Load/Store memory access instructions
— LDR word data load instruction
— LDRB Byte Data Load Instruction
— LDRH half-word data load instruction
— STR word data storage instruction
— STRB byte data storage instruction
— STRH half-word data storage instruction
Data processing instructions
MOV data transfer instruction, the valid number cannot exceed 2 hexadecimal digits (8 binary digits), MOV r2, #0xf800 is legal, MOV r2, 0x1510 is wrong, and the original data is lost after execution.
MVN data inversion transmission instruction; the effective number of inversion cannot exceed 2 hexadecimal digits (8 binary digits), MVN r2, #0xf800 is legal, MVN r2, 0x1510 is wrong, and the original data is lost after execution. CMP comparison instruction
— CMN Negative comparison instruction
— TST bit test instruction
— TEQ Test for equality instruction
— ADD addition instruction
— ADC Add with Carry instruction
— SUB subtraction instruction
— SBC Subtract with borrow instruction
— RSB Reverse subtraction instruction
— RSC Reverse subtraction with borrow instruction
— AND bitwise AND instruction
— ORR Bitwise OR instruction
— EOR bitwise exclusive OR instruction
— BIC bit clear instruction
Multiplication and multiply-add instructions
— MUL 32-bit multiplication instruction
— MLA 32-bit multiply-add instruction
— SMULL 64-bit signed multiplication instruction
— SMLAL 64-bit signed multiply-add instruction
— UMULL 64-bit unsigned multiplication instruction
— UMLAL 64-bit unsigned multiply-add instruction
Status register access instructions
— MRS Program Status Register to General Register Data Transfer Instructions
— MSR general register to program status register data transfer instruction
Shift Instructions
— LSL Logical Shift Left
— ASL Arithmetic Left Shift
— LSR Logical Shift Right
— ASR Arithmetic Shift Right
— ROR Rotate Right
— RRX Rotate right with extend
Jump Instructions
— B Jump instruction
— BL Jump instruction with return
— BLX Jump instruction with return and state switching
— BX Jump instruction with state switching
Coprocessor instructions
— LDC Coprocessor Data Load Instruction
— STC coprocessor data storage instruction
— MCR ARM processor register to coprocessor register data transfer instruction
— MRC coprocessor register to ARM processor register data transfer instruction
— CDP Coprocessor Number Operation Instructions
Other commonly used pseudo-instructions
— AREA
— ALIGN
— CODE16, CODE32
— ENTRY
— END
—EQU
— EXPORT (or GLOBAL)
— IMPORT
— EXTERN
— GET (or INCLUDE)
— INCBIN
— RN
— ROUT
1) ARM miscellaneous pseudo-instructions
1. ADR pseudo-instruction: a small range of address read pseudo-instruction.
The ADR instruction reads the address value based on the PC relative offset into the register. When assembling the source program, the ADR pseudo-instruction is replaced by a suitable instruction by the compiler. Usually the compiler uses an ADD instruction or SUB instruction to implement the function of the ADR pseudo-instruction.
Instruction format: ADR{cond} register ,expr
Register The register to load
Expr expression for program-relative or register-relative offset
Non-word-aligned addresses are in the range of -255 to 255 bytes;
The word-aligned address is in the range of -1020 to 1020 bytes.
Example:
Start MOV R1, #10
ADR R4, start; equivalent to PC-10 and then assigned to R4
2. ADRL instruction: medium range address read pseudo instruction.
The ADRL instruction reads the address value based on the PC relative offset or the address value based on the relative offset into the register, and can read a wider range of addresses than the ADR pseudo-instruction. When assembling the source program, the ADRL pseudo-instruction is replaced by two appropriate instructions by the compiler. If the ADRL pseudo-instruction function cannot be implemented with two instructions, an error will occur and the compilation will fail.
The instruction format is the same as ADR
Non-word-aligned addresses are within the 64K byte range;
Word-aligned addresses are within the 256K byte range.
Example:
Start MOV R1, #10
ADR R4, start+6000 ;=>ADD R4, PC, #0xe800 ADD R4, R4, #0x254
3. LDR instruction Large range address read pseudo instruction
The LDR pseudo-instruction is used to load a 32-bit immediate value or an address value into the specified register.
When assembling the source program, the LDR instruction is replaced by a suitable instruction by the compiler. If the loaded constant does not exceed the range of MOV or MVN, the MOV or MVN instruction is used instead of the LDR pseudo-instruction. Otherwise, the assembler puts the constant into the word pool (memory) and uses a program-relative offset LDR instruction to read the constant from the word pool.
Instruction format: LDR {cond} register , = expr/label_expr
Expr 32-bit immediate value
Label_expr PC-based address expression or external expression
Example
LDR R0, = 0x123987; load 32-bit immediate value
LDR R0, = DATA_BUF + 60; load DATA_BUF address + 60
4. NOP instruction
The NOP instruction generates the required ARM no-operation code. You can use the instruction MOV R0, R0. NOP cannot be used conditionally. Executing and not executing a no-operation instruction is the same, so conditional execution is not required. The ALU state is not affected by NOP.
2) Symbol Definition directive
The symbol definition directive is used to define variables in the ARM assembler, assign values to variables, and define register aliases.
Common symbol definition pseudo-instructions are as follows:
Ø GBLA, GBLL and GBLS for defining global variables
Ø LCLA, LCLL and LCLS for defining local variables
Ø SETA, SETL, SETS used to assign values to variables
Ø RLIST defines the name of the general register list
1. GBLA, GBLL and GBLS
Syntax format:
GBLA (GBLL or GBLS) global variable name
The GBLA, GBLL and GBLS directives are used to define and initialize global variables in an ARM program.
The GBLA directive is used to define a global numeric variable and initialize it to 0;
The GBLL pseudo-instruction is used to define a global logical variable and initialize it to F (false);
The GBLS directive is used to define a global string variable and initialize it to empty;
Since the above three directives are used to define global variables, the variable name must be unique within the entire program.
Example of use:
GBLA Test1; define a global numeric variable named Test1
Test1 SETA 0xaa; assign the variable to 0xaa
GBLL Test2; define a global logic variable named Test2
Test2 SETL {TRUE} ; Assign the variable a value of true
GBLS Test3; define a global string variable named Test3
Test3 SETS " Testing "; assign the variable to " Testing "
2. LCLA, LCLL and LCLS
Syntax format:
LCLA (LCLL or LCLS) local variable name
The LCLA, LCLL, and LCLS pseudo-instructions are used to define and initialize local variables in an ARM program.
The LCLA pseudo-instruction is used to define a local numeric variable and initialize it to 0;
The LCLL pseudo-instruction is used to define a local logic variable and initialize it to F (false);
The LCLS directive is used to define a local string variable and initialize it to empty;
The above three pseudo-instructions are used to declare local variables, and the variable name must be unique within its scope.
Example of use:
LCLA Test4; declare a local numeric variable named Test4
Test3 SETA 0xaa; assign the variable to 0xaa
LCLL Test5; declare a local logic variable named Test5
Test4 SETL {TRUE} ; Assign the variable a value of true
LCLS Test6; define a local string variable named Test6
Test6 SETS “ Testing ”; Assign the variable to “ Testing ”
3. SETA, SETL and SETS
Syntax format:
variable name SETA (SETL or SETS) expression
The pseudo-instructions SETA, SETL, and SETS are used to assign a value to an already defined global variable or local variable.
The SETA pseudo-instruction is used to assign a value to a mathematical variable;
The SETL directive is used to assign a value to a logical variable;
The SETS directive is used to assign a value to a string variable;
The variable name is a global variable or local variable that has been defined, and the expression is the value to be assigned to the variable.
Example of use:
LCLA Test3; declare a local numeric variable named Test3
Test3 SETA 0xaa; assign the variable to 0xaa
LCLL Test4; declare a local logic variable named Test4
Test4 SETL {TRUE} ; Assign the variable a value of true
4. RLIST
Syntax format:
NAME RLIST { register list }
The RLIST directive can be used to define a name for a general register list. The name defined by this directive can be used in the ARM instruction LDM/STM. In the LDM/STM instruction, the registers in the list are accessed in order from low to high according to the register number, regardless of the order of the registers in the list.
Example of use:
RegList RLIST {R0-R5, R8, R10}; defines the register list name as RegList, which can be used to access the register list in the ARM instruction LDM/STM.
3) Data Definition Directive
Data definition directives are generally used to allocate storage units for specific data and can also complete the initialization of the allocated storage units.
Common data definition pseudo instructions are as follows:
— DCB is used to allocate a continuous byte storage unit and initialize it with specified data.
— DCW (DCWU) is used to allocate a continuous half-word storage unit and initialize it with the specified data.
— DCD (DCDU) is used to allocate a continuous block of word memory locations and initialize them with specified data.
— DCFD (DCFDU) is used to allocate a continuous word storage unit for double-precision floating-point numbers and initialize it with the specified data.
— DCFS DCFSU) is used to allocate a continuous word storage unit for single-precision floating-point numbers and initialize it with the specified data.
— DCQ DCQU) is used to allocate a continuous storage unit of 8 bytes and initialize it with the specified data.
Previous article:UART communication of S3C2440
Next article:ARM LPC2132 Flowing Light LCD1602
Recommended ReadingLatest update time:2024-11-16 03:52
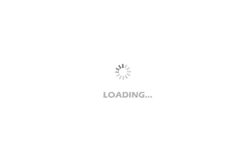
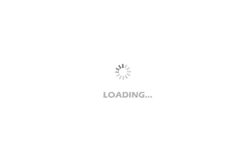
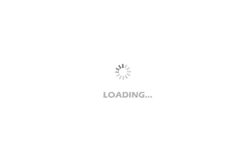
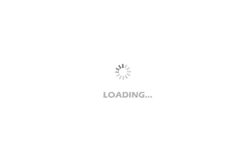
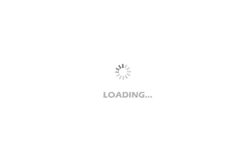
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Share the issues that young electronic engineers are most concerned about. What are you concerned about?
- BK9521 wireless chip transmitter circuit
- EEWORLD University Hall----TI motor driver chip latest technical advantages and applications
- 【ufun learning】Learning chapter 6: "Basic routine 5 - touch button control-PWM"
- 【Development and application based on NUCLEO-F746ZG motor】16. Function calculation of safety tasks - temperature calculation
- [Sipeed LicheeRV 86 Panel Review] 1- Unboxing Review
- EEWORLD University ---- Live Replay: How to complete efficient and reliable USB PD power design in minutes - PI Expert? Step-by-step tutorial
- TI Embedded Processors Live Review Highlights (2020)
- 【Repost】 Thirty Practical Experiences in Switching Power Supply Design (Part 2)
- Tin storage rosin splash