1. ARM Assembly Overview
1. Assembly usage location
Compilation location:
-- Startup code: Bootloader initializes the CPU and coprocessor, etc., and the C language operating environment is not established at this time. At this time, assembly language is used to perform initialization operations;
-- Efficiency requirements: high assembly efficiency. In the Linux kernel, assembly is required where there are special requirements for efficiency.
2. Compilation classification
(1) ARM standard assembly
ARM Standard Assembly Introduction:
-- Usage scenario: Applicable to ARM's assembler, suitable for use on Windows platform, such as ADS;
(2) GNU Assembler
GNU Assembler Introduction:
-- Usage scenario: Assembler for cross-compilation toolchain on Linux platform;
3. ARM assembler framework
ARM Assembly Framework:
-- ARM assembly framework example:
.section .data.section .bss .section .text .global _start _start:
-- Program entry: "_start:" is the entry of the assembler program, equivalent to main();
-- Mark the entry: Use ".global _start" to mark the program entry so that the outside world can recognize that this is the program entry;
-- Mark the code section: ".section .text" marks this as a code section;
-- Mark the bss segment: Use ".section .bss" to mark the bss segment. If there is no bss segment and data segment, start directly from .text;
4. Build an assembly development and debugging environment
(1) Assembler preparation
Program code:
-- Define code section: .text;
-- Define program entry: .globl _start;
-- Code example:
.text .globl _start _start: mov r1,#1 mov r2,#2 mov r3,#3
Makefile code:
-- Link elf format file: Set the program start position 6410 board is 0x50008000 address;
-- Specify the program start address in arm-linux-ld: -Ttext 50008000;
-- If you use a linker script to specify the address: Note that the third line specifies the program start address;
SECTIONS { . = 0x50008000; . = ALIGN(4); .text : { led.o (.text) *(.text) } . = ALIGN(4); .rodata : { *(SORT_BY_ALIGNMENT(SORT_BY_NAME(.rodata*))) } . = ALIGN(4); .data : { *(.data) } . = ALIGN(4); .bss (NOLOAD) : { *(.bss) . = ALIGN(4); } }
-- Code example:
all:start.o arm-linux-ld -Ttext 0x50008000 -o start.elf $^ %.o:%.S arm-linux-gcc -g -o $@ $^ -c clean: rm -rf *.o *.elf
(2) Start JLink debugging
JLink debug start:
-- Ensure the driver is installed: Note that you need to install the Windows driver;
-- Connect JLink: Connect JLink in the lower right corner of the virtual machine;
-- Start JLinkGDBServer:
[root@localhost JLink_Linux_V434a]# ./JLinkGDBServer SEGGER J-Link GDB Server V4.34a JLinkARM.dll V4.34a (DLL compiled Aug 31 2011 11:51:40) Listening on TCP/IP port 2331 J-Link connected Firmware: J-Link ARM V8 compiled Aug 24 2011 17:23:32 Hardware: V8.00 S/N: 17935099 Feature(s): RDI,FlashDL,FlashBP,JFlash J-Link found 2 JTAG devices, Total IRLen = 5 JTAG ID: 0x07B76F0F (ARM11)
(2) Eclipse debugging environment
Build eclipse debugging environment:
-- Import project: Select Makefile Project With Existing Code;
-- Select the imported code location:
-- clean code: select "Project" --> "Clean";
-- Build project: Select "Menu" --> Project --> Build All option;
--Configure Debug parameters:
-- Execute debugging: Press F6 to step through the debugging. You can view the register values in the Register view. You can see that r1 and r2 are assigned 1 and 2.
2. ARM instruction classification
ARM Assembly Manual:
The difference between GNU assembly and ARM standard assembly: The manual above is the ARM standard assembly manual, and we wrote the GNU assembly manual, which is somewhat different;
-- Case difference: ARM standard assembly is all uppercase, GNU assembly can be lowercase;
1. Arithmetic and logical instructions
(1) MOV instruction
Introduction to MOV instruction: assignment operation;
-- Syntax format: MOV
-- Syntax analysis: dest is the destination register, op1 can be an immediate value, a register, an address, etc., which is equivalent to dest = op1;
Assembler comments: Use the "@" symbol to add comments in the assembly;
Sample code:
.text .global _start _start: @mov command example mov r1, #8 @Assign 8 to r1 mov r2, r1 @assign the value in r1 to r2 mov r3, #10 @Assign 10 to register r3
(2) MVN command
MVN instruction introduction: negate assignment operation;
-- Syntax format: MVN
-- Syntax analysis: Invert the operand op1 and assign it to dest;
Command example:
-- Code:
.text .global _start _start: @mvn command example mvn r1, #0b10 @0b10 Binary number inversion, assign to r1 mvn r2, #5 @5 decimal number inversion, assign to r2 mvn r3, r1 @Assign the value of register r1 to r3
(3) SUB instruction
SUB instruction introduction: subtraction operation;
-- Syntax format: SUB
-- Syntax analysis: dest stores the subtraction result, op1 is the subtrahend, op2 is the minuend, dest = op1 - op2;
-- Note: dest op1 cannot use immediate data, but op2 can use immediate data;
Code example:
.text .global _start _start: @sub directive examples @sub r1, #4, #2 Incorrect example, the subtraction number cannot be an immediate number, it must be a register mov r2, #4 sub r1, r2, #4 mov r0, #1 sub r3, r1, r0
(4) ADD instruction
ADD instruction introduction: addition operation;
-- Syntax format: ADD
-- Syntax analysis: dest stores the result of addition, op1 and op2 are the two numbers to be added, dest = op1 + op2;
-- Note: dest op1 cannot use immediate data, but op2 can use immediate data;
Code example:
@add directive example mov r2, #1 add r1, r2, #3
(5) AND instruction
AND instruction introduction: logical and operation;
-- Syntax format: AND
-- Syntax analysis: dest stores the result of logical AND operation, op1 and op2 are two numbers to be ANDed, dest = op1 & op2;
-- Note: dest op1 cannot use immediate data, must use registers, op2 can use immediate data;
Code example:
.text .global _start _start: @and Directive Examples mov r1, #5 and r2, r1, #0 mov r1, #5 mov r2, r1, #1
(6) BIC instruction
BIC instruction introduction: bit clear instruction operation;
-- Syntax format: AND
-- Syntax analysis: dest stores the result of clearing the bit, op1 is the object to be cleared, and op2 is the mask;
-- Example: "bic r0, r0, #0b1011", clear bits 0, 1, and 3 in r0, keep the rest of the bits unchanged, and put the result in r0;
-- Note: dest op1 cannot use immediate data, must use registers, op2 can use immediate data;
-- Binary representation: % in the mask represents binary in standard assembly, but cannot be used in GNU assembly. GNU assembly uses 0b to represent binary;
Code example:
.text .global _start _start: @bic directive examples mov r1, #0b101011 bic r2, r1, #0b101 @ Clear bits 0 and 2 of r1
2. Comparison Instructions
(1) CMP instruction
CMP instruction introduction: comparison instruction;
-- Syntax format: CMP
-- Syntax analysis: There are three comparison results: op1 > op2 (CPSR N = 0), op1 = op2 (CPSR Z = 1), op1 < op2 (CPSR N = 1), the result is placed in the CPSR register;
Code example:
.text .global _start _start: @cmp directive example mov r1, #2 cmp r1, #1 mov r1, #2 cmp r1, #3 mov r1, #2 cmp r1, #2
(2) TST instruction
TST instruction introduction: comparison instruction;
-- Syntax format: TST
-- Syntax analysis: op1 and op2 are bitwise ANDed, the result affects the CPSR register, if the result is not 0, CPSR's Z = 0, if the result is 0, Z = 1;
Code example:
.text .global _start _start: @cmp directive example mov r1, #0b101 tst r1, #0b001 @The bitwise AND result is 0b1, the result is not 0, CPSR Z = 0 mov r1, #0b101 tst r1, #0b10 @The bitwise AND result is 0, the result is not
3. Branch Instructions
(1) B instruction
B Instruction Introduction: Branch instruction;
-- Syntax format: B{condition} address;
-- Syntax analysis: If the condition is met, jump to the address location, if the condition is not met, execute the following statement, if there is no condition, it is 100% executed;;
Code example:
-- Conditional analysis: gt is a greater than condition, if r1 > r2, go to the conditional branch, otherwise continue to execute the next one;
.text
.global _start
_start:
@b branch instruction example
mov r1, #6
mov r2, #5
cmp r1, r2 @Compare the values in r1 and r2
@b can be followed by a condition. {condition} in {} can be added or not. If there is no condition, it will be executed unconditionally 100%.
@gt is a greater than condition instruction. If the condition is met, it will jump to branch1. If not, it will execute the following instruction.
bgt branch1
add r3, r1, r2
b end @In order not to execute branch1 operation here, jump directly to end execution
branch1:
sub r3, r1, r2
end:
nop
(2) BL instruction
BL instruction introduction: branch instruction with connection;
-- Syntax format: BL{condition} address;
-- Syntax analysis: If the condition is met, jump to the address location, if the condition is not met, execute the following statement, if there is no condition, it is 100% executed;;
Code example:
.text
.global _start
_start:
@bl Branch instruction example with connection
Previous article:Camera driver based on WINCE6.0+S3C2443
Next article:[Embedded Development] Introduction to ARM chips (ARM chip types | ARM processor working modes | ARM registers | ARM addressing)
Recommended ReadingLatest update time:2024-11-21 22:44
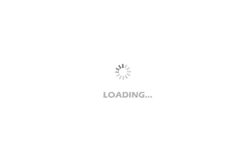
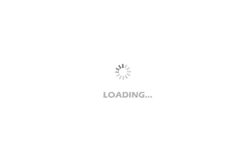
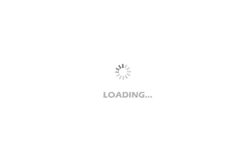
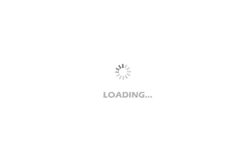
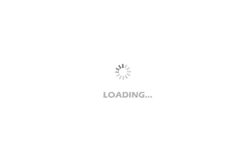
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- Europe's three largest chip giants re-examine their supply chains
- Breaking through the intelligent competition, Changan Automobile opens the "God's perspective"
- The world's first fully digital chassis, looking forward to the debut of the U7 PHEV and EV versions
- Design of automotive LIN communication simulator based on Renesas MCU
- When will solid-state batteries become popular?
- Adding solid-state batteries, CATL wants to continue to be the "King of Ning"
- The agency predicts that my country's public electric vehicle charging piles will reach 3.6 million this year, accounting for nearly 70% of the world
- U.S. senators urge NHTSA to issue new vehicle safety rules
- Giants step up investment, accelerating the application of solid-state batteries
- Guangzhou Auto Show: End-to-end competition accelerates, autonomous driving fully impacts luxury...
- "Playing with the Board" + Replaying MicroPython on the STM32F7DISC (3)
- Make Magazine: The Rise of Python and the Microcontroller of the Year
- Series touch screen (registered version, copy version)
- 【GD32450I-EVAL】+ 05FreeRTOS porting and task creation
- Can't find the startup file for STM32F722
- stm32 got stuck halfway through downloading
- [Zero-knowledge ESP8266 tutorial] Quick start 27 Use of ADXL345 sensor module
- 【LPC8N04 Review】6. Installation and use of KEIL IDE
- I need a total output of 3.3V 15A
- Panasonic doubles profits in 2010 thanks to automotive electronics market