1. Basic Concepts
1. FSMC with non-bus multiplexed 16-bit SRAM interface
configures
SRAM memory and NOR flash memory to share the same FSMC storage block. The protocol used varies depending on the memory type.
To control the SRAM memory, the FSMC should have the following functions:
●
Enable or disable the multiplexing function of the address/data bus.
●
Select the memory type used: NOR flash, SRAM or PSRAM.
●
Define the data bus width of the external memory: 8 or 16 bits.
●
Enable or disable extended mode: Extended mode is used to access memories with different read and write operation timings.
Just like configuring NOR flash memory, the user must calculate and set the following parameters according to the timing data given in the data sheet of the SRAM memory:
●
ADDSET: address setup time
●
ADDHOLD: address hold time
●
DATAST: data setup time
2. Routines
1. FSMC_SRAM.C
/******************** (C) COPYRIGHT 2008 STMicroelectronics ********************
* File Name : fsmc_sram.c
* Author : MCD Application Team
* Version : V2.0.1
* Date : 06/13/2008
* Description : This file provides a set of functions needed to drive the
* IS61WV51216BLL SRAM memory mounted on STM3210E-EVAL board.
********************************************************************************
* THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS
* WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE TIME.
* AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY DIRECT,
* INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING FROM THE
* CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE CODING
* INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS.
*******************************************************************************/
/* Includes ------------------------------------------------------------------*/
#include "fsmc_sram.h"
/* Private typedef -----------------------------------------------------------*/
/* Private define ------------------------------------------------------------*/
#define Bank1_SRAM3_ADDR ((u32)0x68000000)
#define SRAM_WRITE(Address, Data) (*(vu16 *)(Address) = (Data))
/* Private macro -------------------------------------------------------------*/
/* Private variables ---------------------------------------------------------*/
/* Private function prototypes -----------------------------------------------*/
/* Private functions ---------------------------------------------------------*/
/*******************************************************************************
* Function Name : FSMC_SRAM_Init
* Description : Configures the FSMC and GPIOs to interface with the SRAM memory.
* This function must be called before any write/read operation
* on the SRAM.
* Input : None
* Output : None
* Return : None
*******************************************************************************/
void FSMC_SRAM_Init(void)
{
FSMC_NORSRAMInitTypeDef FSMC_NORSRAMInitStructure;
FSMC_NORSRAMTimingInitTypeDef p;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOD | RCC_APB2Periph_GPIOG | RCC_APB2Periph_GPIOE |
RCC_APB2Periph_GPIOF, ENABLE);
/*-- GPIO Configuration ------------------------------------------------------*/
/* SRAM Data lines configuration */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_8 | GPIO_Pin_9 |
GPIO_Pin_10 | GPIO_Pin_14 | GPIO_Pin_15;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOD, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_7 | GPIO_Pin_8 | GPIO_Pin_9 | GPIO_Pin_10 |
GPIO_Pin_11 | GPIO_Pin_12 | GPIO_Pin_13 | GPIO_Pin_14 |
GPIO_Pin_15;
GPIO_Init(GPIOE, &GPIO_InitStructure);
/* SRAM Address lines configuration */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3 |
GPIO_Pin_4 | GPIO_Pin_5 | GPIO_Pin_12 | GPIO_Pin_13 |
GPIO_Pin_14 | GPIO_Pin_15;
GPIO_Init(GPIOF, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3 |
GPIO_Pin_4 | GPIO_Pin_5;
GPIO_Init(GPIOG, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_11 | GPIO_Pin_12 | GPIO_Pin_13;
GPIO_Init(GPIOD, &GPIO_InitStructure);
/* NOE and NWE configuration */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_4 |GPIO_Pin_5;
GPIO_Init(GPIOD, &GPIO_InitStructure);
/* NE3 configuration */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_Init(GPIOG, &GPIO_InitStructure);
/* NBL0, NBL1 configuration */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1;
GPIO_Init(GPIOE, &GPIO_InitStructure);
/*-- FSMC Configuration ------------------------------------------------------*/
p.FSMC_AddressSetupTime = 0;
p.FSMC_AddressHoldTime = 0;
p.FSMC_DataSetupTime = 2;
p.FSMC_BusTurnAroundDuration = 0;
p.FSMC_CLKDivision = 0;
p.FSMC_DataLatency = 0;
p.FSMC_AccessMode = FSMC_AccessMode_A;
FSMC_NORSRAMInitStructure.FSMC_Bank = FSMC_Bank1_NORSRAM3;
FSMC_NORSRAMInitStructure.FSMC_DataAddressMux = FSMC_DataAddressMux_Disable;
FSMC_NORSRAMInitStructure.FSMC_MemoryType = FSMC_MemoryType_SRAM;
FSMC_NORSRAMInitStructure.FSMC_MemoryDataWidth = FSMC_MemoryDataWidth_16b;
FSMC_NORSRAMInitStructure.FSMC_BurstAccessMode = FSMC_BurstAccessMode_Disable;
FSMC_NORSRAMInitStructure.FSMC_WaitSignalPolarity = FSMC_WaitSignalPolarity_Low;
FSMC_NORSRAMInitStructure.FSMC_WrapMode = FSMC_WrapMode_Disable;
FSMC_NORSRAMInitStructure.FSMC_WaitSignalActive = FSMC_WaitSignalActive_BeforeWaitState;
FSMC_NORSRAMInitStructure.FSMC_WriteOperation = FSMC_WriteOperation_Enable;
FSMC_NORSRAMInitStructure.FSMC_WaitSignal = FSMC_WaitSignal_Disable;
FSMC_NORSRAMInitStructure.FSMC_ExtendedMode = FSMC_ExtendedMode_Disable;
FSMC_NORSRAMInitStructure.FSMC_AsyncWait = FSMC_AsyncWait_Disable;
FSMC_NORSRAMInitStructure.FSMC_WriteBurst = FSMC_WriteBurst_Disable;
FSMC_NORSRAMInitStructure.FSMC_ReadWriteTimingStruct = &p;
FSMC_NORSRAMInitStructure.FSMC_WriteTimingStruct = &p;
FSMC_NORSRAMInit(&FSMC_NORSRAMInitStructure);
/* Enable FSMC Bank1_SRAM Bank */
FSMC_NORSRAMCmd(FSMC_Bank1_NORSRAM3, ENABLE);
}
/*******************************************************************************
* Function Name : FSMC_SRAM_WriteBuffer
* Description : Writes a Half-word buffer to the FSMC SRAM memory.
* Input : - pBuffer : pointer to buffer.
* - WriteAddr : SRAM memory internal address from which the data
* will be written.
* - NumHalfwordToWrite : number of half-words to write.
*
* Output : None
* Return : None
*******************************************************************************/
void FSMC_SRAM_WriteBuffer(u16* pBuffer, u32 WriteAddr, u32 NumHalfwordToWrite)
{
for(; NumHalfwordToWrite != 0; NumHalfwordToWrite--) /* while there is data to write */
{
/* Transfer data to the memory */
*(u16 *) (Bank1_SRAM3_ADDR + WriteAddr) = *pBuffer++;
/* Increment the address*/
WriteAddr += 2;
}
}
/*******************************************************************************
* Function Name : FSMC_SRAM_ReadBuffer
* Description : Reads a block of data from the FSMC SRAM memory.
* Input : - pBuffer : pointer to the buffer that receives the data read
* from the SRAM memory.
* - ReadAddr : SRAM memory internal address to read from.
* - NumHalfwordToRead : number of half-words to read.
* Output : None
* Return : None
*******************************************************************************/
void FSMC_SRAM_ReadBuffer(u16* pBuffer, u32 ReadAddr, u32 NumHalfwordToRead)
{
for(; NumHalfwordToRead != 0; NumHalfwordToRead--) /* while there is data to read */
{
/* Read a half-word from the memory */
*pBuffer++ = *(vu16*) (Bank1_SRAM3_ADDR + ReadAddr);
/* Increment the address*/
ReadAddr += 2;
}
}
/******************* (C) COPYRIGHT 2008 STMicroelectronics *****END OF FILE****/
/***************************************************************
* Function Name : FSMC_SRAM_WriteHalfWord
* Description : Writes a half-word to the SRAM memory.
* Input : - WriteAddr : SRAM memory internal address to write to.
* - Data : Data to write.
* Output : None
* Return :
*******************************************************************************/
void FSMC_SRAM_WriteHalfWord(u32 WriteAddr, u16 Data)
{
//NOR_WRITE(ADDR_SHIFT(0x05555), 0x00AA);
// NOR_WRITE(ADDR_SHIFT(0x02AAA), 0x0055);
// NOR_WRITE(ADDR_SHIFT(0x05555), 0x00A0);
SRAM_WRITE((Bank1_SRAM3_ADDR + WriteAddr), Data);
//return (FSMC_NOR_GetStatus(Program_Timeout));
}
/******************************************************************************
* Function Name : FSMC_SRAM_ReadHalfWord
* Description : Reads a half-word from the SRAM memory.
* Input : - ReadAddr : NOR memory internal address to read from.
* Output : None
* Return : Half-word read from the SRAM memory
*******************************************************************************/
u16 FSMC_SRAM_ReadHalfWord(u32 ReadAddr)
{
//NOR_WRITE(ADDR_SHIFT(0x005555), 0x00AA);
//NOR_WRITE(ADDR_SHIFT(0x002AAA), 0x0055);
//NOR_WRITE((Bank1_NOR2_ADDR + ReadAddr), 0x00F0 );
return (*(vu16 *)((Bank1_SRAM3_ADDR + ReadAddr)));
}
2.MAIN.C
/******************** (C) COPYRIGHT 2008 STMicroelectronics ********************
* File Name : main.c
* Author : MCD Application Team
* Version : V2.0.1
* Date : 06/13/2008
* Description : Main program body
********************************************************************************
* THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS
* WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE TIME.
* AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY DIRECT,
* INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING FROM THE
* CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE CODING
* INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS.
*******************************************************************************/
/* Includes ------------------------------------------------------------------*/
#include "fsmc_sram.h"
/* Private typedef -----------------------------------------------------------*/
/* Private define ------------------------------------------------------------*/
#define BUFFER_SIZE 0x400
#define WRITE_READ_ADDR 0x8000
/* Private macro -------------------------------------------------------------*/
/* Private variables ---------------------------------------------------------*/
GPIO_InitTypeDef GPIO_InitStructure;
ErrorStatus HSEStartUpStatus;
u16 TxBuffer[BUFFER_SIZE];
u16 RxBuffer[BUFFER_SIZE];
u32 WriteReadStatus = 0, Index = 0;
/* Private function prototypes -----------------------------------------------*/
void RCC_Configuration(void);
void NVIC_Configuration(void);
void Fill_Buffer(u16 *pBuffer, u16 BufferLenght, u32 Offset);
/* Private functions ---------------------------------------------------------*/
/*******************************************************************************
* Function Name : main
* Description : Main program.
* Input : None
* Output : None
* Return : None
*******************************************************************************/
int main(void)
{
#ifdef DEBUG
debug();
#endif
/* System Clocks Configuration */
RCC_Configuration();
/* NVIC Configuration */
NVIC_Configuration();
/* PF.06 and PF.07 config to drive LD1 and LD2 ******************************/
/* Enable GPIOF clock */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOF, ENABLE);
/* Configure PF.06 and PF.07 as Output push-pull */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOF, &GPIO_InitStructure);
/* Write/read to/from FSMC SRAM memory *************************************/
/* Enable the FSMC Clock */
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_FSMC, ENABLE);
/* Configure FSMC Bank1 NOR/SRAM3 */
FSMC_SRAM_Init();
/* Write data to FSMC SRAM memory */
/* Fill the buffer to send */
Fill_Buffer(TxBuffer, BUFFER_SIZE, 0x3212);
FSMC_SRAM_WriteBuffer(TxBuffer, WRITE_READ_ADDR, BUFFER_SIZE);
/* Read data from FSMC SRAM memory */
FSMC_SRAM_ReadBuffer(RxBuffer, WRITE_READ_ADDR, BUFFER_SIZE);
/* Read back SRAM memory and check content correctness */
for (Index = 0x00; (Index < BUFFER_SIZE) && (WriteReadStatus == 0); Index++)
{
if (RxBuffer[Index] != TxBuffer[Index])
{
WriteReadStatus = Index + 1;
}
}
if (WriteReadStatus == 0)
{ /* OK */
/* Turn on LD1 */
GPIO_SetBits(GPIOF, GPIO_Pin_6);
}
else
{ /* KO */
/* Turn off LD2 */
GPIO_SetBits(GPIOF, GPIO_Pin_7);
}
while (1)
{
}
}
/*******************************************************************************
* Function Name : RCC_Configuration
* Description : Configures the different system clocks.
* Input : None
* Output : None
* Return : None
*******************************************************************************/
void RCC_Configuration(void)
{
/* RCC system reset(for debug purpose) */
RCC_DeInit();
/* Enable HSE */
RCC_HSEConfig(RCC_HSE_ON);
/* Wait till HSE is ready */
HSEStartUpStatus = RCC_WaitForHSEStartUp();
if(HSEStartUpStatus == SUCCESS)
{
/* Enable Prefetch Buffer */
FLASH_PrefetchBufferCmd(FLASH_PrefetchBuffer_Enable);
/* Flash 2 wait state */
FLASH_SetLatency(FLASH_Latency_2);
/* HCLK = SYSCLK */
RCC_HCLKConfig(RCC_SYSCLK_Div1);
/* PCLK2 = HCLK */
RCC_PCLK2Config(RCC_HCLK_Div1);
/* PCLK1 = HCLK/2 */
RCC_PCLK1Config(RCC_HCLK_Div2);
/* PLLCLK = 8MHz * 9 = 72 MHz */
RCC_PLLConfig(RCC_PLLSource_HSE_Div1, RCC_PLLMul_9);
/* Enable PLL */
RCC_PLLCmd(ENABLE);
/* Wait till PLL is ready */
while(RCC_GetFlagStatus(RCC_FLAG_PLLRDY) == RESET)
{
}
/* Select PLL as system clock source */
RCC_SYSCLKConfig(RCC_SYSCLKSource_PLLCLK);
/* Wait till PLL is used as system clock source */
while(RCC_GetSYSCLKSource() != 0x08)
{
}
}
}
/*******************************************************************************
* Function Name : NVIC_Configuration
* Description : Configures Vector Table base location.
* Input : None
* Output : None
* Return : None
*******************************************************************************/
void NVIC_Configuration(void)
{
#ifdef VECT_TAB_RAM
/* Set the Vector Table base location at 0x20000000 */
NVIC_SetVectorTable(NVIC_VectTab_RAM, 0x0);
#else /* VECT_TAB_FLASH */
/* Set the Vector Table base location at 0x08000000 */
NVIC_SetVectorTable(NVIC_VectTab_FLASH, 0x0);
#endif
}
/*******************************************************************************
* Function name : Fill_Buffer
* Description : Fill the global buffer
* Input : - pBuffer: pointer on the Buffer to fill
* - BufferSize: size of the buffer to fill
* - Offset: first value to fill on the Buffer
* Output param : None
*******************************************************************************/
void Fill_Buffer(u16 *pBuffer, u16 BufferLenght, u32 Offset)
{
u16 IndexTmp = 0;
/* Put in global buffer same values */
for (IndexTmp = 0; IndexTmp < BufferLenght; IndexTmp++ )
{
pBuffer[IndexTmp] = IndexTmp + Offset;
}
}
#ifdef DEBUG
/*******************************************************************************
* Function Name : assert_failed
* Description : Reports the name of the source file and the source line number
* where the assert_param error has occurred.
* Input : - file: pointer to the source file name
* - line: assert_param error line source number
* Output : None
* Return : None
*******************************************************************************/
void assert_failed(u8* file, u32 line)
{
/* User can add his own implementation to report the file name and line number,
ex: printf("Wrong parameters value: file %s on line %d/r/n", file, line) */
/* Infinite loop */
while (1)
{
}
}
#endif
Previous article:STM32 uses FSMC to control NAND flash routine
Next article:STM32 uses fsmc to control NOR flash routine
Recommended ReadingLatest update time:2024-11-15 15:08
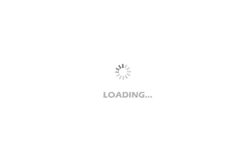
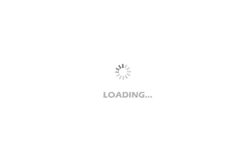
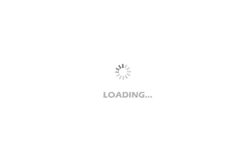
- Popular Resources
- Popular amplifiers
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
- [Raspberry Pi Pico Review] - PWM and breathing light examples
- A Maximum Power Point Tracking (MPPT) solar charge controller for 12V and 24V solar panels
- 【Silicon Labs Development Kit Review】+DEMO Program Download
- IoT indoor environment monitor based on ESP32-S2-Kaluga-1
- EEWORLD University Hall----Kalman Filter
- SensorTile.box related information
- Review summary: Anxinke ESP32-Audio-Kit free review and trial
- Detailed analysis of the damage causes of power MOS tubes
- Which is the control chip of this flyback power supply?
- Chanel lost the lawsuit against Huawei for logo infringement. Do you think these two logos are similar?