In daily work, if you use MSP430 as the main control chip, you often need to write SPI or I2C drivers to read and control peripherals (such as LCD screens and some sensors). In order to reduce repetitive work, this article summarizes the detailed steps of SPI driver writing with a specific example (using MSP430FR6989 to drive the integrated analog front end AFE4400):
MCU SPI pin settings
SPI read and write timing settings
Register Write
Final Thoughts
MCU SPI pin settings
Generally, SPI has 3-wire and 4-wire, the difference lies in whether it has a chip select terminal - STE pin. The function description of the 4 pins is:
UCxS0MI: data input in master mode, data output in slave mode;
UCxSIMO: data output in master mode, data input in slave mode;
UCxCLK: USCI SPI clock;
UCxSTE: USCI SPI enable terminal;
Pin setup code:
void Set_UCB0_SPI(void)
{
P1SEL0 |= BIT4 | BIT6 | BIT7; // Activate the corresponding pin as SPI function, here use USCI_B0 (SPI P1.4 UCB0CLK P1.7 UCB0SOMI)
P1DIR &= ~BIT6; // P1.6 UCB0SOMI The MCU here is the host, set to input direction
P1DIR |= BIT5 | BIT4 | BIT7; // P1.5 (SPI STE) P1.4 (UCB0CLK) P1.7 (UCB0SOMI) are all output pins
P1OUT |= BIT5; // Enable the terminal to be high, and SPI communication is not performed at this time
PM5CTL0 &= ~LOCKLPM5; // Activate the setting of the above pins of the MCU. Attention!!! Special commands of MSP430FR series MCU. I have not found this before.
// commands, all pin configurations don't work, I was screwed by 00 for a long time!!!
UCB0CTLW0 |= UCSWRST; // Enable SW reset
UCB0CTLW0 |= UCMSB+UCCKPH+UCMST+UCSYNC;
// 1 - Synchronous mode
// [b2-1] 00- 3-pin SPI
// [b3] 1 - Master mode
// [b4] 0 - 8-bit data
// [b5] 1 - MSB first
// [b6] 0 - Clock polarity high.
//[b7] 1 - Clock phase - Data is captured on the first UCLK edge and changed on the following edge.
//For the above settings, refer to the manual of the module to be driven, pay attention to the [b3] [b4] bits!
UCB0CTLW0 |= UCSSEL_2; // SMCLK
UCB0BR0 = 0x01; // 16 MHz
UCB0BR1 = 0; //
UCB0CTLW0 &= ~UCSWRST; // Clear SW reset, resume operation
//UCB0IE = 0x00;
}
SPI read and write timing settings
According to the data sheet, understanding the module's SPI read and write timing is the key step to successful programming! ! !
The following is the SPI read and write timing diagram of AFE4400:
When reading data: pull STE low, first send a byte of register address to AFE4400, wait for a while, AFE4400 will return the data of the address to the microcontroller, send byte by byte, a total of 3 bytes of 24-bit data. (The microcontroller needs to send three times at a time)
When writing data: pull STE low, first send the register address you want to write, and then send 3 bytes of 24-bit data in sequence, you can change the data of the corresponding register in AFE4400. (The microcontroller needs to send three times at a time)
SPI reads the code of AFE4400 register value:
unsigned long AFE4400_Reg_Read(unsigned char Reg_address)
{
unsigned char SPI_Rx_buf[4]; //Store the read register value
unsigned long retVal;
retVal = 0;
P1OUT&= ~BIT5; // Pull STE low
UCB0TXBUF = Reg_address; // Send the register address to be read
while ( (UCB0STAT & UCBUSY) ); // USCI_B0 TX buffer ready?
SPI_Rx_buf[0] = UCB0RXBUF; // Read the received data, which is empty data at this time
UCB0TXBUF = 0; // Empty instruction, waiting for delay effect
while ( (UCB0STAT & UCBUSY) ); // USCI_B0 TX buffer ready?
SPI_Rx_buf[1] = UCB0RXBUF; // Read received data: Data[23:16]
UCB0TXBUF = 0; // Empty instruction, waiting for delay effect
while ( (UCB0STAT & UCBUSY) ); // USCI_B0 TX buffer ready?
SPI_Rx_buf[2] = UCB0RXBUF; // Read received data: Data[15:8]
UCB0TXBUF = 0; // Empty instruction, waiting for delay effect
while ( (UCB0STAT & UCBUSY) ); // USCI_B0 TX buffer ready?
SPI_Rx_buf[3] = UCB0RXBUF; // Read received data: Data[7:0]
P1OUT|=BIT5; // Reading completed, pull STE high
retVal = SPI_Rx_buf[1]; //Integrate data into 24-bit data
retVal = (retVal << 8) | SPI_Rx_buf[2];
retVal = (retVal << 8) | SPI_Rx_buf[3];
return retVal;
}
SPI writes data into the AFE4400 register code:
void AFE4400_Reg_Write (unsigned char reg_address, unsigned long data)
{
unsigned char dummy_rx;
P1OUT&= ~BIT5; // Pull STE low
UCB0TXBUF = reg_address; // Send the register address to be written
while ( (UCB0STAT & UCBUSY) ); // USCI_B0 TX buffer ready?
dummy_rx = UCB0RXBUF; // Empty instruction, waiting for delay effect
UCB0TXBUF = (unsigned char)(data >>16); // Pass the data to be written: Data[23:16] to the transmit buffer
while ( (UCB0STAT & UCBUSY) ); // USCI_B0 TX buffer ready?
dummy_rx = UCB0RXBUF; // Empty instruction, waiting for delay effect
UCB0TXBUF = (unsigned char)(((data & 0x00FFFF) >>8)); // Pass the data to be written: Data[15:8] to the transmit buffer
while ( (UCB0STAT & UCBUSY) ); // USCI_B0 TX buffer ready?
dummy_rx = UCB0RXBUF; // Empty instruction, waiting for delay effect
UCB0TXBUF = (unsigned char)(((data & 0x0000FF))); // Pass the data to be written: Data[7:0] to the transmit buffer
while ( (UCB0STAT & UCBUSY) ); // USCI_B1 TX buffer ready?
dummy_rx = UCB0RXBUF; // Empty instruction, waiting for delay effect
P1OUT|=BIT5; // Writing completed, pull STE high
}
Register Write
After completing the above two steps, AFE4400 can be used by us obediently and listen to us very much! By checking the register function manual and writing the corresponding values to configure the functions of AFE4400, we can achieve the functions we want.
Some registers of AFE4400:
Previous article:【TI MSP430】How to implement analog serial communication
Next article:MSP430 SPI communication routine (SD card initialization-theoretical explanation)
Recommended ReadingLatest update time:2024-11-23 11:29
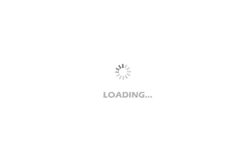
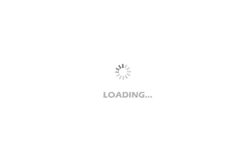
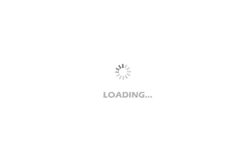
- Popular Resources
- Popular amplifiers
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Raspberry Pi Development in Action (2nd Edition) ([UK] Simon Monk)
-
Automotive Electronics S32K Series Microcontrollers: Based on ARM Cortex-M4F Core
-
Learn C language for AVR microcontrollers easily (with video tutorial) (Yan Yu, Li Jia, Qin Wenhai)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Resistor power selection problem
- Want to be a power engineer? Find out these first
- [GD32L233C-START Review] 2. Non-blocking way to light up, blink, blink, blink...
- Two new millimeter-wave radars debut with a detection distance of 200 meters
- [F-Wireless Microphone Amplification System] Hubei Province_First Prize
- Debugging there (汇编及C语言) program,sja1000+tja1040
- The main technical problems and solutions of MMS at present
- [Repost] Nine things to note when testing PCB boards
- [Fudan Micro FM33LC046N Review] + GPTIM_PWM
- Automotive RFCMOS 77GHz Radar Module Design