Operation on pins:
E.g. light up the LED light:
1. First, you need to know the pins corresponding to the LED lights, find its module block diagram and clock tree in the datasheet of the corresponding chip, find the parent clock and turn it on. Then initialize it with the structure, and configure the corresponding parameters according to your needs during initialization.
The clock tree of the ZET6 datasheet is as follows:
Module diagram:
2. Initialize it using the initialization function.
3. Find the schematic diagram and find out whether the component to be operated requires a high level or a low level. For example, the LED light here requires a high level, and the level can be raised through the void GPIO_SetBits(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin) function.
If the component requires a low level, you can pull the level low through the void GPIO_ResetBits(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin) function.
The level can also be set directly through bit operations.
Use the void GPIO_WriteBit(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin, BitAction BitVal) function to directly write all the level conditions of a module.
The operation is as follows:
For example, you want to set GPIO_Pin_10 to a high level.
You can use GPIO_SetBits(GPIOA,GPIO_Pin_10);
You can also use GPIO_WriteBit(0x01<<10);
Above code:
void Led_Init()
{
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10|GPIO_Pin_11|GPIO_Pin_12|GPIO_Pin_13;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOE, ENABLE);
GPIO_Init(GPIOE, &GPIO_InitStructure);
}
Previous article:STM32 IO configuration lighting
Next article:STM32 Review Notes (15) Infrared Remote Control
Recommended ReadingLatest update time:2024-11-22 21:12
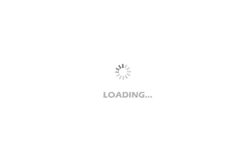
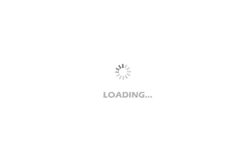
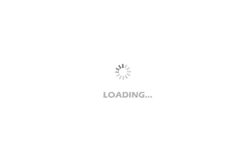
- Popular Resources
- Popular amplifiers
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Why does the STM series MCU use SMD T card instead of traditional T card?
- LM258 circuit help
- Some Experience on Loop Compensation
- Why are chips so difficult to make? It's a miracle to make these
- What are the advantages of transistor + MOS over MOS alone? After all, the battery is a single cell, and the full charge is 4.2V, which is low voltage.
- Issues with identifiers in AD09
- The problem that the main function cannot be run after downloading the program with IAR compiler
- msp430fr5992 runtime problem
- Stick to your job
- [Hua Diao Experience] 07 Building a Xingkong board development environment for VSCode programming