simple, we will configure the AXI timer according to the required PWM period and the desired duty cycle.
#include
#include "platform.h"
#include "xil_printf.h"
#include "xtmrctr.h"
#define TMRCTR_DEVICE_ID XPAR_TMRCTR_0_DEVICE_ID
#define PWM_PERIOD 1000000 /* PWM period in (500 ms) */
#define TMRCTR_0 0 /* Timer 0 ID */
#define TMRCTR_1 1 /* Timer 1 ID */
#define CYCLE_PER_DUTYCYCLE 10 /* Clock cycles per duty cycle */
#define MAX_DUTYCYCLE 100 /* Max duty cycle */
#define DUTYCYCLE_DIVISOR 2 /* Duty cycle Divisor */
XTmrCtr TimerCounterInst;
void display_menu()
{
//Clear the screen
xil_printf("�33[2J");
//Display the main menu
xil_printf("******************************************
");
xil_printf("**** www.adiuvoengineering.com ****
");
xil_printf("**** Motor Control Example ****
");
xil_printf("******************************************
");
xil_printf("
");
xil_printf("MM10 Motor Control
");
xil_printf("---------------------------------------------
");
xil_printf("
");
xil_printf("Select a Speed:
");
xil_printf(" (1) - Stop
");
xil_printf(" (2) - 25 %
");
xil_printf(" (3) - 33 %
");
xil_printf(" (4) - 50 %
");
xil_printf(" (5) - 66 %
");
xil_printf(" (6) - 75 %
");
xil_printf(" (7) - 100 %
");
xil_printf("
");
}
void set_pwm(u32 cycle)
{
u32 HighTime;
XTmrCtr_PwmDisable(&TimerCounterInst);
HighTime = PWM_PERIOD * (( float) cycle / 100.0 );
XTmrCtr_PwmConfigure(&TimerCounterInst, PWM_PERIOD, HighTime);
XTmrCtr_PwmEnable(&TimerCounterInst);
}
int main()
{
u8 Div;
u32 Period;
u32 HighTime;
char key_input;
u8 DutyCycle;
init_platform();
print("Hello World
");
print("Successfully ran Hello World application");
XTmrCtr_Initialize(&TimerCounterInst, TMRCTR_DEVICE_ID);
Div = DUTYCYCLE_DIVISOR;
XTmrCtr_PwmDisable(&TimerCounterInst);
Period = PWM_PERIOD;
HighTime = PWM_PERIOD / Div--;
XTmrCtr_PwmConfigure(&TimerCounterInst, Period, HighTime);
XTmrCtr_PwmEnable(&TimerCounterInst);
while(1){
display_menu();
read(1, (char*)&key_input, 1);
xil_printf("Echo %c
",key_input);
switch (key_input) {
case '1': //stop
XTmrCtr_PwmDisable(&TimerCounterInst);
break;
case '2': //25%
xil_printf("25%
");
DutyCycle = 25;
set_pwm(DutyCycle);
break;
case '3': //33%
DutyCycle = 33;
set_pwm(DutyCycle);
break;
case '4': //50%
DutyCycle = 50;
set_pwm(DutyCycle);
break;
case '5': //66%
DutyCycle = 66;
set_pwm(DutyCycle);
break;
case '6': //75%
DutyCycle = 75;
set_pwm(DutyCycle);
break;
case '7': //100%
DutyCycle = 100;
set_pwm(DutyCycle);
break;
}
}
cleanup_platform();
return 0;
}
Of course, the motor I chose contains two Hall Effect sensors. The direction of rotation can be determined by the output of one Hall Effect sensor located in front of another Hall Effect sensor.
Rotate clockwise
Counterclockwise rotation
We can use the pulse frequency to determine the speed of the motor, and we will look at this in more detail later in the project.
Previous article:What problems does BLDC solve? What are the advantages and disadvantages of BLDC motors?
Next article:Steps for configuring the PROFINET X2 interface in the S7-1200 project
Recommended ReadingLatest update time:2024-11-15 05:32
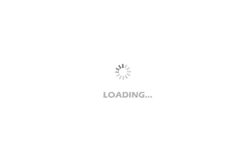
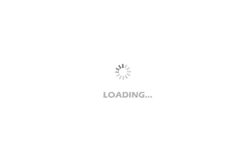
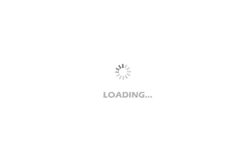
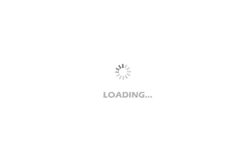
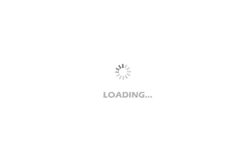
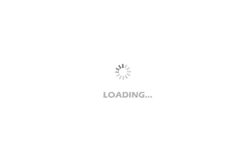
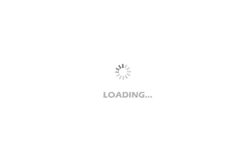
- Popular Resources
- Popular amplifiers
-
Summary of non-synthesizable statements in FPGA
-
Learn CPLD and Verilog HDL programming technology from scratch_Let beginners easily learn CPLD system design technology through practical methods
-
Single-chip microcomputer C language programming and simulation
-
Embedded high-speed serial bus technology - implementation and application based on FPGA
- Red Hat announces definitive agreement to acquire Neural Magic
- 5G network speed is faster than 4G, but the perception is poor! Wu Hequan: 6G standard formulation should focus on user needs
- SEMI report: Global silicon wafer shipments increased by 6% in the third quarter of 2024
- OpenAI calls for a "North American Artificial Intelligence Alliance" to compete with China
- OpenAI is rumored to be launching a new intelligent body that can automatically perform tasks for users
- Arm: Focusing on efficient computing platforms, we work together to build a sustainable future
- AMD to cut 4% of its workforce to gain a stronger position in artificial intelligence chips
- NEC receives new supercomputer orders: Intel CPU + AMD accelerator + Nvidia switch
- RW61X: Wi-Fi 6 tri-band device in a secure i.MX RT MCU
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- CGD and Qorvo to jointly revolutionize motor control solutions
- CGD and Qorvo to jointly revolutionize motor control solutions
- Keysight Technologies FieldFox handheld analyzer with VDI spread spectrum module to achieve millimeter wave analysis function
- Infineon's PASCO2V15 XENSIV PAS CO2 5V Sensor Now Available at Mouser for Accurate CO2 Level Measurement
- Advanced gameplay, Harting takes your PCB board connection to a new level!
- Advanced gameplay, Harting takes your PCB board connection to a new level!
- A new chapter in Great Wall Motors R&D: solid-state battery technology leads the future
- Naxin Micro provides full-scenario GaN driver IC solutions
- Interpreting Huawei’s new solid-state battery patent, will it challenge CATL in 2030?
- Are pure electric/plug-in hybrid vehicles going crazy? A Chinese company has launched the world's first -40℃ dischargeable hybrid battery that is not afraid of cold
- Implementing USB source code with Verilog (FPGA)
- How good is Hangshun's chip? Let's see if Huawei 5G uses its chip
- ESP-USB-Bridge turns the ESP32-S2/S3 development board into a JTAG debugger, and cooperates with OpenOCD to debug STM32 and other chips
- Analog Electronics Textbook Exercises
- Teach you step by step: Use STM32F103 to drive ST7567 LCD screen
- CCS
- Download "ADI Arbitrary Waveform Generator Solution" and get a gift!
- TTI and Molex live broadcast at 10 am today | Smaller, faster, more reliable connectors drive new developments in IoT applications
- MSP430 Timer Parameters
- 【NXP Rapid IoT Review】+First Online Project