The TIM1 timer of ST's STM8 microcontroller is a 16-bit advanced control timer that can be used to achieve basic timing and PWM wave generation. Here we mainly analyze the basic timing function.
Let's first look at the initialization function of the TIM1 timer in the library function provided by ST
void TIM1_TimeBaseInit(uint16_t TIM1_Prescaler,
TIM1_CounterMode_TypeDef TIM1_CounterMode,
uint16_t TIM1_Period,
uint8_t TIM1_RepetitionCounter)
Among them, TIM1_Prescaler is a 16-bit frequency division configuration, and the input value can be 0-65535. This value is finally written into the TIM1_PSCR register, and the formula for calculating the timer frequency is: fcnk = fsys/(PSCR[15:0]+1), where fsys is the system clock frequency and PSCR[15:0] is the 16-bit value of the TIM1_PSCR register;
TIM1_CounterMode is the counting mode, there are 5 modes in total, namely:
TIM1_COUNTERMODE_UP Up counting mode
TIM1_COUNTERMODE_DOWN Down counting mode
TIM1_COUNTERMODE_CENTERALIGNED1
TIM1_COUNTERMODE_CENTERALIGNED2
TIM1_COUNTERMODE_CENTERALIGNED3
TIM1_Period, count value, this item can take any value from 1 to 65535
TIM1_RepetitionCounter, the number of repetition counts. This item can take any value from 0 to 255.
Assuming our system clock is 8Mhz and we set the timer to 1 second, then our initialization settings should be:
TIM1_TimeBaseInit(7,TIM1_COUNTERMODE_UP,1000,100);
Time calculation:
The frequency of the timer fcnk = 8Mhz/(7+1) = 1Mhz, which means that one count is 1us, 1000 counts are 1ms, and if the count is repeated 100 times, the interrupt timing time is 100ms. In order to achieve the effect of 1s, we also need to make a judgment 10 times in the interrupt function.
Interrupt function code implementation
INTERRUPT_HANDLER(TIM1_UPD_OVF_TRG_BRK_IRQHandler, 11)
{
count_number++;
if(count_number < 10)
{
}
else
{
count_number = 0; //1 second timer has been reached
}
TIM1_ClearITPendingBit(TIM1_IT_UPDATE);
}
The following is the relevant code to implement 1S timing
/*********************
*Function name: TIM_Config
*Function description: timer configuration
*Parameter Description:
* Input parameters None
* Output parameters None
*********************/
void TIM_Config(void)
{
TIM1_DeInit();
TIM1_TimeBaseInit(7,TIM1_COUNTERMODE_UP,1000,100);
TIM1_ARRPreloadConfig(ENABLE);
TIM1_ITConfig(TIM1_IT_UPDATE,ENABLE);
TIM1_Cmd(ENABLE);
}
/**
* @brief Timer1 Update/Overflow/Trigger/Break Interrupt routine.
* @param None
* @retval None
*/
INTERRUPT_HANDLER(TIM1_UPD_OVF_TRG_BRK_IRQHandler, 11)
{
/* In order to detect unexpected events during development,
it is recommended to set a breakpoint on the following instruction.
*/
count_number++;
if(count_number < 10)
{
}
else
{
count_number = 0;
}
TIM1_ClearITPendingBit(TIM1_IT_UPDATE);
}
Previous article:STM8S0 TIM1_PWM complementary output
Next article:stm8s003MCU_PWM_pin function configuration
Recommended ReadingLatest update time:2024-11-16 09:17
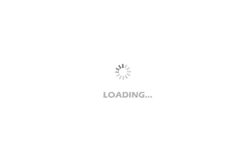
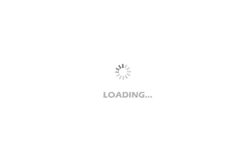
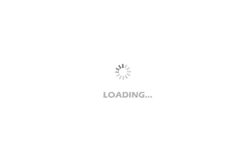
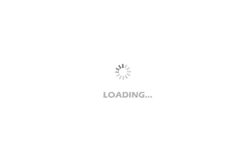
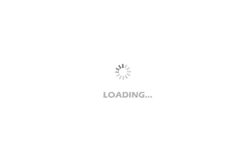
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications