1. MSCAN receiving process
Detect receive flag: The CANRFLG register is used here.
Detect frame mode (standard frame/extended frame): The CANIDR register is used here.
Read identifier: The CANIDR register is used here.
Determine the frame format (remote frame/data frame): The CANIDR register is used here.
Read data length: The CANDLR register is used here.
Read data: The CANDSR register is used here.
Clear the receive flag: The CANTFLG register is used here.
2. Introduction to MSCAN Receive Register
There are two types of CANIDR registers: standard frame type and extended frame type.
Standard frame type:
Extended frame type:
3. CAN receiving configuration specific program implementation
1. Standard frame program
The specific code is as follows:
struct can_msg //Message structure
{
unsigned int id; //帧ID
unsigned char RTR; // RTR: 1 for remote frame, 0 for data frame
unsigned char data[8]; //frame data
unsigned char len; //data length
unsigned char prty; //priority
};
/*************************************************************/
/* CAN0 receiving */
/*************************************************************/
int MSCAN0_Receive(struct can_msg *msg)
{
unsigned char sp2;
// Check the reception flag
if(!(CAN0RFLG_RXF))
return(FALSE);
// Detect CAN protocol message mode (general/extended) identifier
if(CAN0RXIDR1_IDE) //0 is standard format, 1 is extended format
// IDE = Recessive (Extended Mode)
return(FALSE);
// Read the identifier
msg.id = (unsigned int)(CAN0RXIDR0<<3) |
(unsigned char)(CAN0RXIDR1>>5);
if(CAN0RXIDR1&0x10)
msg.RTR = TRUE;
else
msg.RTR = FALSE;
// Read data length
msg.len = CAN0RXDLR;
// Read data
for(sp2 = 0; sp2 < msg.len; sp2++)
msg.data[sp2] = *((&CAN0RXDSR0)+sp2);
// Clear RXF flag (buffer is ready to receive)
CAN0RFLG = 0x01;
return(TRUE);
}
2. Extended frame program
The specific code is as follows:
struct can_msg //Message structure
{
unsigned long ID; //帧ID
unsigned char RTR; // RTR: 1 for remote frame, 0 for data frame
unsigned char data[8]; //frame data
unsigned char len; //data length
unsigned char prty; //priority
};
/*************************************************************/
/* CAN0 receiving */
/*************************************************************/
int MSCAN0_Receive(struct can_msg *msg)
{
unsigned char sp2;
// Check the reception flag
if(!(CAN0RFLG_RXF))
return(FALSE);
// Detect CAN protocol message mode (general/extended) identifier
if(!CAN0RXIDR1_IDE) //0 is standard format, 1 is extended format
// IDE = Recessive (Extended Mode)
return(FALSE);
// Read the identifier
msg.ID = ((unsigned long)(CAN1RXIDR0&0xff))<<21;
msg.ID = can1_msg_get.ID & ((unsigned long)0xff<<21);
msg.ID = can1_msg_get.ID | (((unsigned long)(CAN1RXIDR1&0xe0))<<13);
msg.ID = can1_msg_get.ID | (((unsigned long)(CAN1RXIDR1&0x07))<<15);
msg.ID = can1_msg_get.ID | (((unsigned long)(CAN1RXIDR2&0xff))<<7);
msg.ID = can1_msg_get.ID | (((unsigned long)(CAN1RXIDR3&0xfe))>>1);
if(CAN0RXIDR3&0x01)
msg.RTR = TRUE;
else
msg.RTR = FALSE;
// Read data length
msg.len = CAN0RXDLR;
// Read data
for(sp2 = 0; sp2 < msg.len; sp2++)
msg.data[sp2] = *((&CAN0RXDSR0)+sp2);
// Clear RXF flag (buffer is ready to receive)
CAN0RFLG = 0x01;
return(TRUE);
}
Previous article:Freescale MC9S12X SCI driver
Next article:Freescale MC9S12X: CAN initialization configuration
Recommended ReadingLatest update time:2024-11-15 07:28
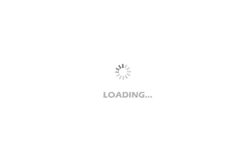
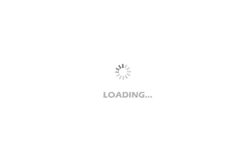
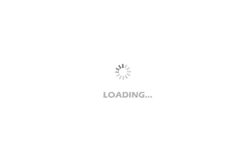
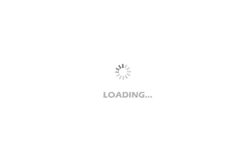
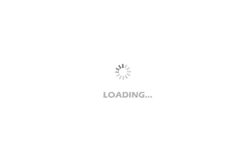
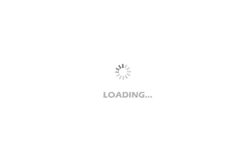
- Popular Resources
- Popular amplifiers
-
Detailed explanation of big data technology system: principles, architecture and practice (Dong Xicheng)
-
Automotive CAN embedded intrusion detection system based on deep learning
-
Lightweight FPGA-based IDS-ECU architecture for automotive CAN networks
-
A review of deep learning applications in traffic safety analysis
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- CGD and Qorvo to jointly revolutionize motor control solutions
- CGD and Qorvo to jointly revolutionize motor control solutions
- Keysight Technologies FieldFox handheld analyzer with VDI spread spectrum module to achieve millimeter wave analysis function
- Infineon's PASCO2V15 XENSIV PAS CO2 5V Sensor Now Available at Mouser for Accurate CO2 Level Measurement
- Advanced gameplay, Harting takes your PCB board connection to a new level!
- Advanced gameplay, Harting takes your PCB board connection to a new level!
- A new chapter in Great Wall Motors R&D: solid-state battery technology leads the future
- Naxin Micro provides full-scenario GaN driver IC solutions
- Interpreting Huawei’s new solid-state battery patent, will it challenge CATL in 2030?
- Are pure electric/plug-in hybrid vehicles going crazy? A Chinese company has launched the world's first -40℃ dischargeable hybrid battery that is not afraid of cold
- CC2640 debugging experience: adding serial port
- 【NXP Rapid IoT Review】+ GUI Usage
- N32WB452 asked: Does N32WB452 have a basically complete ported RT-Thread development kit?
- IoT Gateways – A Simple Guide to the Internet of Things
- [Anxinke UWB indoor positioning module NodeMCU-BU01] No.004-Distance measurement test, evaluation completed
- [Bluesun AB32VG1 RISC-V evaluation board] IDE installation and use
- It's New Year's Eve soon. I wish you all a happy New Year.
- 【DIY Creative LED】Add touch button and install it on LED lamp
- [RISC-V MCU CH32V103 Review] - 2: Making an LED flash is not easy
- SensorTile.box uses air pressure sensors to analyze environmental changes