Other modes: audio decoding mode, frame mode, see the data sheet for details
Interrupts
The SPI module is capable of generating interrupts to reflect events that occur during data communications. It can generate the following types of interrupts: The receive data available interrupt is indicated by SPI1RXIF and SPI2RXIF. This event occurs when
new data is assembled in the SPIxBUF receive buffer .
The transmit buffer is empty, interrupt is indicated by SPI1TXIF and SPI2TXIF. This event occurs when there is
space and new data can be written.
Error interrupts are indicated by SPI1EIF and SPI2EIF. This event occurs when the SPIxBUF receive buffer has an overflow condition (ie,
new receive data has been assembled but the previous data has not yet been read), when the transmit buffer is insufficient, or when a FRMERR
event .
bit 3-2 STXISEL<1:0>: SPI Transmit Buffer Empty Interrupt Mode bits(1,3)
11 = SPIxTXIF is set when the buffer is not full (has one or more empty elements) 10
= SPIxTXIF is set when the buffer is half empty or more 01
= SPIxTXIF is set when the buffer is completely empty 00
= SPIxTXIF is set when the last transmit data is shifted out of the SPISR and the transmit operation is complete
bit 1-0 RTXISEL<1:0>: SPI Receive Buffer Full Interrupt Mode bits(1,3)
11 = SPIxRXIF is set when the buffer is full 10
= SPIxRXIF is set when the buffer is half empty or more 01
= SPIxRXIF is set when the buffer is not empty 00 = SPIxRXIF is set when the last word
in the receive buffer is read (i.e., the buffer is empty)
Light up the digital tube
#include
#pragma config FPLLIDIV = DIV_2 // PLL Input Divider (2x Divider)
#pragma config FPLLMUL = MUL_24 // PLL Multiplier (24x Multiplier)
#pragma config FPLLODIV = DIV_2 // System PLL Output Clock Divider (PLL Divide by 2)
#pragma config FNOSC = FRCPLL // Oscillator Selection Bits (Fast RC Osc with PLL)
#pragma config JTAGEN = OFF // JTAG Enable (JTAG Disabled)
#pragma config FWDTEN = OFF // Watchdog Timer Enable (WDT Disabled (SWDTEN Bit Controls))
char Led[]={0x42, 0xf3, 0x86, 0xa2, 0x33, 0x2a, 0x0a, 0xf2, 0x02, 0x22, 0x40, 0xf1, 0x84, 0xa0, 0x31, 0x28, 0x08, 0xf0, 0x00, 0x20, 0x1e, 0x0e, 0x0f, 0xbf, 0x23, 0x9b, 0x8b};
//led字库
void spiout(char image[],int len)
{
int i;
PORTClearBits(IOPORT_B, BIT_9);
for (i = 0; i < len; i++)
{
SpiChnPutC(2, image[ i]);
}
for(i=0;i<60;i++); //Wait for data to be stored successfully
PORTSetBits(IOPORT_B, BIT_9); //Latch data to be displayed
}
int main()
{
PPSOutput(2, RPB8, SDO2);
//Output pin group 2, look up the table to use pin RPB8 as data output port 2 SDO2. The actual connection is the same
SpiOpenFlags oFlags = SPI_OPEN_MSTEN | SPI_OPEN_CKP_HIGH | SPI_OPEN_MODE8 | SPI_OPEN_ON; //As a host, the clock pulse is high when idle, 8-bit data mode, SPI is enabled
SpiChnOpen(2, oFlags, 6); //Open channel 2, namely SDO2, configure SPI, divide frequency fpbDiv (2~1024). Baud rate BR=Fpb/fpbDiv
PORTSetPinsDigitalOut(IOPORT_B, BIT_9); //External shift register data latch, 1 latch, 0 open
// SPI2CONCLR=(1<<15); SPI2CONCLR=0X8000; Multiple operations
spiout(Led,4);
}
The chip 74HC595 used in the digital tube description
has an 8-bit shift register and a memory inside. The shift register and the storage register have independent clock signals. The data is input at the rising edge of the shift register clock signal SHCP and enters the storage register at the rising edge of the storage register clock signal STCP. The shift register has a serial shift input DS, a serial output Q7' and an asynchronous low-level reset MR. Since the output high level of the input/output port of PIC32MX is a voltage of 3.3V, it cannot directly drive the chip 74HC595 powered by 5V. A level conversion matching circuit composed of a diode and a pull-up resistor is used. The circuit is simple, reliable and low-cost.
When the SDO pin receives a low-level signal output by the SPI of the PIC32MX, the diode is turned on and OUT1 is a low-level signal. When the SDO pin receives a high-level signal, the OUT1 end of the diode is connected to 5V through a pull-up resistor to output a high-level signal higher than 4V, thereby realizing the matching function of level conversion.
When multiple seven-segment digital tubes are required for display, the following processing can be performed: each 74HC595 shares the SHCP and STCP clock signals, and Q7' of the previous stage 74HC595 is connected to the DS of the next stage 74HC595 in turn.
When all the data are shifted into the shift registers of all 74HC595s, and all the shift registers of 74HC595s have been updated, the SLCK signal is used to shift all the data into the storage register for latching, thereby realizing the latching and display of the LED display signal.
Appendix 1
typedef enum
{
// master opening mode 主
SPI_OPEN_MSTEN = _SPIxCON_MASK_(MSTEN_MASK), // set the Master mode
SPI_OPEN_SMP_END = _SPIxCON_MASK_(SMP_MASK), // Master Sample Phase for the input bit at the end of the data out time. Otherwise data is sampled in the middle.
SPI_OPEN_MSSEN = _SPIxCON_MASK_(MSSEN_MASK), // enable the driving of the Slave Select (SS) output pin by the Master
SPI_OPEN_MSSEN_HIGH = _SPIxCON_MASK_(FRMPOL_MASK), // Master driven SS output active high. Otherwise low.
// slave opening mode 从
SPI_OPEN_SLVEN = 0, // set the Slave mode
SPI_OPEN_SSEN = _SPIxCON_MASK_(SSEN_MASK), // enable the SS input pin.
SPI_OPEN_MCLKSEL = _SPIxCON_MASK_(MCLKSEL_MASK),
// clocking opening mode 时钟
SPI_OPEN_CKP_HIGH = _SPIxCON_MASK_(CKP_MASK), // set the clock polarity to (idle-high, active-low). Otherwise is (idle-low, active-high).
SPI_OPEN_CKE_REV = _SPIxCON_MASK_(CKE_MASK), // set the Clock Edge reversed: transmit from active to idle. Otherwise transmit when clock goes from idle to active
// data characters opening mode 数据
SPI_OPEN_MODE8 = 0, // set 8 bits/char
SPI_OPEN_MODE16 = _SPIxCON_MASK_(MODE16_MASK), // set 16 bits/char
SPI_OPEN_MODE32 = _SPIxCON_MASK_(MODE32_MASK), // set 32 bits/char
// framed mode opening mode 帧高级
SPI_OPEN_FRMEN = _SPIxCON_MASK_(FRMEN_MASK), // Enable the Framed SPI support. Otherwise the Framed SPI is disabled.
SPI_OPEN_FSP_IN = _SPIxCON_MASK_(FRMSYNC_MASK), // Frame Sync Pulse (FSP) direction set to input (Frame Slave).
// Otherwise the FSP is output and the SPI channel operates as a Frame Master.
SPI_OPEN_FSP_HIGH = _SPIxCON_MASK_(FRMPOL_MASK), // FSP polarity set active high. Otherwise the FSP is active low.
SPI_OPEN_FSP_CLK1 = _SPIxCON_MASK_(SPIFE_MASK), // Set the FSP to coincide with the 1st bit clock.
// Otherwise the FSP precedes the 1st bit clock
SPI_OPEN_FSP_WIDE = _SPIxCON_MASK_(FRMSYPW_MASK), // set the FSP one character wide. Otherwise the FSP is one clock wide.
SPI_OPEN_FRM_CNT1 = (0 << _SPIxCON_MASK_(FRMCNT_POSITION)), // set the number of characters per frame (Frame Counter) to 1 (default)
SPI_OPEN_FRM_CNT2 = (1 << _SPIxCON_MASK_(FRMCNT_POSITION)), // set the Frame Counter to 2
SPI_OPEN_FRM_CNT4 = (2 << _SPIxCON_MASK_(FRMCNT_POSITION)), // set the Frame Counter to 4
SPI_OPEN_FRM_CNT8 = (3 << _SPIxCON_MASK_(FRMCNT_POSITION)), // set the Frame Counter to 8
SPI_OPEN_FRM_CNT16 = (4 << _SPIxCON_MASK_(FRMCNT_POSITION)), // set the Frame Counter to 16
SPI_OPEN_FRM_CNT32 = (5 << _SPIxCON_MASK_(FRMCNT_POSITION)), // set the Frame Counter to 32
// enhanced buffer (FIFO) opening mode enhanced buffer and interrupt mode
SPI_OPEN_ENHBUF = _SPIxCON_MASK_(ENHBUF_MASK), // enable the enhanced buffer mode
SPI_OPEN_TBE_NOT_FULL = (3 << _SPIxCON_MASK_(STXISEL_POSITION)), // Tx Buffer event issued when Tx buffer not full (at least one slot empty)
SPI_OPEN_TBE_HALF_EMPTY = (2 << _SPIxCON_MASK_(STXISEL_POSITION)), // Tx Buffer event issued when Tx buffer >= 1/2 empty
SPI_OPEN_TBE_EMPTY = (1 << _SPIxCON_MASK_(STXISEL_POSITION)), // Tx Buffer event issued when Tx buffer completely empty
SPI_OPEN_TBE_SR_EMPTY = (0 << _SPIxCON_MASK_(STXISEL_POSITION)), // Tx Buffer event issued when the last character is shifted out of the internal Shift Register
// and the transmit is complete
SPI_OPEN_RBF_FULL = (3 << _SPIxCON_MASK_(SRXISEL_POSITION)), // Rx Buffer event issued when RX buffer is full
SPI_OPEN_RBF_HALF_FULL = (2 << _SPIxCON_MASK_(SRXISEL_POSITION)), // Rx Buffer event issued when RX buffer is >= 1/2 full
SPI_OPEN_RBF_NOT_EMPTY = (1 << _SPIxCON_MASK_(SRXISEL_POSITION)), // Rx Buffer event issued when RX buffer is not empty
SPI_OPEN_RBF_EMPTY = (0 << _SPIxCON_MASK_(SRXISEL_POSITION)), // Rx Buffer event issued when RX buffer is empty (the last character in the buffer is read).
// general opening mode 常用
SPI_OPEN_DISSDO = _SPIxCON_MASK_(DISSDO_MASK), // disable the usage of the SDO pin by the SPI
SPI_OPEN_DISSDI = _SPIxCON_MASK_(DISSDI_MASK), // disable the usage of the SDI pin by the SPI
SPI_OPEN_SIDL = _SPIxCON_MASK_(SIDL_MASK ), // enable the Halt in the CPU Idle mode. Otherwise the SPI will be still active when the CPU is in Idle mode.
SPI_OPEN_ON = _SPIxCON_MASK_(ON_MASK ), // turn ON the SPI (not used in SpiChnOpen)
}SpiOpenFlags; // open flags that can be used with SpiChnOpen. Defined in the processor header file.
Previous article:PIC16F914 outputs adjustable duty cycle PWM waveform
Next article:PIC32 Output Compare (PWM)
Recommended ReadingLatest update time:2024-11-22 20:56
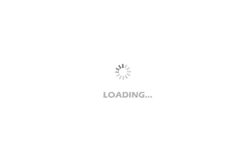
- Popular Resources
- Popular amplifiers
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Using a Digital Multimeter to Detect Intermittent Faults
- Can I use Android system programming to realize USB port output to simulate keyboard and mouse to control PC functions?
- Tips on how to modify the original battery to enhance the battery of digital camera
- TLP3547 Review (Part 1)
- Related applications of ultrasonic water level sensors
- Research on Several Issues of Portable Image Acquisition System Based on ARM and FPGA
- 51 single chip microcomputer DC brushless motor drive solution
- China's fiber optic recovery
- What skills do you need to master to switch to integrated circuits? Let's discuss
- What types of antennas are there? Detailed explanation of antenna types