timing data implemented using timer A mode 2 (continuous) controls TACTL in the program. When TACTL is assigned the following value:
TACTL = TASSEL_2 + MC_2 + TAIE; // SMCLK, contmode, interrupt
TASSEL_X can be changed to achieve different timings.
When it is TASSEL_1, ACLK is selected as the timing clock, which can achieve the shortest timing of 2S, followed by timing of multiples of 2S. When
it is TASSEL_2, SMCLK is selected as the timing clock. The timing depends on the clock source of SMCLK.
1. SMCLK selects DCO = 800KHZ as the clock source. The shortest timing time is 0.08S, followed by its multiples.
2. SMCLK selects XT2, which is an external high-frequency crystal oscillator. At this time, the timing depends on the crystal oscillator frequency and its frequency division setting. The main thing is to set BCSCTL1 and BCSCTS2.
BCSCTL1 &= ~XT2OFF;
BCSCTL2 |= SELS + DIVS_3;
Different delays can be achieved by setting the above two sentences differently.
/************************************************************************
// MSP-FET430P140 Demo - Timer_A, Toggle P3.4, Overflow ISR, DCO SMCLK
//
// Description: Toggle P3.4 using software and Timer_A overflow ISR.
// In this example an ISR triggers when TA overflows. Inside the TA
// overflow ISR P3.4 is toggled. Toggle rate is approximatlely 12Hz. // Proper use of the TAIV interrupt vector generator
is demonstrated. // ACLK = n/a, MCLK = SMCLK = TACLK = default DCO ~
800kHz
//
// MSP430 F149
// --------------- //
/|| IAR Embedded Workbench Version: 3.42A Function: Timer A uses 800KHz DCO to achieve a timing interrupt of nearly one second; calculation method: T = 1/800,000 = 1.25uS so According to the continuous counting mode, when the count reaches 0XFFFF, an overflow interrupt occurs. Therefore, the overflow time is: 65536*1.25 = 0.0819S, so 12 overflows are required to count 1 second. ************************************************************************/ #include typedef unsigned char uchar; typedef unsigned int uint; uchar flag=0; void main(void) { WDTCTL = WDTPW + WDTHOLD; // Stop WDT BCSCTL1 &= ~XT2OFF; BCSCTL2 |= SELS + DIVS_3; 3D IR |= BIT4; TACTL = TASSEL_2 + MC_2 + TAIE; // SMCLK, contmode, interrupt _BIS_SR(LPM0_bits + GIE); // Enter LPM0 w/ interrupt } // Timer_A3 Interrupt Vector (TAIV) handler #pragma vector= TI MERA1_VECTOR __interrupt void Timer_A(void) { switch( TAIV ) { case 2: break; // CCR1 not used case 4: break; // CCR2 not used

case 10: P4OUT ^= BIT5; // overflow
break;
}
}
/*
// Timer A0 interrupt service routine
#pragma vector=TIMERA0_VECTOR
__interrupt void Timer_A (void)
{
P4OUT ^= BIT5; // Toggle P3.4
}
*/
(2) Timing data using timer A mode 1 (rising)
At this time, TACCTL0 and TACCR0 are used. Different timings can be achieved by setting different TACCR0 and different TASSEL_X.
However, the interrupt program needs to be changed.
Description of timer A interrupt:
1. The interrupt address of TIMERA1_VECTOR is 0XFFEA. This interrupt entry address contains 3 interrupt sources. It is a multi-source interrupt. The value of TAIV can be used to know which source the interrupt is from. When TAIV is 0X02H, the interrupt source is TACCR1 CCR1IFG.
When TAIV is 0X04H, the interrupt source is TACCR2 CCR2IFG
. When TAIV is 0X0AH, the interrupt source is TA OVERFLOW TAIFG.
2. The interrupt address of TIMERA0_VECTOR is 0XFFEC. It is a single-source interrupt. The interrupt source is TACCR0 CCR0IFG.
[page]
Description of timing time:
When TASSEL_1, select ACLK = 32.768KHZ. At this time, different delays can be achieved by selecting different TACCR0.
When TASSEL_2, select SMCLK = DCO When
SMCLK = XT2 is selected,
the key statements are:
TACTL = TASSEL_X + MC_1;
TACCR0 = ~~~~~~~;
TACCTL0 = CCIE;
(3) By using the comparison mode of timer A, output mode 4 and continuous counting mode, four independent timing intervals or four different frequency outputs can be realized at the same time.
In the process of the experiment, the four segments of the digital tube are driven at the same time.
In the comparison mode, the interrupt vectors and entry addresses of TACCR0 TACCR1 TACCR2 and TAIFG, as well as the output modes (8 in total) are mainly distinguished.
The program is as follows:
#include
typedef unsigned char uchar;
typedef unsigned int uint;
uchar flag=0;
uchar table[] = {0x18,0x7e,0x51,0x52,0x36,0x92,0x90,0x5E,0x10,0x12}; //Corresponding to the common anode code of 0---9
void main(void)
{
WDTCTL = WDTPW + WDTHOLD; // Stop WDT
//BCSCTL1 |= XT2OFF;
//BCSCTL2 |= SELS + DIVS_3;
TACCTL0 = OUTMOD_4 +CCIE ; //Enable interrupt
TACCTL1 = OUTMOD_4 +CCIE ;
TACCTL2 = OUTMOD_4 +CCIE ;
TACCR1 = 0X8000;
TACCR2 = 0X2000;
TACCR0 = 0XF000;
P3DIR |= BIT6 + BIT7; // P3.4 output
P3OUT |= BIT6 + BIT7;
P4DIR = 0XFF;
P4OUT = 0XFF;
TACTL = TASSEL_2 + MC_2 + TAIE; // SMCLK, contmode, interrupt
_BI S_SR(LPM0_bits + GIE); // Enter LPM0 w/ interrupt
}
/**/
// Timer_A3 Interrupt Vector (TAIV) handler
#pragma vector=TIMERA1_VECTOR
__interrupt void Timer_A(void)
{
switch( TAIV )
{
case 2: P4OUT ^= BIT5; TACCR1 += 0X8000;break; // CCR1 not used
case 4: P4OUT ^= BIT3;TAACCR2 += 0X2000;break; // CCR2 not used
case 10: flag++;
if(flag==12){P4OUT ^= BIT2; flag = 0; } // overflow
break;//flag++;
}
}
/**/
// Timer A0 interrupt service routine
#pragma vector=TIMERA0_VECTOR
__interrupt void Timer0_A (void )
{
P4OUT ^= BIT4; // Toggle P3.4
TACCR0 += 0XF000;
}
Previous article:MSP430 non-simulated IIC bus control program
Next article:My opinion on MSP430 introduction
Recommended ReadingLatest update time:2024-11-15 07:22
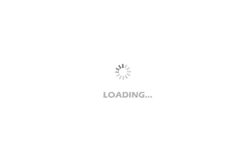
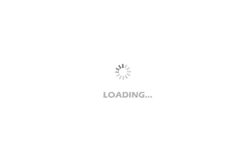
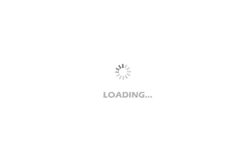
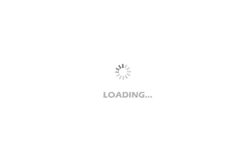
- Popular Resources
- Popular amplifiers
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- CGD and Qorvo to jointly revolutionize motor control solutions
- CGD and Qorvo to jointly revolutionize motor control solutions
- Keysight Technologies FieldFox handheld analyzer with VDI spread spectrum module to achieve millimeter wave analysis function
- Infineon's PASCO2V15 XENSIV PAS CO2 5V Sensor Now Available at Mouser for Accurate CO2 Level Measurement
- Advanced gameplay, Harting takes your PCB board connection to a new level!
- Advanced gameplay, Harting takes your PCB board connection to a new level!
- A new chapter in Great Wall Motors R&D: solid-state battery technology leads the future
- Naxin Micro provides full-scenario GaN driver IC solutions
- Interpreting Huawei’s new solid-state battery patent, will it challenge CATL in 2030?
- Are pure electric/plug-in hybrid vehicles going crazy? A Chinese company has launched the world's first -40℃ dischargeable hybrid battery that is not afraid of cold
- How to perform OTA upgrade on the OKT507-C development board on the Android system
- RT-Thread Application Practice-TI Temperature and Humidity HDC1000 Software Package Design and Production
- About Flash memory sectors, blocks, and pages
- Components Science Popularization: Component Selection Specifications
- Domestic PCB drawing software
- Solutions to the "Disconnected-you are now offline" issue when connecting to the Ubuntu system
- Are there step by step instructions for adding a custom service?
- ESP8266 Getting Started Guide
- Millimeter wave sensors bring new intelligence to industrial applications
- Switching Power Supply Interest Group Task 05