Introduction: There is a clock switching function inside the STM8, which can change the clock frequency when needed. The board is STM8_Discovery, and the LED is PD0.
The procedure is as follows:
//Function: STM8 internal clock frequency division switch
#include "iostm8s105c6.h"
// Function: Delay function
// Input parameter: ms -- the number of milliseconds to delay, assuming the CPU frequency is 2MHZ
void DelayMS(unsigned int ms)
{
unsigned char i;
while(ms != 0)
{
for(i=0;i<250;i++)
{
}
for(i=0;i<75;i++)
{
}
ms--;
}
}
//GPIO port initialization
void GPIO_init(void)
{
PD_DDR = 0x01; //Configure the direction register PD0 output of the PD port
PD_CR1 = 0x01; //Set PD0 to push-pull output
PD_CR2 = 0x00;
}
// Clock initialization
void CLK_init(void)
{
CLK_SWR = 0xE1; //Select the 16MHZ RC oscillator inside the chip as the main clock
}
main()
{
int i;
GPIO_init();
CLK_init();
while(1) //Enter an infinite loop
{
//Set the CPU clock divider below so that CPU clock = main clock / 4
//Through the LED, we can see that the speed of the program has indeed dropped significantly
CLK_CKDIVR = 0x1A; // Main clock = 16MHZ/8 = 2MHz
//CPU clock = main clock / 4 = 500KHZ
for(i=0;i<10;i++)
{
PD_ODR = 0x01;
DelayMS(100);
PD_ODR = 0x00;
DelayMS(100);
}
// Set the CPU clock divider below so that the CPU clock = the main clock
CLK_CKDIVR = 0x0A; // Main clock = 16MHZ/2 = 8MHz
//CPU clock = main clock/4 = 2MHZ
for(i=0;i<10;i++)
{
PD_ODR = 0x01;
DelayMS(100);
PD_ODR = 0x00;
DelayMS(100);
}
}
}
Previous article:Clock system of STM8 microcontroller
Next article:STM8 realizes perpetual calendar (highlight time adjustment)
Recommended ReadingLatest update time:2024-11-16 19:39
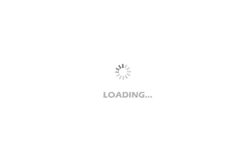
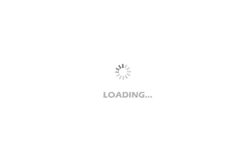
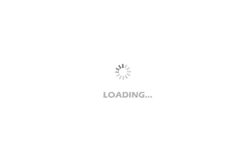
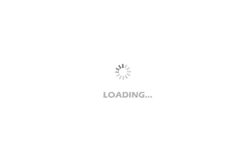
- Popular Resources
- Popular amplifiers
-
STM8 C language programming (1) - basic program and startup code analysis
-
Description of the BLDC back-EMF sampling method based on the STM8 official library
-
Signal Integrity and Power Integrity Analysis (Eric Bogatin)
-
STM32 MCU project example: Smart watch design based on TouchGFX (8) Associating the underlying driver with the UI
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Prize live broadcast: TI low-power MCU products and Zigbee wireless solutions are now open for registration~
- FPGA_100 Days Journey_VGA Design
- CCS Basics Tutorial
- Design of wireless network sensor based on Bluetooth protocol
- MCU experts, do I need to modify the USB virtual serial port program generated by STM32cubeMX?
- 2021 ST Industrial Summit Tour starts to reserve seats: "Motor Drive, Power Supply, Industrial Automation" + "New Products, Courses, Training"
- Happy Dragon Boat Festival, salty rice dumplings, sweet rice dumplings, which kind do you like?
- [Topmicro Intelligent Display Module Review] 5. Development Board NUCLEO-F746ZG Communication with Intelligent Display Module
- TI MCU has launched a new product! August live broadcast reveals new features video replay summary!
- How to safely measure high voltage with a LOTO oscilloscope?