1. Use proteus to draw a simple circuit diagram for subsequent simulation
2. Programming
/********************************************************************************************************************
---- @Project: Pointer
---- @File: main.c
---- @Edit: ZHQ
---- @Version: V1.0
---- @CreationTime: 20200809
---- @ModifiedTime: 20200809
---- @Description:
---- The baud rate is: 9600.
---- Communication protocol: EB 00 55 XX
---- Call the corresponding array data of the lower computer through the upper computer.
---- Send EB 00 55 XX command to the MCU through the computer serial port debugging assistant, where EB 00 55 is the data header, and the value range of XX is 0x01 to 0x05. Each different value represents calling different array data of the lower computer. 0x01 calls the first group of data, 0x02 calls the second group of data, and 0x05 calls the fifth group of data.
---- Group 1: 11 12 13 14 15
---- Group 2: 21 22 23 24 25
---- Group 3: 31 32 33 34 35
---- Group 4: 41 42 43 44 45
---- Group 5: 51 52 53 54 55
----
---- The lower computer returns 21 data. The first 5 are the data returned by the first function without a pointer. The middle 5 are the data returned by the second function without a pointer. The last 5 are the data returned by the third function with a pointer. The two groups of EE EE EE in between are the dividing lines of the data returned by each function. They are for the convenience of observation and have no practical significance.
---- For example, computer sends: EB 00 55 02
---- The MCU returns: 21 22 23 24 25 EE EE EE 21 22 23 24 25 EE EE EE 21 22 23 24 25
---- Microcontroller: AT89C52
********************************************************************************************************************/
#include "reg52.h"
/*——————Macro definition——————*/
#define FOSC 11059200L
#define BAUD 9600
#define T1MS (65536-FOSC/12/500) /*0.5ms timer calculation method in 12Tmode*/
#define const_array_size 5 /* The size of the array involved in sorting*/
#define const_voice_short 19 /*Duration of the buzzer short call*/
#define const_rc_size 10 /*Buffer array size for receiving serial port interrupt data*/
#define const_receive_time 5 /*If no serial data comes in after this time, it is considered that a string of data has been received. This time can be adjusted according to the actual situation*/
/*——————Variable function definition and declaration——————*/
/*Buzzer driver IO port*/
sbit BEEP = P2^7;
/*LED*/
sbit LED = P3^5;
unsigned int uiSendCnt = 0; /*Timer used to identify whether the serial port has received a string of data*/
unsigned char ucSendLock = 1; /*Self-locking variable of the serial port service program, each time a string of data is received, it is only processed once*/
unsigned int uiRcregTotal = 0; /*Indicates how much data the current buffer has received*/
unsigned char ucRcregBuf[const_rc_size]; /*buffer array for receiving serial port interrupt data*/
unsigned int uiRcMoveIndex = 0; /*Intermediate variable used to parse data protocol*/
unsigned int uiVoiceCnt = 0; /*Buzzer beeping duration counter*/
const unsigned char array_0x01[] = {0x11, 0x12, 0x13, 0x14, 0x15};
const unsigned char array_0x02[] = {0x21, 0x22, 0x23, 0x24, 0x25};
const unsigned char array_0x03[] = {0x31, 0x32, 0x33, 0x34, 0x35};
const unsigned char array_0x04[] = {0x41, 0x42, 0x43, 0x44, 0x45};
const unsigned char array_0x05[] = {0x51, 0x52, 0x53, 0x54, 0x55};
/**
* @brief Timer 0 initialization function
* @param None
* @retval initialize T0
**/
void Init_T0(void)
{
TMOD = 0x01; /*set timer0 as mode1 (16-bit)*/
TL0 = T1MS; /*initial timer0 low byte*/
TH0 = T1MS >> 8; /*initial timer0 high byte*/
}
/**
* @brief Serial port initialization function
* @param None
* @retval initialize T0
**/
void Init_USART(void)
{
SCON = 0x50;
TMOD = 0x21;
TH1=TL1=-(FOSC/12/32/BAUD);
}
/**
* @brief peripheral initialization function
* @param None
* @retval Initialize peripheral
* Transfer the contents displayed by the digital tube to the following variable interfaces to facilitate the writing of higher-level window programs in the future.
* Simply change the content of the corresponding variables below to display the numbers you want.
**/
void Init_Peripheral(void)
{
ET0 = 1;/*Enable timer interrupt*/
TR0 = 1;/*Start timing interrupt*/
TR1 = 1;
ES = 1; /*Enable serial port interrupt*/
EA = 1;/*Open the general interrupt*/
}
/**
* @brief initialization function
* @param None
* @retval initialize the MCU
**/
void Init(void)
{
LED = 0;
Heat_T0();
init_USART();
}
/**
* @brief delay function
* @param None
* @retval None
**/
void Delay_Long(unsigned int uiDelayLong)
{
unsigned int i;
unsigned int j;
for(i=0;i for(j=0;j<500;j++) /*Number of empty instructions in the embedded loop*/ { ; /*A semicolon is equivalent to executing an empty statement*/ } } } /** * @brief delay function * @param None * @retval None **/ void Delay_Short(unsigned int uiDelayShort) { unsigned int i; for(i=0;i ; /*A semicolon is equivalent to executing an empty statement*/ } } /** * @brief Serial port sending function * @param unsigned char ucSendData * @retval Function that sends a byte to the host computer **/ void eusart_send(unsigned char ucSendData) { ES = 0; /* Disable serial port interrupt */ TI = 0; /* Clear the serial port transmission completion interrupt request flag */ SBUF = ucSendData; /* Send one byte */ Delay_Short(400); /* The delay between each byte is very critical and is also the most prone to error. Please adjust the delay size according to the actual project*/ TI = 0; /* Clear the serial port transmission completion interrupt request flag */ ES = 1; /* Enable serial port interrupt */ } /** * @brief The first function * @param ucArraySec * @retval * The first function has no internal pointer and follows the instructions of the host computer. * Directly returns the corresponding array. Since there is no pointer, 5 more for loops are used to move the array. * It consumes more program ROM space and is not concise and clear enough. **/ void send_array_1(unsigned char ucArraySec) { unsigned char i; switch (ucArraySec) { case 1: /* Directly return the first constant array*/ for(i = 0; i < 5; i ++) { eusart_send(array_0x01[i]); } break; case 2: /* Directly return the second constant array*/ for(i = 0; i < 5; i ++) { eusart_send(array_0x02[i]); } break; case 3: /* Directly return the third constant array*/ for(i = 0; i < 5; i ++) { eusart_send(array_0x03[i]); } break; case 4: /* Directly return the 4th constant array*/ for(i = 0; i < 5; i ++) { eusart_send(array_0x04[i]); } break; case 5: /* Directly return the 5th constant array*/ for(i = 0; i < 5; i ++) { eusart_send(array_0x05[i]); } break; } } /** * @brief The second function * @param ucArraySec * @retval * The second function has no internal pointer and follows the instructions of the host computer. * First transfer the corresponding array to an intermediate variable array, and then send the array. * Since there is no pointer, 6 more for loops are used to move the array. * Like the first function, it consumes more program ROM space and is not concise and clear enough. **/ void send_array_2(unsigned char ucArraySec) { unsigned char i; unsigned char array_temp[5]; /* Temporary intermediate array*/ switch (ucArraySec) { case 1: /* Directly return the first constant array*/ for(i = 0; i < 5; i ++) { array_temp[i] = array_0x01[i]; /* First transfer the corresponding array data to the intermediate array one by one*/ } break; case 2: /* Directly return the second constant array*/ for(i = 0; i < 5; i ++) { array_temp[i] = array_0x02[i]; /* First transfer the corresponding array data to the intermediate array one by one*/ } break; case 3: /* Directly return the third constant array*/ for(i = 0; i < 5; i ++) { array_temp[i] = array_0x03[i]; /* First transfer the corresponding array data to the intermediate array one by one*/ } break; case 4: /* Directly return the 4th constant array*/ for(i = 0; i < 5; i ++) { array_temp[i] = array_0x04[i]; /* First transfer the corresponding array data to the intermediate array one by one*/ } break; case 5: /* Directly return the 5th constant array*/ for(i = 0; i < 5; i ++) { array_temp[i] = array_0x05[i]; /* First transfer the corresponding array data to the intermediate array one by one*/ } break; } for(i = 0; i < 5; i ++) { eusart_send(array_temp[i]); /* Send all the data temporarily stored in the intermediate array*/ } } /** * @brief The third function * @param ucArraySec * @retval * The third function has a pointer inside, and according to the relevant instructions of the host computer, * First pass the corresponding array first address to an intermediate pointer, and then pass * The pointer sends the data of the entire array. Because of the pointer, switching and transferring the data of the array is very fast. * Just pass the first address to the pointer, which is very efficient. The whole function only uses one for loop. * Compared with the first and second functions, it saves more program capacity, has faster processing speed and is more concise. **/ void send_array_3(unsigned char ucArraySec) { unsigned char i; unsigned char *p_array; /* Temporary intermediate pointer, as a transfer station for the array, very efficient*/
Previous article:Use interrupts and mutexes to protect global variables shared by multiple threads
Next article:Add const to pointers to avoid accidental modification of input-only data
Recommended ReadingLatest update time:2024-11-15 08:32
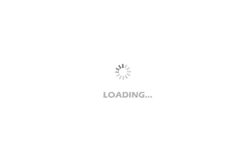
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- CGD and Qorvo to jointly revolutionize motor control solutions
- CGD and Qorvo to jointly revolutionize motor control solutions
- Keysight Technologies FieldFox handheld analyzer with VDI spread spectrum module to achieve millimeter wave analysis function
- Infineon's PASCO2V15 XENSIV PAS CO2 5V Sensor Now Available at Mouser for Accurate CO2 Level Measurement
- Advanced gameplay, Harting takes your PCB board connection to a new level!
- Advanced gameplay, Harting takes your PCB board connection to a new level!
- A new chapter in Great Wall Motors R&D: solid-state battery technology leads the future
- Naxin Micro provides full-scenario GaN driver IC solutions
- Interpreting Huawei’s new solid-state battery patent, will it challenge CATL in 2030?
- Are pure electric/plug-in hybrid vehicles going crazy? A Chinese company has launched the world's first -40℃ dischargeable hybrid battery that is not afraid of cold
- BLE over-the-air upgrade
- go go go, make the LED of RSL10 start blinking (there is a little easter egg at the end of the article)
- CH224D Review: USB PD Portable Soldering Iron is Here
- Bluetooth, 4-Digit True RMS Digital Multimeter
- Zibee transparent transmission program development
- June 14th prize live broadcast | TI takes you to experience the interconnected and efficient smart home solutions
- World clock controlled by NXP LPC845
- EEWORLD University Hall----Live Replay: TI's low-power wireless technology for building automation sensor applications
- What is the difference between TVCC and VCC in ST-LINK V2, with pictures
- Download the NI Practical Guide to Maximizing DC Measurement Performance