STC Experiment Box 4
IAP15W4K58S4
Keil uVision V5.29.0.0
PK51 Prof.Developers Kit Version:9.60.0.0
Hard Knowledge
Hardware knowledge
Excerpted from "STC15 Series MCU Device Manual"
Some STC15 series MCUs integrate 3-channel programmable counter array (CCP/PCA) modules (STC15W4K32S4 series MCUs have only 2-channel CCP/PCA), which can be used for software timer, external pulse capture, high-speed pulse output and pulse width modulation (PWM) output.
The following table summarizes the STC15 series MCU models that integrate CCP/PCA/PM functions:
In the above table, √ indicates that the corresponding series has the corresponding function.
Structure of CCP/PWM/PCA module
Some MCUs in the STC15 series have 3-channel programmable counter arrays CCP/PCA/PWM (CCP/PCA/PWM can be set to switch from port P1 to port P2 to port P3 through the AUXR1/P_SW1 register).
The PCA contains a special 16-bit timer with three 16-bit capture/compare modules connected to it, as shown in the figure below.
Each module can be programmed to work in 4 modes: rising/falling edge capture, software timer, high-speed pulse output or modulated pulse output.
The 16-bit PCA timer/counter is the common time base for the three modules, and its structure is shown in the figure below.
Software knowledge
Excerpted from "STC Library Function Usage Reference"
PCA initialization function
PCA_Init
PCA_id: Select the PCA channel to initialize:
The definition of PCA_InitTypeDef is found in the file "PCA.H".
typedef struct
{
u8 PCA_IoUse;
u8 PCA_Clock;
u8 PCA_Mode;
u8 PCA_PWM_Wide;
u8 PCA_Interrupt_Mode;
u8 PCA_Polity;
u16 PCA_Value;
} PCA_InitTypeDef;
PCA_IoUse: Select the IO used by PCA: the value when initializing PCA_Counter, ignored when initializing PCA0~PCA2
PCA_Clock: Select the clock used by PCA: the value used when initializing PCA_Counter, ignored when initializing PCA0~PCA2
PCA_Mode: Set the working mode of the PCA channel: the value when initializing PCA0~PCA2, ignored when initializing PCA_Counter
PCA_PWM_Wide: Set the PWM width when the PCA channel works in PWM mode: Initialize the value of PCA0~PCA2 when working in PWM mode, ignore when initializing PCA_Counter or other modes of PCA channel
PCA_Interrupt_Mode: interrupt enable or disable: value when initializing PCA0~PCA2, ignored when initializing PCA_Counter
Note: The above parameters can be combined as follows:
PCA_InitStructure.PCA_Interrupt_Mode = PCA_Fall_Active | ENABLE; //Falling edge interrupt, enable interrupt.
PCA_InitStructure.PCA_Interrupt_Mode = PCA_Rise_Active | ENABLE; //Rising edge interrupt, enable interrupt.
PCA_InitStructure.PCA_Interrupt_Mode = PCA_Rise_Active | PCA_Fall_Active | ENABLE; //Rising edge and falling edge interrupts, enable interrupts.
If | DISABLE is used afterwards, the interrupt is disabled.
PCA_Polity: Interrupt priority: value taken when initializing PCA_Counter, ignored when initializing PCA0~PCA2
PCA_Value: Set the initial value of the PCA channel. The value used when initializing PCA0~PCA2, ignored when initializing PCA_Counter
PWM update duty cycle function
UpdatePwm
test program
PWM mode generates PWM signal
The UpdatePwm function in PCA.c is commented out by default and needs to be uncommented:
main.c
#include "./Drivers/config.h"
#include "./Drivers/delay.h"
#include "./Drivers/GPIO.h"
#include "./Drivers/PCA.h"
void GPIO_config(void)
{
GPIO_InitTypeDef GPIO_InitStructure; //Structure definition
GPIO_InitStructure.Mode = GPIO_OUT_PP; //Specify IO input or output mode, GPIO_PullUp, GPIO_HighZ, GPIO_OUT_OD, GPIO_OUT_PP
GPIO_InitStructure.Pin = GPIO_Pin_5 | GPIO_Pin_6; //Specify the IO to be initialized, or operation
GPIO_Inilize(GPIO_P2, &GPIO_InitStructure); //P2.5 and P2.6 are initialized as push-pull output
}
void PCA_config(void)
{
PCA_InitTypeDef PCA_InitStructure;
PCA_InitStructure.PCA_Mode = PCA_Mode_PWM; //PCA_Mode_PWM, PCA_Mode_Capture, PCA_Mode_SoftTimer, PCA_Mode_HighPulseOutput
PCA_InitStructure.PCA_PWM_Wide = PCA_PWM_8bit; //PCA_PWM_8bit, PCA_PWM_7bit, PCA_PWM_6bit
PCA_InitStructure.PCA_Interrupt_Mode = DISABLE; //PCA_Rise_Active, PCA_Fall_Active, ENABLE, DISABLE
PCA_InitStructure.PCA_Value = (u16)((1 << 8) * (1 - 0.5)); //For software timing, it is the match comparison value
PCA_Init(PCA0,&PCA_InitStructure);
PCA_InitStructure.PCA_Mode = PCA_Mode_PWM; //PCA_Mode_PWM, PCA_Mode_Capture, PCA_Mode_SoftTimer, PCA_Mode_HighPulseOutput
PCA_InitStructure.PCA_PWM_Wide = PCA_PWM_7bit; //PCA_PWM_8bit, PCA_PWM_7bit, PCA_PWM_6bit
PCA_InitStructure.PCA_Interrupt_Mode = DISABLE; //PCA_Rise_Active, PCA_Fall_Active, ENABLE, DISABLE
PCA_InitStructure.PCA_Value = (u16)((1 << 7) * (1 - 0.5)); //For software timing, it is the match comparison value
PCA_Init(PCA1,&PCA_InitStructure);
PCA_InitStructure.PCA_Clock = PCA_Clock_1T; //PCA_Clock_1T, PCA_Clock_2T, PCA_Clock_4T, PCA_Clock_6T, PCA_Clock_8T, PCA_Clock_12T, PCA_Clock_Timer0_OF, PCA_Clock_ECI
PCA_InitStructure.PCA_IoUse = PCA_P24_P25_P26_P27;//PCA_P12_P11_P10_P37, PCA_P34_P35_P36_P37, PCA_P24_P25_P26_P27
PCA_InitStructure.PCA_Interrupt_Mode = DISABLE; //ENABLE, DISABLE
PCA_InitStructure.PCA_Polity = PolityHigh; //Priority setting PolityHigh, PolityLow
PCA_Init(PCA_Counter,&PCA_InitStructure);
}
void main(void)
{
GPIO_config();
PCA_config();
UpdatePwm(PCA0, (1 << 8) * (1 - 0.75));
UpdatePwm(PCA1, (1 << 7) * (1 - 0.25));
while(1)
{
}
}
Experimental phenomena
16-bit software timer mode to generate PWM signal
PWM frequency = PCA clock frequency / PWMx_DUTY
Modify PWMx_DUTY in PCA.h
Modify the corresponding pin in PCA_Handler
//========================================================================
// 函数: void PCA_Handler (void) interrupt PCA_VECTOR
// Description: PCA interrupt handler.
// Parameters: None
// Returns: none.
// Version: V1.0, 2012-11-22
//========================================================================
void PCA_Handler (void) interrupt PCA_VECTOR
{
if(CCF0) //PCA module 0 interrupt
{
CCF0 = 0; // Clear PCA module 0 interrupt flag
if(P25) CCAP0_tmp += PCA_Timer0; //output is high level, then load the high level time length to the mapping register
else CCAP0_tmp += PWM0_low; //If the output is low level, load the low level time length into the mapping register
CCAP0L = (u8)CCAP0_tmp; //Write the mapping register into the capture register, write CCAP0L first
CCAP0H = (u8)(CCAP0_tmp >> 8); // write CCAP0H later
}
if(CCF1) //PCA module 1 interrupt
{
CCF1 = 0; // Clear PCA module 1 interrupt flag
if(P26) CCAP1_tmp += PCA_Timer1; //output is high level, then load the high level time length to the mapping register
else CCAP1_tmp += PWM1_low; //If the output is low level, load the low level time length into the mapping register
CCAP1L = (u8)CCAP1_tmp; //Write the mapping register into the capture register, write CCAP0L first
CCAP1H = (u8)(CCAP1_tmp >> 8); //后写CCAP0H
}
if(CCF2) //PCA module 2 interrupt
{
CCF2 = 0; // Clear PCA module 1 interrupt flag
if(P27) CCAP2_tmp += PCA_Timer2; //output is high level, then load the high level time length into the mapping register
else CCAP2_tmp += PWM2_low; //If the output is low level, load the low level time length into the mapping register
CCAP2L = (u8)CCAP2_tmp; //Write the mapping register into the capture register, write CCAP0L first
CCAP2H = (u8)(CCAP2_tmp >> 8); // write CCAP0H later
}
if(CF) //PCA overflow interrupt
{
CF = 0; // Clear PCA overflow interrupt flag
}
}
main.c
#include "./Drivers/config.h"
#include "./Drivers/delay.h"
#include "./Drivers/GPIO.h"
#include "./Drivers/PCA.h"
void GPIO_config(void)
{
GPIO_InitTypeDef GPIO_InitStructure; //Structure definition
GPIO_InitStructure.Mode = GPIO_OUT_PP; //Specify IO input or output mode, GPIO_PullUp, GPIO_HighZ, GPIO_OUT_OD, GPIO_OUT_PP
GPIO_InitStructure.Pin = GPIO_Pin_5 | GPIO_Pin_6; //Specify the IO to be initialized, or operation
GPIO_Inilize(GPIO_P2, &GPIO_InitStructure); //P2.5 and P2.6 are initialized as push-pull output
}
void PCA_config(void)
{
PCA_InitTypeDef PCA_InitStructure;
PCA_InitStructure.PCA_Mode = PCA_Mode_HighPulseOutput; //PCA_Mode_PWM, PCA_Mode_Capture, PCA_Mode_SoftTimer, PCA_Mode_HighPulseOutput
PCA_InitStructure.PCA_PWM_Wide = 0; //PCA_PWM_8bit, PCA_PWM_7bit, PCA_PWM_6bit
PCA_InitStructure.PCA_Interrupt_Mode = ENABLE; //PCA_Rise_Active, PCA_Fall_Active, ENABLE, DISABLE
PCA_InitStructure.PCA_Value = 65535; //For software timing, it is the match comparison value
PCA_Init(PCA0,&PCA_InitStructure);
PCA_InitStructure.PCA_Mode = PCA_Mode_HighPulseOutput; //PCA_Mode_PWM, PCA_Mode_Capture, PCA_Mode_SoftTimer, PCA_Mode_HighPulseOutput
PCA_InitStructure.PCA_PWM_Wide = 0; //PCA_PWM_8bit, PCA_PWM_7bit, PCA_PWM_6bit
PCA_InitStructure.PCA_Interrupt_Mode = ENABLE; //PCA_Rise_Active, PCA_Fall_Active, ENABLE, DISABLE
PCA_InitStructure.PCA_Value = 65535; //For software timing, it is the match comparison value
PCA_Init(PCA1,&PCA_InitStructure);
PCA_InitStructure.PCA_Clock = PCA_Clock_12T; //PCA_Clock_1T, PCA_Clock_2T, PCA_Clock_4T, PCA_Clock_6T, PCA_Clock_8T, PCA_Clock_12T, PCA_Clock_Timer0_OF, PCA_Clock_ECI
PCA_InitStructure.PCA_IoUse = PCA_P24_P25_P26_P27; //PCA_P12_P11_P10_P37, PCA_P34_P35_P36_P37, PCA_P24_P25_P26_P27
PCA_InitStructure.PCA_Interrupt_Mode = DISABLE; //ENABLE, DISABLE
PCA_InitStructure.PCA_Polity = PolityHigh; //Priority setting PolityHigh, PolityLow
PCA_Init(PCA_Counter, &PCA_InitStructure);
}
void main(void)
{
GPIO_config();
PCA_config();
PWMn_Update(PCA0, (u16)(PWM0_DUTY * 0.75));
PWMn_Update(PCA1, (u16)(PWM1_DUTY * 0.25));
EA = 1;
while(1)
{
}
}
Experimental phenomena
Capture mode measures cycle length
Modify PCA_Handler in PCA.c
//========================================================================
// 函数: void PCA_Handler (void) interrupt PCA_VECTOR
// Description: PCA interrupt handler.
// Parameters: None
// Returns: none.
// Version: V1.0, 2012-11-22
//========================================================================
void PCA_Handler (void) interrupt PCA_VECTOR
Previous article:[STC15 library function hands-on notes] 8. Comparator
Next article:[STC15 library function hands-on notes] 6. ADC
Recommended ReadingLatest update time:2024-11-15 05:25
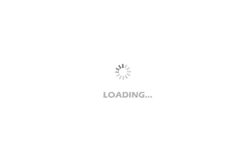
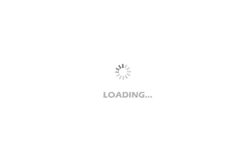
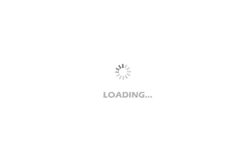
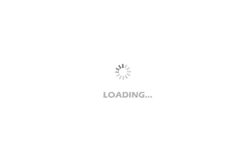
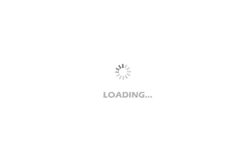
- Popular Resources
- Popular amplifiers
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
A low-latency and low-power PWM comparator circuit design
-
Peak current mode PWM boost switching power supply chip design_Cui Zheyu
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- CGD and Qorvo to jointly revolutionize motor control solutions
- CGD and Qorvo to jointly revolutionize motor control solutions
- Keysight Technologies FieldFox handheld analyzer with VDI spread spectrum module to achieve millimeter wave analysis function
- Infineon's PASCO2V15 XENSIV PAS CO2 5V Sensor Now Available at Mouser for Accurate CO2 Level Measurement
- Advanced gameplay, Harting takes your PCB board connection to a new level!
- Advanced gameplay, Harting takes your PCB board connection to a new level!
- A new chapter in Great Wall Motors R&D: solid-state battery technology leads the future
- Naxin Micro provides full-scenario GaN driver IC solutions
- Interpreting Huawei’s new solid-state battery patent, will it challenge CATL in 2030?
- Are pure electric/plug-in hybrid vehicles going crazy? A Chinese company has launched the world's first -40℃ dischargeable hybrid battery that is not afraid of cold
- Automatic focusing system based on DSP chip TMS320F206 for numerical calculation and implementation control
- NU514-RGBW four-way control constant current linear constant current IC
- Do you know the eight important knowledge points of FPGA design?
- Common usage of MSP430 watchdog and writing method of interrupt function
- MQBoard - MicroPython microframework for managing MQTT
- BT8896A Specification (Zhongke Bluexun)
- Request a Free VICOR UHV BCM Evaluation Sample
- IBM develops 2-nanometer chip
- Crazy Shell AI open source drone ADC (joystick control)
- How to delete the top level in AD