introduction
This application note describes how to manage the internal, partition-erasable data and program flash memory in MAXQ microcontrollers. It generally describes how to build a bootloader application that allows in-application programming of program flash memory. Note: This article does not apply to MAXQ microcontrollers that use page-erasable flash memory, that is, microcontrollers that allow only small amounts of flash memory to be erased. Each MAXQ data sheet will describe the type of flash memory used.
Flash Memory Introduction
Memory ConfigurationsThis application note shows various flash memory configurations for different sizes, which are not necessarily specific to a particular MAXQ device. These configurations are used for examples in this document only. The data sheet for each MAXQ device lists the memory configuration for that device.
There is no operational distinction between the boot, program and data areas. If the boot loader requires more space than the first flash area can be extended to the next partition. However, in the following examples the numbering is different.
Table 1. Flash configuration examples
Data Flash
Data Flash can be used to reliably store some system data that needs to be saved once or periodically during system operation. The capacity of data flash varies depending on the specific MAXQ device, usually between 128 and 2k words.
There are some limitations to the use of data flash. Unlike EEPROM, data flash cannot be erased word by word; a complete partition must be erased each time. Erasing a partition typically takes 0.7 seconds, but can take up to 15 seconds in the worst case. During this time, user code stops running and no other operations can be performed. Therefore, these limitations must be carefully considered when selecting software technology based on system requirements. For most periodic data storage, bounded queues and/or partition switching techniques can meet system reliability requirements. A simple example of partition switching and bounded queue techniques is given below.
Bounded Queue
A bounded queue is a queue that contains a fixed number of elements. This method is often used to process periodic data. For example, a 2k word data flash can be divided into 32 to 64 word entries, as shown in the memory configuration in Table 2.
At initialization, the startup routine scans the queue to determine the next available entry in the queue. Once the queue is full, the next entry can be written only after it is erased. If all entries are to be retained, the partition must be changed to preserve all data. After the flash is erased, new entries can be written. The disadvantage of this approach is that if power is lost during the erase or write process, all data will be lost. Figure 1 shows the flow of loading entries into a bounded queue. Appendix A gives a simple C source code example.
If this bounded queue approach does not meet your system requirements, you can also use partition exchange technology.
Table 2. Bounded queue memory configuration example
FLASHQueue[ ] | |
Queue Index | Data Flash Address |
31 | 0xF7C0-0xF7FF |
30 | 0xF780-0xF7BF |
29 | 0xF740-0xF77F |
. . . . | . . . . |
2 | 0xF080-0xF0BF |
1 | 0xF040-0xF07F |
0 | 0xF000-0xF03F |
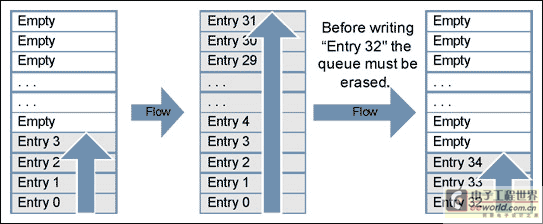
Figure 1. Bounded queue process
Block swapping
Block swapping can effectively prevent data loss or corruption during the lengthy partition erase process. The "block" here is equivalent to the "partition". Block swapping is best suited for situations where the partition size is slightly larger than the total amount of data. The disadvantage is that at least two data flash partitions are required. When the total amount of data to be written is much smaller than the partition size, it is best to combine block swapping with bounded queues.
If block swapping is required, a MAXQ device with at least two data flash partitions should be selected. Table 3 shows an example memory configuration with two 1K x 16 flash partitions. Figure 2 shows the block swap write/erase flow.
Appendix A gives a simple C source code example.
Table 3. Block swap memory configuration example
Flash Sectors | |
Sector Number | Data Flash Address |
0 | 0xF000-0xF3FF |
1 | 0xE000-0xE3FF |
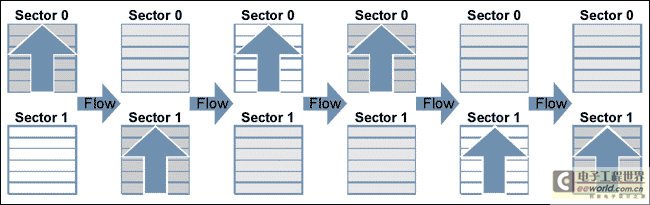
Figure 2. Block exchange process
Bounded Queues and Block Swapping Together
The most reliable and flexible way to manage data flash is to use bounded queues and block swapping together. Combining these two techniques is very beneficial when small amounts of data need to be stored in flash periodically while maintaining data integrity. Table 4 shows an example of two 2K x 16 partitions, each divided into 32 equal entries. Figure 3 shows the flow of data between two partitions within a bounded queue.
The program for this combined method is slightly more complicated than the bounded queue method. Appendix A gives a simple C source code example.
Table 4. Block switching and bounded queue memory configuration examples
|
|
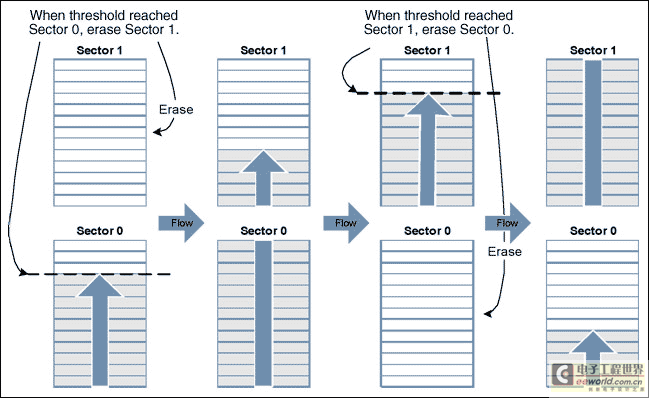
Figure 3. Bounded queue and block exchange process
Application ROM Flash Routines
The MAXQ microcontrollers have on-chip flash support routines that reside in ROM (read-only memory) for programming, erasing, and verifying the flash memory. There are two ways to call these routines. The first and fastest way is direct access, which requires only a header file to be provided with the following code:
u16 flashEraseSector(void *); u16 flashEraseAll(void); u16 flashWrite(u16 *pAddress, u16 iData);Then, add link definitions to assign appropriate addresses to each routine. For an IAR link file, add the following lines:
-DflashEraseSector=0x8XXX -DflashEraseAll=0x8XXX -DflashWrite=0x8XXXWhen used, replace 0x8XXX with the memory address of each routine. Other compilers may use different methods to add these declarations.
Note that the direct access method is not forward compatible with future ROM versions.
The second method is a table lookup. Although this method is more compatible, it takes longer to execute. After each routine description below, the assembly routine uses a table lookup to obtain the address of the ROM application routine. Table 5 shows several flash routines provided by the application ROM. For a complete list of ROM application routines, refer to the user's guide for the specific MAXQ device.
Table 5. Application ROM Flash Examples
Routine Number | Routine Name |
Entry Point
ROMTable = ROM[800Dh] |
Entry Point
Physical Address |
2 | flashEraseSector | ROM[ROMTable + 1] | 0x8XXX |
3 | flashEraseAll | ROM[ROMTable + 2] | 0x8XXX |
15 | flashWrite | ROM[ROMTable + 14] | 0x8XXX |
flashWrite
Routine | u16 flashWrite(u16 *pAddress, u16 iData) |
Summary | Programs a single word of flash memory. |
Inputs |
A[0] - Word address in flash memory to which to write.
A[1] - Word value to write to flash memory. |
Outputs |
Carry: Set on error and cleared on success. If set, then A[0] contains one of the following error codes:
1 : failure due to software timeout 2 : failure reported by hardware (DQ5/FERR) 4 : command not supportedSW_FERR - Set on error, cleared on success. |
Notes | The watchdog must not be active, or the watchdog timeout must be set long enough to complete this routine without triggering a reset. |
The following assembly code example uses the indirect addressing method (table lookup) to call the flashWrite() application routine. This routine can be called from C code.
; This routine is callable by C code using the following prototype ; u16 flashWrite(u16 *pAddress, u16 iData); ; flashWrite: move APC, #0 ; No auto inc/dec of accumulator. move AP, #2 ; Set ACC to A[2] move DP[0], #0800Dh ; This is where the address of the table is stored. move ACC, @DP[0] ; Get the location of the routine table. add #14; Add the index to the flashWrite routine. move DP[0], ACC move ACC, @DP[0] ; Retrieve the address of the routine. call ACC; Execute the routine. ret ; Status returned in A[0]flashEraseSector
Routine | u16 flashEraseSector(void *pAddress) |
Summary | Erases a single sector of flash memory |
Inputs | A[0] - Address located in the sector to erase. |
Outputs |
Carry: Set on error and cleared on success. If set, then A[0] contains one of the following error codes:
1 : failure due to software timeout 2 : failure reported by hardware (DQ5/FERR) 4 : command not supported SW_FERR - Set on error, cleared on success. |
Notes | The watchdog must not be active, or the watchdog timeout must be set long enough to complete this routine without triggering a reset. |
; This routine is callable by C code using the following prototype ; u16 flashEraseSector(void *pAddress); ; flashEraseSector: move APC, #0 ; No auto inc/dec of accumulator. move AP, #1 ; Set ACC to A[1] move DP[0], #0800Dh ; This is where the address of the table is stored. move ACC, @DP[0] ; Get the location of the routine table. add #1; Add the index to the flashEraseSector routine. move DP[0], ACC move ACC, @DP[0] ; Retrieve the address of the routine. call ACC; Execute the routine. ret ; Status returned in A[0]flashEraseAll
Routine | void flashEraseAll(void) |
Summary | Erases the entire program and data flash memory, including the boot loader sector. This routine is not normally used for IAP, as great care must be taken to ensure that the erase/programming sequence is not interrupted. |
Inputs | None |
Outputs | Carry: Set on error and cleared on success.SW_FERR: Set on error, cleared on success. |
Notes | The watchdog must not be active, or the watchdog timeout must be set long enough to complete this routine without triggering a reset. |
; This routine is callable by C code using the following prototype ; void flashEraseAll(void); ; flashEraseAll: move APC, #0 ; No auto inc/dec of accumulator. move AP, #0 ; Set ACC to A[0] move DP[0], #0800Dh ; This is where the address of the table is stored. move ACC, @DP[0] ; Get the location of the routine table. add #2; Add the index to the flashEraseAll routine. move DP[0], ACC move ACC, @DP[0] ; Retrieve the address of the routine. call ACC; Execute the routine. ret
In-application programming
Most flash-based systems require the ability to upgrade the firmware after the system is installed in the end product. This capability is called In-Application Programming (IAP). This section outlines the essentials for creating an IAP application.The application ROM flash routines listed above perform all the erase and write operations required for the flash ROM, allowing user code to operate on the flash memory. Similar to other subroutine calls, control returns to the user code after the program execution is completed.
To achieve reliable IAP, the bootloader must be separated from the main program. This ensures that the programming process can be restarted after an unfinished programming.
Bootloader
After initialization, the entry point of the bootloader should be 0x0000, because the ROM jumps to address 0x0000. The size of the boot flash partition varies from MAXQ device to device. The bootloader can be extended to multiple flash partitions as needed, and the user application code cannot use any partition that is already occupied. Specific requirements that must be met when erasing and writing flash are shown in Table 6.
Table 6. Requirements for calling the application ROM flash routine
You cannot erase or program from the same flash sector from which you are executing code. This is not normally a problem since the flash Boot Sector should never be erased during IAP. |
The watchdog must not be enabled or the watchdog timeout must be set long enough to complete this routine without triggering a reset before the flashEraseSector() routine is called. If the watchdog time out occurs before the erase is complete, it will reset the part. Erasing a sector typically takes 0.7 seconds; it can take up to 15 seconds under worst case conditions. |
Since the System Control Register bit SC.UPA must be set to 0 to access the Utility ROM, a ROM Utility routine cannot be called directly from program memory addresses = 0x8000. If access to a Utility ROM routine is required from a program in upper memory (= 0x8000), the program must indirectly call the ROM routine through a routine residing in lower memory (<0x8000). This effectively limits the boot loader to = 64kB (32kB x 16). |
The flow chart in Figure 4 illustrates the operation of the MAXQ out of reset. After the ROM performs self-diagnostics and determines that the flash memory is ready, the ROM initialization code jumps directly to address 0x0000. Please read the relevant data sheet and user's guide to determine whether your MAXQ microcontroller follows this startup sequence.
Figure 4. ROM initialization process diagram
Figure 5 shows the flow chart of a simple bootloader. When implementing IAP using a bootloader, the entry point for the main application is typically located at address 0x2000 + header offset for a 16kB (8K x 16) bootloader, or at address 0x4000 + header offset for a 32kB (16K x 16) bootloader. A simple application header is shown below:
typedef struct { u16 iSize; // The size of the application in words u32 iCRC; // The CRC of the application u8 ID[8]; // ID string for current application } APPLICATION_HEADER;The boot loader can use the information provided in this header to determine the validity of the main program and, if necessary, report its version identification.
Figure 5. Flash boot loading process diagram
The programming process itself is very simple. First, each sector containing the main program code is erased by calling flashEraseSector(). Then, the code words to be programmed are written word by word by calling flashWrite(). The block containing the application header should be erased first, and the CRC data should be programmed last to minimize the possibility of a false CRC match. Here is a simple routine to flash a microcontroller by getting data through the serial port:
/* // VerySimpleReFlash() // As simple as it gets. // Step 1. Wait for erase command, then erase flash. // Step 2. Wait for program command, then program flash one word // at a time. */ void VerySimpleReFlash() { u16 iStatus; // The status returned from flash utility ROM calls u16 iSize; // The size of the main code to program u16 *pAddress = 0x2000; // The starting address of the main application InitializeCOMM(); // Can be CAN or UART WaitForEraseCommand(); SlowDownWatchdog(); // If watchdog enabled set update > 15s iStatus = flashEraseSector(C_ADDRESS_SECTOR_1); if (iStatus == 0) iStatus = flashEraseSector(C_ADDRESS_SECTOR_2); UpdateWatchdog(); // Prevent watchdog timeout SendFlashErasedResponse(iStatus); if (iStatus) ResetMicro(); iSize = WaitForProgramCommand(); while (iSize--) { u16 iData = GetWordFromCOMM(); iStatus = flashWrite(pAddress, iData); if (iStatus) break; ++pAddress; UpdateWatchdog(); // Prevent watchdog timeout } SendFlashWriteResponse(iStatus); ResetMicro(); }Program space not used by the bootloader can be used for other routines and/or constant storage. A good example would be to store here all subroutines that indirectly call into the Application ROM routines, such as some of the subroutines shown above in the "Application ROM Flashing Routine". One thing to note when using the bootloader partition to store other information is that it cannot be erased without partially or completely erasing the bootloader itself.
Implementing IAP Using a RAM-Based Flash RoutineWhen
fault recovery is not required, a RAM-based flash routine can be used to flash the MAXQ microcontroller.This method requires the main program to copy a small, relocatable flash programming routine into RAM and then jump to that routine.Table 7 lists several limitations that need to be noted when executing code from RAM.
Table 7. Restrictions on executing code from RAM
SC.UPA must be set to 0 before executing a RAM-based routine. This means that the application must jump to the RAM routine from the code segments P0 & P1. |
RAM cannot be accessed as data and program at the same time. This means that only the registers and hardware stack are available for data storage. |
The Interrupt Vector must point to a RAM routine if interrupts are enabled. Typically interrupts are turned off and polling is used due to the simplicity of the RAM reflash routine. |
The flash routine typically communicates via a UART or CAN interface. For a more robust error recovery mechanism, it is best to receive small packets and send some type of acknowledgement. Figure 6 shows an update routine. Remember that the microcontroller will need to be reprogrammed through the JTAG port before powering down if reprogramming is not successfully completed.
Figure 6. Simplified RAM update routine flow chart
Previous article:Getting Started with MAX-IDE
Next article:Building a Remote Key Based on the MAXQ3212
- High signal-to-noise ratio MEMS microphone drives artificial intelligence interaction
- Advantages of using a differential-to-single-ended RF amplifier in a transmit signal chain design
- ON Semiconductor CEO Appears at Munich Electronica Show and Launches Treo Platform
- ON Semiconductor Launches Industry-Leading Analog and Mixed-Signal Platform
- Analog Devices ADAQ7767-1 μModule DAQ Solution for Rapid Development of Precision Data Acquisition Systems Now Available at Mouser
- Domestic high-precision, high-speed ADC chips are on the rise
- Microcontrollers that combine Hi-Fi, intelligence and USB multi-channel features – ushering in a new era of digital audio
- Using capacitive PGA, Naxin Micro launches high-precision multi-channel 24/16-bit Δ-Σ ADC
- Fully Differential Amplifier Provides High Voltage, Low Noise Signals for Precision Data Acquisition Signal Chain
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- Micropython version that supports MCPWM function
- How to solve the problem of No rule to make target when developing zynq using xilinx SDK
- Design of control system for CNC engraving machine based on embedded system
- Development environment installation (II) Cross-compilation environment
- Interpretation and test application of IEC harmonic standards
- VICOR engineers invite you to chat: How to improve the throughput and running time of automatic test equipment?
- The price has increased by at least 200 times! Identification of popular chip material numbers from TI, ST, Infineon, and Qualcomm
- About MSP430-Timer WDT
- Does anyone know the pin map of the YC1021 chip?
- Why Japan failed to kill South Korea's semiconductor industry