It takes a lot of time to watch videos on these basic things, and those who have some basic knowledge may not be very clear about the library when learning. I hope that the usage of the library I summarized can save everyone's time.
void TIM3_Init(u16 arr,u16 psc)
{
TIM3_Handler.Instance=TIM3; //General timer 3
TIM3_Handler.Init.Prescaler=psc; //Division factor
TIM3_Handler.Init.CounterMode=TIM_COUNTERMODE_UP; //Up counter
TIM3_Handler.Init.Period=arr; //Automatic load value
TIM3_Handler.Init.ClockDivision=TIM_CLOCKDIVISION_DIV1; //Clock division factor
HAL_TIM_Base_Init(&TIM3_Handler);
HAL_TIM_Base_Start_IT(&TIM3_Handler); //Enable timer 3 and timer 3 update interrupt: TIM_IT_UPDATE
}
The characteristic of the HAL library is that there are many structures nested so that one handle can configure all functions, which is very convenient.
In the HAL_TIM_Base_Init(&TIM3_Handler); function, the HAL_TIM_Base_MspInit(htim); function is called to configure the NVIC interrupt priority configuration.
We don't need to configure the interrupt configuration. We just need to write the function we want to execute in the callback function. It's super simple. As long as we understand the callback function, it is actually similar to the configuration judgment interruption execution. It just puts what we want to execute into a function call. Very concise
void HAL_TIM_Base_MspInit(TIM_HandleTypeDef *htim)
{
if(htim->Instance==TIM3)
{
__HAL_RCC_TIM3_CLK_ENABLE(); //Enable TIM3 clock
HAL_NVIC_SetPriority(TIM3_IRQn,1,3); //Set interrupt priority, preempt priority 1, subpriority 3
HAL_NVIC_EnableIRQ(TIM3_IRQn); //Enable ITM3 interrupt
}
}
//Timer 3 interrupt service function
void TIM3_IRQHandler(void)
{
HAL_TIM_IRQHandler(&TIM3_Handler);
}
//Callback function, timer interrupt service function call
void HAL_TIM_PeriodElapsedCallback(TIM_HandleTypeDef *htim)
{
if(htim==(&TIM3_Handler))
{
LED1=!LED1; //LED1 inverted
}
}
Now that we understand, let's look at two commonly used functions of general timers (I usually use PWM to control the motor and encoder to feedback the speed. I personally feel that these two functions are very practical, so I wrote a function to test it myself)
I spent three days adjusting the encoder. I had never written it before and used it directly. Now I find that I don’t understand too much. TIM_HandleTypeDef TIM4_Handler; //Timer 4 handle
TIM_Encoder_InitTypeDef TIM4_Encoder_Handler;
//Timer 4 channel 1 input capture configuration
//arr: automatic reload value (TIM2, TIM4 are 16 bits!!)
//psc: clock pre-division number
void TIM4_Encoder_Configration(u16 arr,u16 psc)
{
TIM4_Handler.Instance=TIM4;
TIM4_Handler.Init.Prescaler=0; //Division coefficient
TIM4_Handler.Init.CounterMode=TIM_COUNTERMODE_UP; //Up counter
TIM4_Handler.Init.Period=65535; //Automatically load value
TIM4_Handler.Init.ClockDivision=TIM_CLOCKDIVISION_DIV1; //Clock division silver
//HAL_TIM_Base_Init(&TIM4_Handler); //HAL_TIM_OC_Init(&TIM4_Handler);
TIM4_Encoder_Handler.EncoderMode=TIM_ENCODERMODE_TI12;
TIM4_Encoder_Handler.IC1Filter=0;
TIM4_Encoder_Handler.IC1Polarity=TIM_ICPOLARITY_RISING;
TIM4_Encoder_Handler.IC1Prescaler=TIM_ICPSC_DIV1;
TIM4_Encoder_Handler.IC1Selection=TIM_ICSELECTION_DIRECTTI;
TIM4_Encoder_Handler.IC2Filter=0;
TIM4_Encoder_Handler.IC2Polarity=TIM_ICPOLARITY_RISING;
TIM4_Encoder_Handler.IC2Selection=TIM_ICSELECTION_DIRECTTI;
TIM4_Encoder_Handler.IC2Prescaler=TIM_ICPSC_DIV1;
HAL_TIM_Encoder_Init(&TIM4_Handler,&TIM4_Encoder_Handler);
TIM_Encoder_Start(&TIM4_Handler,TIM_CHANNEL_ALL);
HAL_TIM_Encoder_Start_IT(&TIM4_Handler,TIM_CHANNEL_ALL);//Enable interrupt
TIM4->CNT=0;
__HAL_TIM_ENABLE_IT(&TIM4_Handler,TIM_IT_UPDATE); //Enable update interrupt must be enabled. This sentence has troubled me for two days.
//__HAL_TIM_ENABLE(&TIM4_Handler);
}
void HAL_TIM_Encoder_MspInit(TIM_HandleTypeDef *htim)
{
GPIO_InitTypeDef GPIO_Initure;
if(htim->Instance==TIM4)
{
__HAL_RCC_GPIOD_CLK_ENABLE(); //Turn on GPIOA clock__HAL_RCC_TIM4_CLK_ENABLE
(); //Enable TIM3
clockGPIO_Initure.Pin=GPIO_PIN_12|GPIO_PIN_13; //PA0
GPIO_Initure.Mode=GPIO_MODE_AF_PP; //InputGPIO_Initure.Pull=GPIO_PULLUP
; //Pull
upGPIO_Initure.Alternate=GPIO_AF2_TIM4; //PA0 is multiplexed as TIM4 channel 1
HAL_GPIO_Init(GPIOD,&GPIO_Initure);
//// HAL_NVIC_SetPriority(TIM4_IRQn,2,0); //Set interrupt priority, preempt priority 2, subpriority 0
//// HAL_NVIC_EnableIRQ(TIM4_IRQn); //Enable ITM4 interrupt channel
//
// HAL_NVIC_EnableIRQ(TIM4_IRQn); //Enable ITM4 interrupt channel
//ARPE enable
TIM4->CNT=0;
//TIM4->CNT = 0;
// __HAL_RCC_TIM4_CLK_ENABLE();//Configure timer four to start
}
}
To add, some files using the HAL library are
Clock configuration RCC: stm32f429xx_hal_rcc.h
GPIO configuration: stm32f429xx_hal_gpio.h Clock multiplexing configuration: stm32f429xx_gpio_ex.h
UART configuration: stm32f429xx_hal_time.h
Some configuration interrupts in the program are defined in the system interrupt in 32f429xx.h (this depends on the version of the MCU you use. I use the Zhengdian Atom F429IGT)
Previous article:Use of STM32L0xx_HAL_Driver library——UART
Next article:STM32l151 low power chip serial communication (HAL library)
Recommended ReadingLatest update time:2024-11-23 07:43
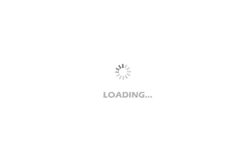
- Popular Resources
- Popular amplifiers
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- openocd command line programming ARM bare metal program problems and solutions
- Qualcomm warns the US government: If it does not sell chips to Huawei, it will give away $8 billion of market share to competitors every year
- The first embedded systems trade fair will be held
- Backpack solar panel modification
- Ultra-wideband technology UWB knowledge
- How is your circuit preparation going?
- How to configure the input and output of a DSP's GPIO?
- Some expansion module products of Raspberry Pi PICO
- How to use wireless firmware upgrades for MSP 430 microcontrollers
- The ultimate reason for high-frequency radiation exceeding the standard