When do we need to use enum? When the value of a variable is between several ranges, such as a week variable that can only take values of Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, and Sunday. It is better to use enum in this case. Of course, define can also be used. However, define is more troublesome to maintain and is suitable for when the value range of the variable is small.
1. Using the enum keyword to describe constants (i.e., describing enumeration constants) has the following advantages:
(1) It makes the program easier to maintain and more intuitive. For example, the enumeration defined below describes the number of transmission bits supported by the UART. From the definition of the enumeration type, we can know the several modes of transmission bits provided by the UART module.
enum { usartDatabits4 = USART_FRAME_DATABITS_FOUR, /* 4 databits (not available for UART). */ usartDatabits5 = USART_FRAME_DATABITS_FIVE, /**< 5 databits (not available for UART). */ usartDatabits6 = USART_FRAME_DATABITS_SIX, /**< 6 databits (not available for UART). */ usartDatabits7 = USART_FRAME_DATABITS_SEVEN, /**< 7 databits (not available for UART). */ usartDatabits8 = USART_FRAME_DATABITS_EIGHT, /**< 8 databits. */ usartDatabits9 = USART_FRAME_DATABITS_NINE, /**< 9 databits. */ usartDatabits10 = USART_FRAME_DATABITS_TEN, /**< 10 databits (not available for UART). */ } USART_Databits_Enum;
(2) Make the program safer
. For example, when writing the underlying driver, a UART initialization function UartInit(uint32 baudrate, uint32 bit) is provided to the upper layer. When calling this function, the programmer may provide the wrong bit parameter because he does not understand the transmission bit mode supported by the module UART. To avoid this phenomenon, we can of course check the bit range in the UartInit() function. If the parameter values are continuous, it is fine. If they are not continuous, multiple if-else or switch will be used. It will consume a certain amount of ROM and CPU time.
We can define the function as UartInit(uint32 baudrate, USART_Databits_Enum bit). On the one hand, we can easily know the transmission bit mode supported by UART by checking the USART_Databits_Enum enumeration type. On the other hand, we can avoid passing wrong parameters during compilation.
2. The difference and connection between enumeration and macro definition:
The difference between macro and enumeration lies in the period of action and the form of storage. Macro is replaced in the preprocessing stage, it replaces the text of the code segment, and the macro no longer exists during the program running. Enumeration takes effect after the program is running, and the enumeration constant is stored in the static storage area of the data segment. (In the IAR compilation environment, the enumeration type defaults to character type. In programming under the M3 core, in order to reduce unnecessary character extension instructions, you can use the ?enum_is_int instruction to force all enumeration types to be 4 bytes.)
But we cannot say that macros are better than enumerations. If you need to define a lot of constants, using one enum {…} is obviously clearer than a bunch of defines. Enumerations can also be used to define some special types, such as Bool, such as: type enum {FALSE,TRUE} Bool;
Previous article:My understanding of microcontrollers
Next article:C language pointers and variables in single chip microcomputer
Recommended ReadingLatest update time:2024-11-15 12:06
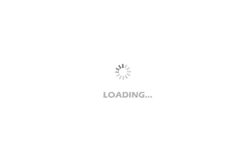
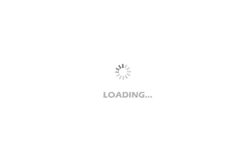
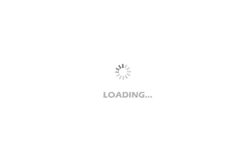
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Microchip Accelerates Real-Time Edge AI Deployment with NVIDIA Holoscan Platform
- Microchip Accelerates Real-Time Edge AI Deployment with NVIDIA Holoscan Platform
- Melexis launches ultra-low power automotive contactless micro-power switch chip
- Melexis launches ultra-low power automotive contactless micro-power switch chip
- Molex leverages SAP solutions to drive smart supply chain collaboration
- Pickering Launches New Future-Proof PXIe Single-Slot Controller for High-Performance Test and Measurement Applications
- Apple faces class action lawsuit from 40 million UK iCloud users, faces $27.6 billion in claims
- Apple faces class action lawsuit from 40 million UK iCloud users, faces $27.6 billion in claims
- The US asked TSMC to restrict the export of high-end chips, and the Ministry of Commerce responded
- The US asked TSMC to restrict the export of high-end chips, and the Ministry of Commerce responded
- Doorbell program + circuit made by AVR microcontroller timer
- [N32L43X Review] 2.IO Operation and Delay
- AR1021X series WiFi module selection reference and driver discussion in the field of wireless image transmission
- Allegro software placement problem
- 2812 TxPR register write failure caused by TxCON highest bit
- Fabrication process of fiber-embedded microfluidic chip
- TMS320F28335 general purpose input/output port GPIO related register introduction
- On-line debugging method for embedded processors
- "Qinheng Evaluation Board Sincerely Send" Activity Q&A Post
- The problem with "LTE Cat 1"