1. Specific code of led.c:
/*----------------------------------------------------------*/
#include "led.h"
/* -------------------------------------------------------------------------
File name: led.c
Description: Configure the LED port according to the hardware connection and open the corresponding register
---------------------------------------------------------------------------*/
void LED_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
//Open the PB port clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB,ENABLE);
//Open the PE port clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOE,ENABLE);
//PB5, PE5 pin settings
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_5;
//Port speed
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
//Port mode, this is output push-pull mode
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(GPIOB,&GPIO_InitStructure);
GPIO_Init(GPIOE,&GPIO_InitStructure);
}
Initialization: First, turn on the clock, set the pins, set the port speed, and set the port mode.
2. The header file of led.h
#ifndef __LED_H
#define __LED_H
#include "stm32f10x.h"
#define LED2_OFF GPIO_SetBits(GPIOE,GPIO_Pin_5)
#define LED2_ON GPIO_ResetBits(GPIOE,GPIO_Pin_5)
#define LED2_REV GPIO_WriteBit(GPIOE,GPIO_Pin_5,(BitAction)(1-(GPIO_ReadOutputDataBit(GPIOE,GPIO_Pin_5))))
#define LED3_OFF GPIO_SetBits(GPIOB,GPIO_Pin_5)
#define LED3_ON GPIO_ResetBits(GPIOB,GPIO_Pin_5)
#define LED3_REV GPIO_WriteBit(GPIOB,GPIO_Pin_5,(BitAction)(1-(GPIO_ReadOutputDataBit(GPIOB,GPIO_Pin_5))))
void LED_Init(void);
#endif
LED switch, flip
3. main.c function code
/*----------------------------------------------------------------------------------
File name: Control LED2 and LED3 to flash
Hardware platform: STM32F103 development board
Author: Qiushi
Firmware library: V3.5
-----------------------------------------------------------------------------------*/
/* Includes ------------------------------------------------------------------*/
#include "stm32f10x.h"
#include "led.h"
int main(void)
{
uint32_t i;
LED_Heat();
LED2_ON;
LED3_OFF;
for(i=0; i < 0xffffff; i++)
while (1)
{
for(i = 0; i < 0xfffff;i++);
LED2_REV;
LED3_REV;
}
}
When we use functions when writing code, we give priority to using function macros.
Previous article:stm32 serial port lighting
Next article:STM32 Getting Started: Lighting
Recommended ReadingLatest update time:2024-11-23 02:49
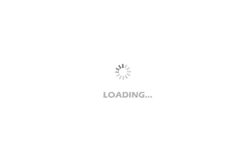
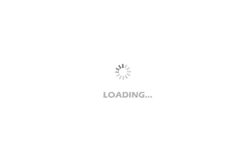
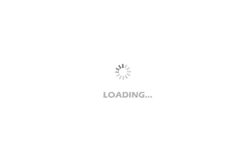
- Popular Resources
- Popular amplifiers
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- What is a servo motor? Summary of servo motor knowledge
- [SC8905 EVM Review] Keeping up with the progress - Preliminary testing of the evaluation board and comparison with similar products
- MatLab Commands.pdf
- [GD32E503 Review] Part 4: Screen and RTC code integration
- Application skills/Design and implementation of single chip caller display
- Bipolar current source maintains high output impedance at high frequencies
- Are there really any breakthroughs in battery technology today?
- Apple's 140W GaN charger is as powerful as ever
- Design of Fully Digital Quadrature Phase Shift Keying Demodulator
- "Play Board" + Shared Bicycle Control Board - GPS Module ublox MAX-M8Q