I worked on it all night... but got nothing.
Maybe influenced by STM32, I always wanted to use its own library, but I was depressed for a long time.
No, without its own library
I went online to look up a routine for operating an LED.
I looked at several GPIO registers myself
There are mainly the following
1. Port x output data register (Px_ODR)
In output mode, the value written to the register is added to the corresponding pin through the latch. Reading the ODR register returns the previously latched register value.
In input mode, the value written to the ODR will be latched into the register, but will not change the pin state. The ODR register is always 0 after reset. Bit manipulation instructions (BSET, BRST) can be used to set the DR register to drive the corresponding pin, but will not affect other pins.
2. Port x input register (Px_IDR)
IDR[7:0]: Port input data register bit
Whether the pin is in input or output mode, the pin status value can be read through this register. This register is a read-only register.
0: logic low level
1: logic high level
3. Port x data direction (Px_DDR)
These bits can be set to 1 or 0 by software to select the pin as input or output
0: Input mode
1: Output mode
4. Port x Control Register 1 (Px_CR1)
In input mode (DDR=0):
0: floating input
1: input with pull-up resistor
In output mode (DDR=1):
0: simulated open-drain output (not true open-drain output)
1: push-pull output, output slew rate controlled by the corresponding bit of CR2
5. Port x control register 2 (Px_CR2) in input mode (DDR=0):
0: Disable external interrupt
1: Enable external interrupt
In output mode (DDR=1):
0: Maximum output speed is 2MHZ.
1: Maximum output speed is 10MHZ
The IDE I use is STVD. After creating a new project, put the STM8S208R.h library file in the newly created file
The following is programming
This is the code I wrote... It's pretty bad
///////////////////////////////////////////////////////////
/*============================================================================*/
/* PROJECT: LED Control by timer OC */
/* MODULE: main.c */
/* COMPILER: STM8 Cosmic C Compiler */
/* DATE: Feb 2009 */
/*----------------------------------------------------------------------------*/
/* DESCRIPTION: Demonstration firmware for STM8 Mini Kit */
/* Control LED blink, on/off/, brightness. */
/*============================================================================*/
/******************************************************************************
*
* THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS
* WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE
* TIME. AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY
* DIRECT, INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING
* FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE
* CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS.
*
* COPYRIGHT 2008 STMicroelectronics
******************************************************************************
*/
/* Includes ------------------------------------------------------------------*/
#include "stm8s208r.h" /* Registers and memory mapping file. */
/******************************************************************************/
/* Function definitions */
/******************************************************************************/
/* -------------------------------------------------------------------------- */
/* ROUTINE NAME: GPIO_Init */
/* INPUT/OUTPUT: None. */
/* DESCRIPTION: Initialize GPIOs. */
/* IN: None. */
/* OUT: None. */
/* -------------------------------------------------------------------------- */
void GPIO_Init(void)
{
/* LED IO Configuration */
/* LD3: PD3 */
/* LD2: PD1 */
/* LD1: PD0 */
PD_DDR |= 0xFF; /* Output. */
PD_CR1 |= 0xFF; /* PushPull. */
PD_CR2 = 0x00; /* Output speed up to 2MHz. */
PD_ODR |= 0x55;//IO口要输出的数据
}
/* -------------------------------------------------------------------------- */
/* ROUTINE NAME: CLK_Init */
/* INPUT/OUTPUT: None. */
/* DESCRIPTION: Initialize the clock source */
/* -------------------------------------------------------------------------- */
void CLK_Init(void)
{
/* Configure HSI prescaler*/
CLK_CKDIVR &= ~0x10; /* 01: fHSI= fHSI RC output/2. */
/* Configure CPU clock prescaler */
CLK_CKDIVR |= 0x01; /* 001: fCPU=fMASTER/2. */
}
/* -------------------------------------------------------------------------- */
/* ROUTINE: main */
/* main entry */
/* -------------------------------------------------------------------------- */
void main ( void )
{
while(1)
{
CLK_Init();
GPIO_Init();
}
}
/*---------------------------- End of file -----------------------------------*/
////////////////////////////////////////////////////////////////
This is just a very simple function
The road is long and arduous, and there is still a long way to go
Previous article:STM8 study notes - PWM module
Next article:STM8 study notes (II): GPIO input
Recommended ReadingLatest update time:2024-11-15 14:44
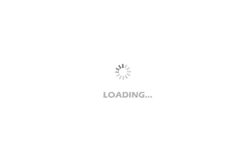
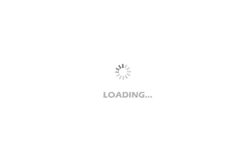
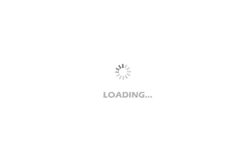
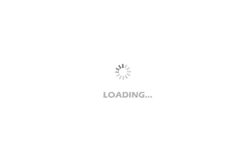
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
- [100 pieces 600 yuan] Free electronic kits, and [10,000 yuan in cash] are waiting for you to win! Digi-Key competition is waiting for you to join the team!
- CAN communication based on c5051f040
- SPI Communication of DSP28335
- [Creative Collection] MPS Exploration Camp "Serious Technology Play" | Unlock the infinite ways to play with lithium battery charging!
- [CH579M-R1] Problems encountered in ADC
- Register for the online seminar to win gifts | A complete analysis of the most powerful five-in-one smart door lock solution
- To solve the problem of impedance continuity in PCB design, just read this article!
- [Gizwits Gokit3 Review] Part 1: Some problems encountered during unboxing test
- Power-on issue with bq40z50-r1
- Ensure the quality and safety of child safety seats to protect children's travel