Introduction: In this experiment, after pressing a key, the relevant signal is transmitted to 74HC165 in parallel, and then transmitted to the processor in serial mode. The processor controls the buzzer by controlling the P0.22 port (MAT0.0).
In this experiment, after pressing a key, the relevant signal is transmitted to 74HC165 in parallel, and then transmitted to the processor in serial mode. The processor controls the buzzer by controlling the P0.22 port (MAT0.0).
#include //Macro definition #define SCLK 0x01<<24 #define SCK0 0x01<<4 #define MISO 0x01<<5 #define MOSI 0x01<<6 #define RCK 0x01<<7 void HC595_Init(void); void WriteByte(unsigned char data); void Write595(void); void HC165_CS(char flag); unsigned char Read165(void); void Delayn(unsigned long n); //595 extended interface data display cache unsigned int HC595_DATA = 0xFFFFFFFF; //Initialize 595 interface void HC595_Init(void) { IO0DIR |= MOSI|RCK|SCK0; IO1DIR |= SCLK; IO1CLR |= SCLK; IO0DIR |= RCK; HC595_DATA = 0xFFFFFFFF; Write595(); } //Write bytes void WriteByte(unsigned char data) { unsigned char i; //IO0CLR = RCK; for(i=0;i<8;i++) { if(data&0x01) IO0SET=MOSI; else IO0CLR=MOSI; IO1SET=SCLK; data>>=1; IO1CLR=SCLK; } //IO0SET = RCK; } //Refresh 595 data void Write595(void) { IO0CLR = RCK; WriteByte(HC595_DATA&0xff); WriteByte((HC595_DATA&0xff00)>>8); WriteByte((HC595_DATA&0xff0000)>>16); WriteByte((HC595_DATA&0xff000000)>>24); IO0SET = RCK; } //165 chip select void HC165_CS(char flag) { if(flag) ////CLK INH writes high level, HC595_DATA &= ~(1<<1); else HC595_DATA |= (1<<1); Write595(); } //Read the data of 165 unsigned char Read165(void) { unsigned char RD=0,i; HC165_CS(1); // IO1CLR_bit.P1_25 = 1; //Write 1 to make the corresponding pin output low level, even if S/L (pin 1) gets low level, before the arm board receives data, the pin must be pulled low, the purpose is to load the data on the parallel data A--H into the internal register, which reflects the LOAD function Delayn(10); IO1SET_bit.P1_25 = 1; //Write 1 to make the corresponding pin output high level. Then, in the process of receiving data, the pin must be pulled high to enable the data in the register to be moved, so as to move the data from QH or QN' into the microcontroller. This reflects the SHIFT function. for(i=0;i<8;i++) { RD <<= 1; //Shift left one bit if(IO0PIN&MISO) //MISO0 is the serial data output by 74HC165. Check whether 74HC165 receives the data. If MISO0 is not 0, it means that the data is received. RD |= 1; //Accept high position first IO0SET=SCK0; //The clock is high, and the next data is shifted out on the rising edge (CE is allowed to change from low to high only when CP is high) Delayn(10); IO0CLR=SCK0; //Clock low level (We only need to pull down or raise a port of the arm processor, so that the data can be received step by step. Please remember that the rising edge is valid.) } HC165_CS(0); return RD; } main() { PINSEL0=0x000000; PINSEL1=0x000000; //All pins are connected to GPIO unsigned char HC165_DATA; IO1DIR_bit.P1_25 = 1; IO1SET_bit.P1_25 = 1; PINSEL0_bit.P0_5 = 0; //IO0DIR_bit.P0_5 = 0; //HC165 input pin IO0DIR=1<<22; //Buzzer control port is set to output, the rest are input HC595_Init(); while(1) { HC165_DATA = Read165(); if((HC165_DATA&(1<<4))==0) IO0SET=1<<22; //When KEY2 is pressed, the buzzer sounds (see below for explanation) else IO0CLR=1<<22; //Release KEY2, the buzzer stops beeping } } //Delay cycle number void Delayn(unsigned long n) { while(n--); } Final Notes: Explanation of if((HC165_DATA&(1<<4))==0) IO0SET=1<<22 in the main function, 1<<4 makes the 4th bit become 1, there is a functional diagram in the 74HC165 data sheet: The data transmission method of 74HC165 in the program needs to be studied. The reason why 74HC595 is used is that the board of Litian Electronics connects the chip select (165 CS) of 74HC165 to 595 during design, so 595 must be operated accordingly. It can be seen that the peripheral pin corresponding to the 4th bit is 3. From the connection diagram of the 74HC165 in the first figure above, it can be found that it is connected to KEY INT2, so the corresponding key is KEY2.
Previous article:Implementation of Bootloader in Embedded System
Next article:ARM architecture/features (processors)
Recommended ReadingLatest update time:2024-11-15 19:57
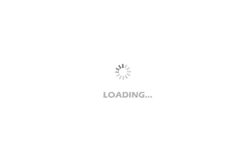
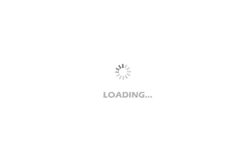
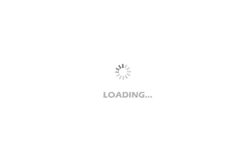
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Today at 10:00 AM R&S Live Broadcast with Prizes [USB 3.2 Compliance Test]
- How to measure code execution time on ARM Cortex-M MCUs?
- 2019 Altium "Mobile Weekend Lab Class" Free Tickets- Shanghai Station
- EEWORLD University - Common techniques for designing low-power DC-DC converters
- Let's talk about five cents: Today's manager is standing at work
- 【DFRobot wireless communication module】Hardware analysis
- Teach you how to design an accurate and thermally efficient wearable body temperature detection system?
- EtherCAT Interface Reference Design for High-Performance MCUs
- Popular Science: Why is the PPS charger not compatible with laptop batteries?
- US NB frequency band