Write a program to complete the following logical functions:
Q = XYZ\F + XY\ZF + X\YZF + XYZF (\Y means Y is not, \Z means Z is not, etc.)
This logical expression can be simplified to: Q = XYZ + XYF + XZF
Assume that the pins corresponding to each variable are as follows:
X: P1.0
Y: P1.1
Z: P1.2
F: P1.3
Q: P1.7
The procedure is as follows:
MOV C, P1.0
ANL C, P1.1
ANL C, P1.2
MOV F0, C
MOV C, P1.0
ANL C, P1.1
ANL C, P1.3
ORL C, F0
MOV F0, C
MOV C, P1.0
ANL C, P1.2
ANL C, P1.3
ORL C, F0
MOV P1.7, C
END
=============================
MCU: Please write a program to implement the XOR operation of bit X and bit Y.
2010-10-12 22:03 The Invincible Chinese | Category: Other Programming Languages | Views: 1546
Assume X and Y are stored at 00H and 01H respectively, and the result is that Z is stored at 02H.
(Note: 00H, 01H, 02H are bit addresses, belonging to D0, D1, D2 of internal RAM20H)
CLR 02H
MOV C, 00H
ANL C, 01H
JC _END_
MOV C, 00H
ORL C, 01H
JNC _END_
SETB 02H
_END_:
;over
END
=============================
Microcontroller: Please write a program to implement the XOR operation of bit X and bit Y.
Assume X and Y are stored in 00H and 01H respectively, and the result is that Z is stored in 02H.
Note: 00H, 01H, 02H are bit addresses, belonging to D0, D1, D2 of internal RAM20H
method 1:
;
MOV C, 00H
ANL C, /01H
MOV F0, C
MOV C, 01H
ANL C, /00H
ORL A, F0
MOV 02H, C
END
;-----------------------
Method 2:
;
CLR 02H
JNB 00H, ZZZ
JNB 01H, EXIT
SJMP EXIT
ZZZ:
JB 01H, EXIT
SETB 02H
EXIT:
END
;-----------------------
Method 3:
;
MOV A, 20H
R R
XRL A, 20H
RL A
RL A
ANL A, #00000100B
JZZZ
ORL 20H, A
SJMP EXIT
ZZZ:
CPL A
ANL 20H, A
EXIT:
END
;-----------------------
Previous article:Very amazing microcontroller program
Next article:Microcontroller assembly language programming: square root of the content in A
Recommended ReadingLatest update time:2024-11-16 03:48
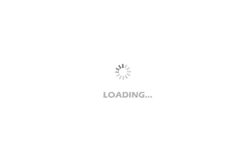
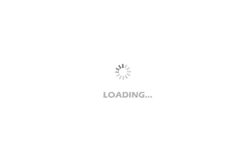
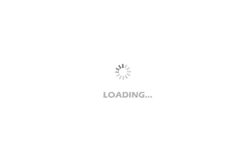
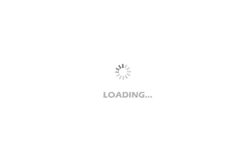
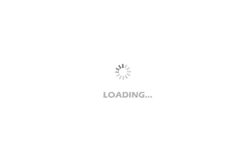
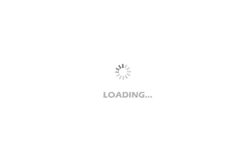
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Examples of several level conversion circuits
- Eliminating Software Failures with MSP432
- Single-button, double-button touch
- Does the IR21X series have a half-bridge driver chip with an output phase difference of 180°?
- BasicRF Simple Wireless Point-to-Point Transmission Protocol
- After this test, the source code can run normally. The trajectory planning of the robot arm can be realized in MATLAB
- Ultra-high precision level conversion circuit diagram
- ESP-Box: Espressif's open source 3D printing project based on ESP32-S3
- About the problem that the C51 microcontroller program cannot be downloaded in the HC6800 development board
- How to improve EMI on PCB by component placement?