mov r1, #2
cmp r1, #1
@Now jump to func1. After executing func1, the program cannot return. If you use bl to jump, the program will return.
@b func1
@At this time, use bl to jump to func1 for execution. After func1 is executed, it will return to execute the following statement
bl func1
mov r1, #2
cmp r1, #3
func1:
mov r1, #2
cmp r1, #2
mov r1, #4
cmp r1, #6
4. Shift instructions
(1) LSL instruction
LSL instruction introduction: logical left shift instruction;
-- Syntax format: Rx, LSL#2;
-- Syntax analysis: shift the value in the Rx register left by 2 bits;
Code example:
.text .global _start _start: @lsl left shift instruction example mov r1, #0b1 @Shift the value in r1 left by 2 bits and put it into register r1 mov r1, r1, lsl#2
(2) ROR instruction
ROR instruction introduction: circular right shift instruction;
-- Syntax format: Rx, ROR#2;
-- Syntax analysis: Circularly shift the value in the Rx register right by 2 bits;
Code example:
.text .global _start _start: @ror Rotate right instruction example mov r1, #0b11 @The result is ob1000...0001 mov r1, r1, ror#1
5. Program status word access instructions
Program status word: CPSR and SPSR;
-- Note: The program status word cannot be accessed using general register statements such as MOV, etc. It must be read and written using the program status register's dedicated instructions;
Code example:
.text .global _start _start: @mrs directive example @rs is s -> r, sr is r -> s mrs r0, cpsr @Move the data in cpsr to r0 orr r0, #0b100 @Set the third bit in cpsr to 1 msr cprs, r0
6. Memory access instructions
(1) STR instruction
STR instruction introduction: save the value in the register to the memory;
-- Syntax format: str r0, address;
-- Syntax parsing: Save the value in register R0 to the memory address;;
Code example:
.text .global _start _start: @str directive example mov r0, #0xff @Change r1 value to 50000000 (OK-6410) str r0, [r1]
-- Debug: Add address monitoring and monitor in Memory view;
(2) LDR instruction
LDR instruction introduction: save the value in the register to the memory;
-- Syntax format: ldr r0, address;
-- Syntax parsing: load the value stored in the memory address into r0;
Code example:
@ldr directive example mov r0, #0xff @Change r1 value to 50000000 (OK-6410) str r0, [r1] ldr r0, [r1]
7. All code examples above
All the above code examples: easy to debug and learn;
.text
.global _start
_start:
@ldr directive example
mov r0, #0xff
@Change r1 value to 50000000 (OK-6410)
str r0, [r1]
ldr r0, [r1]
@str directive example
mov r0, #0xff
@Change r1 value to 50000000 (OK-6410)
str r0, [r1]
@mrs msr command example
@rs is s -> r, sr is r -> s
mrs r0, cpsr @Move the data in cpsr to r0
orr r0, #0b100 program entry, usage ".globol _start", note the dot in front; @ sets the third position in cpsr to 1
msr cprs, r0
@ror Rotate right instruction example
mov r1, #0b11
@The result is ob1000...0001
mov r1, r1, ror#1
@lsl left shift instruction example
mov r1, #0b1
@Shift the value in r1 left by 2 bits and put it into register r1
mov r1, r1, lsl#2
@bl Branch instruction example with connection
mov r1, #2
cmp r1, #1
@Now jump to func1. After executing func1, the program cannot return. If you use bl to jump, the program will return.
@b func1
@At this time, use bl to jump to func1 for execution. After func1 is executed, it will return to execute the following statement
bl func1
mov r1, #2
cmp r1, #3
func1:
mov r1, #2
cmp r1, #2
mov r1, #4
cmp r1, #6
@b branch instruction example
mov r1, #6
mov r2, #5
cmp r1, r2 @Compare the values in r1 and r2
@b can be followed by a condition. {condition} in {} can be added or not. If there is no condition, it will be executed unconditionally 100%.
@gt is a greater than condition instruction. If the condition is met, it will jump to branch1. If not, it will execute the following instruction.
bgt branch1
add r3, r1, r2
b end @In order not to execute branch1 operation here, jump directly to end execution
branch1:
sub r3, r1, r2
end:
nop
@cmp directive example
mov r1, #0b101
tst r1, #0b001 @The bitwise AND result is 0b1, the result is not 0, CPSR Z = 0
mov r1, #0b101
tst r1, #0b10 @The bitwise AND result is 0, the result is not
@cmp directive example
mov r1, #2
cmp r1, #1
mov r1, #2
cmp r1, #3
mov r1, #2
cmp r1, #2
@bic directive examples
mov r1, #0b101011
bic r2, r1, #0b101 @ Clear bits 0 and 2 of r1
@and Directive Examples
mov r1, #5
and r2, r1, #0
mov r1, #5
mov r2, r1, #1
@add directive example
mov r2, #1
add r1, r2, #3
@mov command example
mov r1, #8 @Assign 8 to r1
mov r2, r1 @assign the value in r1 to r2
mov r3, #10 @Assign 10 to register r3
@mvn command example
mvn r1, #0b10 @0b10 Binary number inversion, assign to r1
mvn r2, #5 @5 decimal number inversion, assign to r2
mvn r3, r1 @Assign the value of register r1 to r3
@sub directive examples
@sub r1, #4, #2 Incorrect example, the subtraction number cannot be an immediate number, it must be a register
mov r2, #4
sub r1, r2, #4
mov r0, #1
sub r3, r1, r0
3. ARM pseudo instructions
Reference document: ARM document Page 110, which is available for download.
1. ARM machine code
(1) Machine code disassembly example
Assembler execution process: assembly code--> assembler--> machine code--> CPU runs;
Disassembly example: find an elf file and use arm-linux-objdump to disassemble it;
-- Command: Use arm-linux-objdump -S -D start.elf command to disassemble, where "e3a01001" in "50008000: e3a01001 mov r1, #1 ; 0x1" is the machine code, as shown in the marked part below;
--Disassembly results:
[root@localhost 04_assembly]# arm-linux-objdump -S -D start.elf start.elf: file format elf32-littlearm Disassembly of section .text: 50008000 <_start>: .text .globl _start _start: mov r1,#1 50008000: e3a01001 mov r1, #1 ; 0x1 mov r2,#2 50008004: e3a02002 mov r2, #2; 0x2 mov r3,#3 50008008: e3a03003 mov r3, #3; 0x3 Disassembly of section .debug_aranges:
(2) Machine code format
Machine code format: Screenshot from arm document P110;
-- ARM machine code bit number: 32 bits;
-- Machine code segment:
(3) Parsing the MOV instruction machine code
Code preparation:
-- Assembly code:
.text .globl _start _start: mov r0, r1 moveq r0, #0xff
-- Makefile script:
all:start.o arm-linux-ld -Ttext 0x50008000 -o start.elf $^ %.o:%.S arm-linux-gcc -g -o $@ $^ -c clean: rm -rf *.o *.elf
Disassemble the elf file:
-- Disassembled content: Omit most of the following;
[root@localhost 04_assembly]# arm-linux-objdump -S -D start.elf start.elf: file format elf32-littlearm Disassembly of section .text: 50008000 <_start>: .text .globl _start _start: mov r0, r1 50008000: e1a00001 mov r0, r1 moveq r0, #0xff 50008004: 03a000ff moveq r0, #255; 0xff Disassembly of section .debug_aranges:
Compiled machine code:
-- "mov r0, r1" : hex 0xe1a00001, binary 111000011010000000000000000000001;
-- "moveq r0, #0xff" : hex 0x03a000ff, binary 00000011101000000000000011111111;
Machine code analysis:
First: 1110 00 0 1101 0 0000 0000 000000000001
Article 2: 0000 00 1 1101 0 0000 0000 000011111111
-- Condition bit comparison (first paragraph 31 ~ 28): the first one is 1110 corresponding to AL always executed, the second one is 0000 corresponding to EQ;
-- Comparison of reserved bits (second paragraph 27 ~ 26): the first line is 00, the second line is 00, obviously the same;
-- I operand type identification bit (third paragraph 25): marks whether the last stored immediate value or register, if it is 0, it means register, if it is 1, it means immediate value;
-- Operation code bit (the fourth segment 24 ~ 21): distinguish different instructions, 1101 is the MOV instruction;
-- S Status register change flag (fifth paragraph 20): whether to affect the CPSR register, if S = 0, no effect, if S = 1, effect;
-- Rn source operation register (sixth paragraph 19 ~ 16): MOV and MVN do not use the Rn bit, register number;
-- Rd destination operation register (seventh segment 15 ~ 12): register number;
-- shifter_operand source operation book (section 8, 11 ~ 0): source operand, this is combined with the I bit, if I = 0, this bit indicates the register number, if I = 1, this bit indicates the size of the immediate value, the immediate value has a range, if it exceeds the range, an error will be reported, here you need to use a pseudo instruction;
(4) Machine code related documents
Related Documents:
-- Bit number document: P116, The ARM Instruction Set, A3.4.1 Instruction encoding;
-- MOV and MVN instructions: machine code format "
-- Conditional bit documentation: Page 112, The ARM Instruction Set, A3.2.1 Condition code 0b1111;
2. Pseudo-instructions
Introduction to pseudo-instructions: Pseudo-instructions have no corresponding machine code. This type of instruction only works during compilation. Pseudo-instructions need to be converted into other assembly instructions to run, such as defining macros, and will not generate machine code;
(1) globol directive
globol pseudo-instruction introduction:
--Pseudo-instruction function: used to define the program entry, usage ".globol _start", note the dot in front;
-- Code example:
.text .global _start _start: @lsl left shift instruction example mov r1, #0b1 @Shift the value in r1 left by 2 bits and put it into register r1 mov r1, r1, lsl#2
(2) data acsii byte word pseudo instruction
Previous article:Camera driver based on WINCE6.0+S3C2443
Next article:[Embedded Development] Introduction to ARM chips (ARM chip types | ARM processor working modes | ARM registers | ARM addressing)
Recommended ReadingLatest update time:2024-11-24 18:00
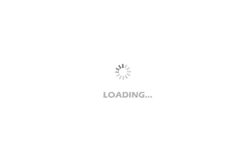
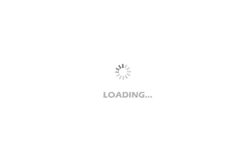
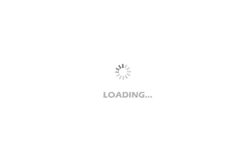
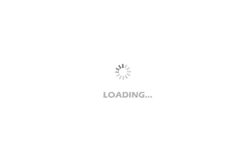
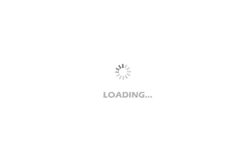
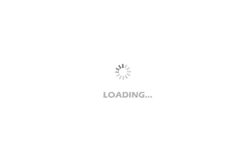
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- 【ESP32-S2-Kaluga-1 Review】Getting weather information via https
- LE audio, Bluetooth positioning, Bluetooth mesh... Where will the next technological explosion point of Bluetooth be? ?
- Introduction to the assembly process of FPC flexible circuit board
- RAM is equivalent to the computer's running memory. Can the memory stick also be expanded?
- LPS25HB Barometric Pressure Sensor PCB Package and Code
- Test Technology of Analog Integrated Circuits
- Can the glass fiber effect be quantified through simulation?
- In Cadence, I want to reserve space for components in PCB design. How do I do it in the principle?
- Circuit Schematic Analysis
- Does anyone know about the FDS9435 chip?