To use the timer/counter interrupt, the following conditions must be met.
1) Interrupts are always enabled EA = 1;
2) The interrupt source is allowed to be turned on ET0 = 1 or ET1 = 1;
3) Set the working mode of the timer/counter (set TMOD)
4) Load the timer and set the initial calculation value of the counter.
5) Start the timer (TR0 = 1 or TR1 = 1 in TCON)
6) Interrupt service function
Through the study of some previous interrupt articles, I believe that except for the 4th point above, using timers/counters is not difficult.
Load the timer. As we know from the previous timer chapter, there are 4 modes for timer/counter. They are 13-bit manual load (mode 0), 16-bit manual load (mode 1), 8-bit auto-reload (mode 2), and 8-bit manual load (mode 3). According to the number of bits of the timer/counter, TH0 (1) and TL0 (1) are required to form the number of bits of the timer/counter.
The input clock pulse is obtained by dividing the output of the crystal oscillator by 12, so the timer is a counter of the machine cycle, each machine cycle +1, that is, the value of the number of bits of TH0 (1) and TL0 (1) that make up the timer/counter is +1 in each machine cycle. When the value overflows, a timer/counter interrupt is generated.
The following uses method 1 and method 2 to perform timing and light up the LED.
Method 1
/****************************************
Header:
File Name: main.c
Author: adam
Date: September 19, 2020
**********************************************/
#include "reg52.h"
#include "stdio.h"
typedef unsigned char uchar;
typedef unsigned int uint;
uint countms = 0;
void DelayMs(uint ms);
void main(){
EA = 1; //General interrupt enabled
ET0 = 1; //Timer T0 interrupt enabled
TMOD = 0x01; //Set to mode 1, timer mode: C/T=0; do not start external INT0 control to start timing: GATE=0,
//Timing 1ms, machine cycle 1us, 1000 machine cycles 1ms
//16-bit timer overflow condition: full value 65535 (#FFFF) + 1 overflows, so timing 1ms requires (65535+1-1000);
// Divide the value into two registers, each of which can hold 256 numbers.
TH0 = (65536-1000)/256; //Load initial value TH
TL0 = (65536-1000)%256; //Load initial value TL
TR0 =1; //Start timer TR0
while(1)
{
DelayMs(1000);
P0=~P0;
}
}
void DelayMs(uint ms)
{
while(countms } void Timer0(void) interrupt 1 { //Reinstall the initial value TH0 = (65535-1000)/256; TL0 = (65535-1000)%256; countms++; //Every ms, countms++ } Method 2 /**************************************** Header: File Name: main.c Author: adam Date: September 19, 2020 **********************************************/ #include "reg52.h" #include "stdio.h" typedef unsigned char uchar; typedef unsigned int uint; uint count = 0; uint countms = 0; void DelayMs(uint ms); void main(){ EA = 1; //General interrupt enabled ET0 = 1; //Timer T0 interrupt enabled TMOD = 0x02; //Set to mode 2, timer mode: C/T=0; do not start external INT0 control to start timing: GATE=0, //Since the mode is only 8 bits, the maximum timing can only be 0.256us, so first time 100us, and then time ms through 100us. TH0 = 256-100; //Load value TH, automatic reload TL0 = 256-100; //Load value TL, overflow generates interrupt. TR0 =1; //Start timer TR0 while(1) { DelayMs(100); P0=~P0; } } void DelayUs(uint us){ while(countus } void DelayMs(uint ms) { while(countms countms++; } countms=0; } void Timer0(void) interrupt 1 { countus++; //every ms, countms++ } Note: In the above two examples, the interrupts are 1ms and 100us respectively. It is not recommended to use the timer to set the interrupt to a smaller time. Although the timer can be set to generate an interrupt in 1us, 1us is only one machine cycle. After the interrupt is responded, the interrupt service function is entered. It may take four machine cycles to execute a simple statement, plus the protection and restoration of the scene, which will take up a long machine cycle and make the timing inaccurate. When GATE = 1, the timer/counter is affected by the INT0/INT1 level. INT0/INT1 = 1 starts counting, and INT0/INT1 = 0 stops counting. When GATE = 1, after the microcontroller is powered on, the initial values of P0~P3 are all high levels, so there is no need to connect an external high level to start the timer/counter. Generally, INT0/INT1 uses the following circuit. When the button is pressed, it is low level and the timer/counter stops counting.
Previous article:C51 Programming 15-Interrupts (Timer Interrupts 2)
Next article:C51 Programming 17-Interrupt (Serial Communication 1)
Recommended ReadingLatest update time:2024-11-16 00:46
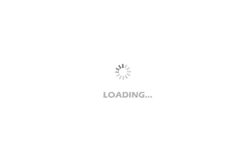
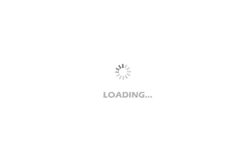
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Please help me write a program to monitor the status of 2 buttons. Is it really that difficult? I just can't figure it out!
- The underlying technology that opens the LPWAN 2.0 era: Advanced M-FSK (Part 2)
- Design of TPS53355 ripple injection circuit
- Ultra-low power multi-sensor data logger with NFC interface
- 【Bluesun AB32VG1 RISC-V Evaluation Board】Development Board Introduction
- How to prevent wage subsidy fraud? All Sohu employees were exposed to wage subsidy fraud
- How to use a power amplifier to amplify and output a pulse train signal? How to use the Burst function of a signal generator?
- 【DIY Creative LED】LED lights and holes
- Surge arrester explanation and working principle
- Is there any error in the schematic diagram of the electrostatic generator?