1. What is the Systick tick timer?
The Systick timer is a system tick timer, a 24-bit countdown timer that automatically reloads the timing initial value from the RELOAD register when it counts to 0. As long as the enable bit in the SysTick control and status register is not cleared, it will never stop and can work even in sleep mode.
A timer is used to execute an action after a specified time.
Systick timer is often used for delay or heartbeat clock of real-time system. This can save MCU resources and avoid wasting a timer. For example, in UCOS, time-division multiplexing requires a minimum timestamp. Generally, in STM32+UCOS system, Systick is used as UCOS heartbeat clock.
------------------------------------------------------------------------------------------------------------------------------------------------------
2. Systick is generally used for delay and precise delay.
There are 4 Systick registers in total
CTRL SysTick Control and Status Register
LOAD SysTick automatically reloads the initial value register
VAL SysTick current value register
CALIB SysTick Calibration Value Register
------------------------------------------------------------------------------------------------------------------------------------------------------
3. Systick timer principle
This timer sets an initial value, and then decrements the initial value to 0, which means the timing is completed. After completion, an interrupt can be generated or not.
Bit 0 is the enable bit, which can enable or disable the timer
The first bit is the interrupt enable bit, which determines whether an interrupt is generated after the subtraction is completed.
The second bit is the clock selection bit, which can select external or internal clock as the clock source.
The 16th bit is the flag bit. After the number is subtracted to 0, this bit is set to 1 and is automatically cleared after being read.
------------------------------------------------------------------------------------------------------------------------------------------------------
4. uSysTick reload value register-LOAD
When the current value register is decremented to 0, the value of RELOAD will be automatically assigned to the current value register.
if(VAL == 0)
VAL = RELOAD
------------------------------------------------------------------------------------------------------------------------------------------------------
5. SysTick Current Value Register - VAL
VAL is counted down from the initial value (RELOAD) by one, and when it reaches 0, the initial value (RELOAD) is reassigned to VAL.
------------------------------------------------------------------------------------------------------------------------------------------------------
6. Implementation of tick timer
For STM32, the external clock source is 1/8 of HCLK (AHB bus clock) and the core clock is HCLK clock
1. Select the clock source
SysTick_CLKSourceConfig(); //Select the clock source in the misc.c file
SysTick_Config(uint32_t ticks) //Initialize systick, set the clock to HCLK, and enable interrupt in core_cm4.h file
2. Write a delay function
3. Set interrupt priority grouping
NVIC_PriorityGroupConfig( NVIC_PriorityGroup_2);
Generally, when using interrupts, we need to group our priorities at the beginning, and then set the priorities after the grouping.
Try not to change groups frequently in the same program. Frequent switching of groups may cause unexpected errors in the program.
------------------------------------------------------------------------------------------------------------------------------------------------------
7. Use interrupts to implement delay
Systick interrupt service function: void SysTick_Handler(void);
-----------------------------------------------------------------------------------------------------------------
Notice:
In uCortex-M system, Systick code can be used universally.
If you find that the delay is inconsistent during use, the problem is usually caused by different core clocks. You can modify the ticks value.
------------------------------------------------------------------------------------------------------------------------------------------------------
The reference code is as follows:
void init_delay(void)
{
/*Our external crystal oscillator is 8MHz, and then multiplied to 168M, then the Systick clock is 21M, which is the Systick counter
Every time VAL decreases by 1, it means 1/21us has passed*/
//1. Select the clock source (select external clock)
//External clock needs to be /8, internal clock is never used
SysTick_CLKSourceConfig(SysTick_CLKSource_HCLK_Div8);
}
//2. Write a delay function
//The maximum delay does not exceed 798.915ms, which is (2^24)/21/1000
void delay_us(u32 nus)
{
u32 temp = 0;
//1. Realize a delay of 1us*nus
//21 is the external clock frequency, if you choose the internal clock it is 168M/8
SysTick->LOAD = 21*nus; //Set the automatic load value to 21 (1us)
SysTick->VAL = 0x00; //Set the current initial value to 0
SysTick->CTRL |= SysTick_CTRL_ENABLE_Msk; // Turn on (enable) counting: enable SysTick timer
do
{
//Read the control register
temp = SysTick->CTRL;
}while(!(temp & (1<<16)));//Wait for the count time to arrive (bit 16)
SysTick->CTRL &= ~SysTick_CTRL_ENABLE_Msk; // Disable count
SysTick->VAL = 0x00; //Set the current initial value to 0 and reset VAL
}
void delay_ms(u32 nms)
{
u32 temp = 0;
//1. Realize a delay of 1us*nus
SysTick->LOAD = 21000*nms; //Set the automatic load value to 21 (1us)
SysTick->VAL = 0x00; //Set the current initial value to 0
SysTick->CTRL |= SysTick_CTRL_ENABLE_Msk; // Turn on (enable) counting
do
{
//Read the control register
temp = SysTick->CTRL;
}while(!(temp & (1<<16)));
SysTick->CTRL &= ~SysTick_CTRL_ENABLE_Msk; // Disable count
SysTick->VAL = 0x00; //set the current initial value to 0
}
void delay_s(u32 s)
{
while(s--)
{
delay_ms(500);
delay_ms(500);
}
}
Previous article:STM32 serial port (UART) and serial communication principle
Next article:STM32 clock system architecture
Recommended ReadingLatest update time:2024-11-15 11:36
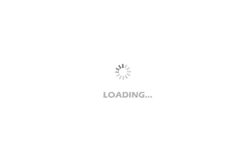
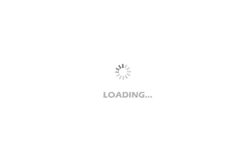
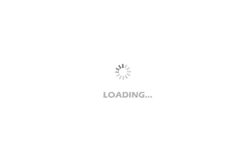
- Popular Resources
- Popular amplifiers
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Melexis launches ultra-low power automotive contactless micro-power switch chip
- Melexis launches ultra-low power automotive contactless micro-power switch chip
- Molex leverages SAP solutions to drive smart supply chain collaboration
- Pickering Launches New Future-Proof PXIe Single-Slot Controller for High-Performance Test and Measurement Applications
- Apple faces class action lawsuit from 40 million UK iCloud users, faces $27.6 billion in claims
- Apple faces class action lawsuit from 40 million UK iCloud users, faces $27.6 billion in claims
- The US asked TSMC to restrict the export of high-end chips, and the Ministry of Commerce responded
- The US asked TSMC to restrict the export of high-end chips, and the Ministry of Commerce responded
- ASML predicts that its revenue in 2030 will exceed 457 billion yuan! Gross profit margin 56-60%
- Detailed explanation of intelligent car body perception system
- 46% of open source maintainers are unpaid, and 26% earn more than $1,000 per year
- How to start learning analog electronics
- I would like to ask about the AMS1117 and LM2596S chips
- See good people and emulate them, Zhu Ge is full of positive energy^_^
- [Mil MYS-8MMX] Mil MYS-8MMQ6-8E2D-180-C Unpacking Report 2 - Power on
- MSP430 MCU timer TA interrupt program
- Free review: Domestic FPGA Anlu SparkRoad development board
- Replaying MicroPython on STM32F7DISC - DCExpert
- Problems in PCB design
- Please share the installation package of modelsim10.4 or other versions