1. Problems and Phenomena
There is no problem in using USART_SendData() function to send single characters non-continuously; when sending characters continuously (no delay between two characters), the sending buffer will overflow. If the amount of data sent is very small, the serial port will only send the last character. When the amount of data sent is large, the sent data will be lost inexplicably.
like:
1
2
|
for(TxCounter = 0;TxCounter < RxCounter; TxCounter++)
USART_SendData(USART1, RxBuffer[TxCounter]);
|
2. Reasons
This API function is imperfect. There is no statement in the function body to determine whether a character has been sent. Instead, the data is directly placed in the send buffer. When sending data continuously, due to the speed limit of the send shift register (related to the communication baud rate), the data in the send buffer overflows. The old data has not been sent out in time, and the new data overwrites the old data in the send buffer.
3. Solution
I won't talk about the method of waiting for a period of time after sending. The waiting time is uncertain, which is the worst option. The following two solutions are provided:
Solution 1. Check the status bit after each character is sent
USART_SendData(USART1, RxBuffer[TxCounter]);
while(USART_GetFlagStatus(USARTx, USART_FLAG_TXE) == RESET){} //Wait for the send buffer to be empty before sending the next character
Solution 2. Modify the library function
Modify the USART_SendData() function and add the USART_FLAG_TXE status detection statement of the send buffer to ensure that one character is completely sent before sending the next character.
Implementation method: Check the status register every time a character is sent to ensure that the data has been sent. The specific steps are as follows.
Function definition body before modification
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
void USART_SendData(USART_TypeDef* USARTx, u16 Data)
{
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_DATA(Data));
USARTx->DR = (Data & (u16)0x01FF);
}
|
Modified function definition body
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
void USART_SendData(USART_TypeDef* USARTx, u16 Data)
{
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_DATA(Data));
USARTx->DR = (Data & (u16)0x01FF);
while(USART_GetFlagStatus(USARTx, USART_FLAG_TXE) == RESET){} //Wait for the send buffer to be empty before sending the next character
}
|
Some people may think, why not handle this problem in advance in the library function, and throw the solution to the user. Personally, I think the reason why ST does this is: use the send interrupt function.
STM32 library function USART_SendData problem and solution | Xiao Xie's blog http://blog.xieyc.com/stm32-lib-function-usart-send-data-problem-and-solution/
Previous article:STM32 hardware multiplier - another difference from MSP430 hardware multiplier
Next article:8 working modes of GPIO in STM32!
Recommended ReadingLatest update time:2024-11-15 10:49
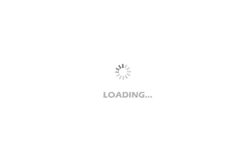
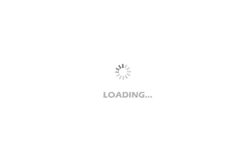
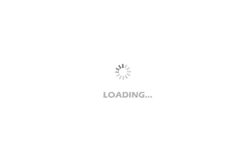
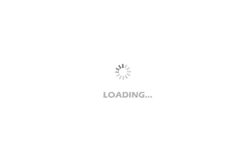
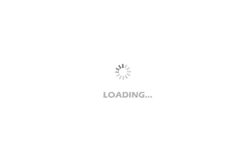
- Popular Resources
- Popular amplifiers
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- ASML predicts that its revenue in 2030 will exceed 457 billion yuan! Gross profit margin 56-60%
- Detailed explanation of intelligent car body perception system
- How to solve the problem that the servo drive is not enabled
- Why does the servo drive not power on?
- What point should I connect to when the servo is turned on?
- How to turn on the internal enable of Panasonic servo drive?
- What is the rigidity setting of Panasonic servo drive?
- How to change the inertia ratio of Panasonic servo drive
- What is the inertia ratio of the servo motor?
- Is it better for the motor to have a large or small moment of inertia?
- Analysis of embedded real-time operating system uCOS II
- Some Misunderstandings of FPGA Learning
- Automatic phase and frequency shifting circuit diagram in karaoke, collection required
- Today's broadcast starts at 10:00: The development and latest applications of sensors in industrial motors
- 【ST NUCLEO-G071RB review】——by lising
- Playing with Zynq Serial 4 - Introduction to AXI Bus Protocol 2
- 150V Non-Synchronous Buck Solution with Voltage Controller
- When will the gifts redeemed from TI Mall be shipped?
- WPI Group sincerely invites you to gather in Chongqing to attend the "Ansemester and NXP - Automotive Electronics Innovation Products and Technology Seminar"
- RVB2601 Evaluation Board Trial 5: Remote Audio Acquisition System