In addition to collecting and comparing electric potential, it is also necessary to collect, calculate and process analog signals such as bus voltage, bus current, heat sink temperature, potentiometer, etc.
This priority processing is very important. However, ST wrote the program very well, at least I think so. It puts different tasks into accurate time periods for collection and processing.
First post its core AD collection and processing and then analyze it.
#ifdef SENSORLESS
#endif
I changed the above code a little bit, that is, to collect one more average current.
There are two types of AD acquisition, one is synchronous and the other is asynchronous. There are three acquisition channels in synchronous and three acquisition channels in asynchronous. The channels in synchronous are back EMF channel, instantaneous current, and average current. The channels in asynchronous acquisition are bus voltage, temperature value, and potentiometer.
Asynchronous acquisition is performed after synchronization is completed. Synchronous acquisition is triggered by channel 4 of TIM1.
Therefore, two analog signals are collected in each PWM cycle. The asynchronous collection channel has nothing to do with the ON and OFF states of PWM, so it is arranged in asynchronous collection. The back electromotive force in synchronous collection needs to be collected at a fixed time of PWM, either ON or OFF, depending on the zero-crossing comparison scheme of BEMF. The instantaneous current is generally collected at the TON moment. Because ST originally had a user channel for special PWM moments, I added the average current to this channel. In fact, the average current collection can also be put into asynchronous. It doesn't matter, the function is implemented without any problem.
In addition, the back-EMF channel in asynchronous acquisition is always set as the floating phase channel. Moreover, the back-EMF acquisition is between D and Z, that is, the asynchronous acquisition between the end of demagnetization and the zero crossing point is all back-EMF, while the instantaneous current acquisition is between Z and C, that is, the asynchronous acquisition between zero crossing and phase change is all instantaneous current. Therefore, the user's channel (average current) is between phase change and demagnetization.
Previous article:How to use IIC bus
Next article:Active brake in stm8-MC KIT library
Recommended ReadingLatest update time:2024-11-23 01:39
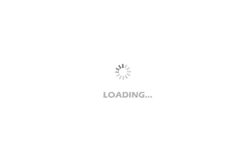
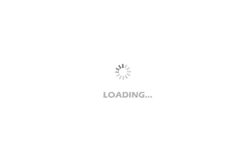
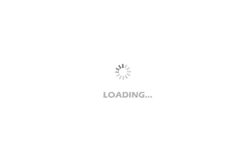
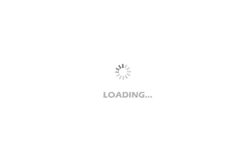
- Popular Resources
- Popular amplifiers
-
STM8 C language programming (1) - basic program and startup code analysis
-
Description of the BLDC back-EMF sampling method based on the STM8 official library
-
STM32 MCU project example: Smart watch design based on TouchGFX (8) Associating the underlying driver with the UI
-
uCOS-III Kernel Implementation and Application Development Practical Guide - Based on STM32 (Wildfire)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Internet of Things Electricity Metering System Based on RVB2610
- Introduction to the advantages of GaN, a key technology for realizing 5G
- 【NXP Rapid IoT Review】 NO4. Development Kit Engineering Construction and Actual Development
- Reference design for high-efficiency GaN inverter power stage
- DIY sun-tracking solar panels
- PGA_100 Days Journey_Simple State Machine
- Geng Shougong made a mobile phone charger in the shape of a thumbs-up, haha, it's quite interesting.
- Discussion on the Dilemma and Improvement Measures of RF Circuit PCB Design
- I am a PhD student
- TMS320C6678 Evaluation Module Core and Device Benchmarks