The P1 switch controls the low four-bit running water light. The speed is a bit fast, so adjust it yourself. There are notes.
The microcontroller source program is as follows:
#include #define led P1 //Can be changed to other ports void delay(int x); void left(int x); void right(int x); void flash1(int x); void flash2(int x); void delay(int x) //delay function 1ms (approximately) {int i; while(x) { for(i=0;i<125;i++); x--; } } void left(int x) //From P1^3 to P1^0 (I call it left shift) {int i,j; while(x) {for(i=0;i<3;i++) // loop 3 times {led=0xfe; delay(50); //Each light is on for 50ms for(j=0;j<3;j++) //4 digits in total { led=led<<1|0xf1; delay(50); } } x--; } } void right(int x) //From the lowest bit to the highest bit (I call it right shift) {int i,j; while(x) //Others are the same as left shift {for(i=0;i<3;i++) { led=0xf7; delay(50); for(j=0;j<3;j++) { led=led>>1|0xf8; delay(50); } } x--; } } void flash1(int x) //Four-bit full flash (can be changed in many ways) {int i; while(x) {led=0xf0; delay(100); //lights up for 100ms each time for(i=0;i<1;i++) //You can change the number of loops { led=~led|0xf0; //shield the upper four bits delay(100); } x--; } } void flash2(int x) // flash every other bit (full flash change) {int i; while(x) //Basically the same as full flash {led=0xf5; delay(100); for(i=0;i<1;i++) { led=~led|0xf0; delay(100); } x--; } } main() {while(1) {led=0xff; switch(led) //When all lights are off, multiple buttons will not work when pressed at the same time; {case 0xef :{left(2);}break; //Press P1^4 to shift left and change the number of loops case 0xdf :{right(2);}break; //Press P1^5 to move right case 0xbf :{flash1(2);}break;//Press P1^6 to execute full flash case 0x7f :{flash2(2);}break;//Press P1^7 to execute full flash change default:{led=0xff;} } } } Copy code #include #define led P1 //Can be changed to other ports void delay(int x); void left(int x); void right(int x); void flash1(int x); void flash2(int x); void delay(int x) //delay function 1ms (approximately) {int i; while(x) { for(i=0;i<125;i++); x--; } } void left(int x) //From P1^3 to P1^0 (I call it left shift) {int i,j; while(x) {for(i=0;i<3;i++) // loop 3 times {led=0xfe; delay(50); //Each light is on for 50ms for(j=0;j<3;j++) //4 digits in total { led=led<<1|0xf1; delay(50); } } x--; } } void right(int x) //From the lowest bit to the highest bit (I call it right shift) {int i,j; while(x) //Others are the same as left shift {for(i=0;i<3;i++) { led=0xf7; delay(50); for(j=0;j<3;j++) { led=led>>1|0xf8; delay(50); } } x--; } } void flash1(int x) //Four-bit full flash (can be changed in many ways) {int i; while(x) {led=0xf0; delay(100); //lights up for 100ms each time for(i=0;i<1;i++) //You can change the number of loops { led=~led|0xf0; //shield the upper four bits delay(100); } x--; } } void flash2(int x) // flash every other bit (full flash change) {int i; while(x) //Basically the same as full flash {led=0xf5; delay(100); for(i=0;i<1;i++) { led=~led|0xf0; delay(100); } x--; } } main() {while(1) {led=0xff; //When all lights are off, multiple buttons will not work when pressed at the same time; if(led==0xef) //Press P1^4 to shift left, you can modify the number of loops; {left(2);} else if(led==0xdf) //Press P1^5 to move right {right(2);} else if(led==0xbf) //Press P1^6 to execute full flash {flash1(2);} else if(led==0x7f) //Press P1^7 to execute full flash change {flash2(2);} } }
Previous article:Single chip temperature control infrared remote control fan source program servo control direction of DC motor
Next article:STC89C51 MCU and LCD12864 display sin function image
Recommended ReadingLatest update time:2024-11-15 15:04
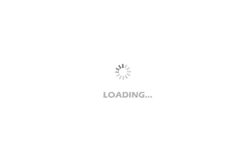
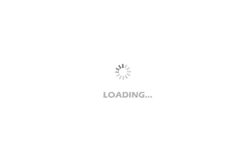
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
- Looking for AD component integrated library package library
- TMS320C6678 Evaluation Module
- Power Line Communication Simulation Reference Design for RS-485
- Buck circuit ripple test oscilloscope settings problem
- [RISC-V MCU CH32V103 Review] RTC Electronic Clock
- Implementing Inductive Proximity Sensing with a Single Chip
- 2596 ADJ proportional resistance selection
- Reference design of a true wireless headset charging box with ultra-low standby power consumption
- Is there any D/E power amplifier related information recommended?
- Continue to ask for help and supplement the "Sketch of Halogen Lamp Driver Power Supply Schematic" drawing.