Schematic
Universal Design
When working with PIC and AVR devices, you usually need to get the datasheet for that specific device and understand how to use the peripherals. Sometimes, the peripherals on one PIC may be different than on another PIC, so you can't simply copy and paste the code from one PIC to another. However, the STM8 is completely different because all STM8 devices use a common layout instead of having a unique configuration. This means that code designed for one STM8 can be directly copied and pasted to a different controller and it will still work (assuming the new device has the desired peripherals).
A typical example is the UART peripheral. An STM8 device can have up to three UART ports (1, 2, and 3), and UART1 on one STM8 device is the same as UART1 on another STM8 device. However, the datasheets for the individual STM8 devices do not contain much information on how to use the peripheral, so when using any STM8 device you need to use the datasheets; a device-specific datasheet containing the pinout, and another datasheet containing device family details.
For our STM8 project we will make use of information from these two datasheets:
STM8S103F3 device datasheet (PDF) - contains basic details and pinout information
STM8 Series Device Overview (PDF) - contains detailed peripheral and CPU information
If you want to know where the pins are on a device, use the device datasheet, if you want to learn how to use a peripheral, use the family device overview sheet.
GPIO
While the development board allows us to program the STM8 microcontroller and CPU functions, it is meaningless unless we can connect the micro to other devices and external circuits. To be able to perform such tasks, General Purpose Input Outputs or GPIOs are used. GPIOs are pins on a device that can be electrically connected to external circuits to control them or read information from them. While it is possible to read analog data, this tutorial will focus only on digital values (digital values that are turned on or off).
When it comes to GPIO, there are four main registers:
DDR - Data Direction Register
ODR - Output Data Register
IDR - Input Data Register
CR1 and CR2 – Control Registers
Image courtesy of the RM0016 reference manual.
Accessing registers and bits
Accessing GPIO on the STM8 is somewhat similar to AVR, except that STM8S.h uses structures. For example, PORT B on the STM8S has its own structure called GPIOB, and inside are all the registers that control it (like DDR, ODR, IDR, etc.). Accessing these registers can be done like this:
GPIOB→xxx where xxx is the register in question
Data Direction Register (DDR)
When configuring a pin as an input, the corresponding DDR bit needs to be cleared (0), and for an output, the bit needs to be set (1). So, let's say we only want to configure B0 and B1 as inputs while keeping the rest as outputs. We can do the following:
GPIOB→DDR = 0xFC;
or
GPIOB→DDR = 0b11111100;
Control registers CR1 and CR2
CR1 and CR2 are control registers that can be configured to provide different I/O functions. For example, they can be configured to allow interrupts on individual pins and can be used to create output drivers with push/pull capabilities. As with the other registers, each bit in the CR1 and CR2 registers corresponds to a specific pin. So, for example, bit 0 in CR1 and CR2 is used for pin 0 of the port. The following table (taken from the datasheet) demonstrates the purpose of the CR1 and CR2 registers.
Output Data Register (ODR)
The Output Data Register is used to output digital values (1s and 0s) to a port. Individual bits can be written (using a bit mask), or the entire register can be changed. Writing a 1 to an ODR bit will turn the corresponding pin on, and writing a 0 will turn the corresponding pin off. The first example below turns all pins on a port on, and the second example turns all pins off.
GPIOB→ODR = 0xFF; or GPIOB→ODR = 0b11111111; // turn on all pins
GPIOB→ODR = 0x00; or GPIOB→ODR = 0b00000000; // turn off all pins
Input Data Register (IDR)
The IDR register can be used to read the digital values on the port pins. These values can be either on (1) or off (0), with bit 0 of the IDR register corresponding to pin 0, while bit 7 corresponds to pin 7.
pinRead = GPIOB→IDR;
Useful bit operations
Because I/O ports consist of individual pins, it is more helpful to access individual bits rather than entire registers. However, individual bits are not available (similar to AVR devices), so we need to use some bit manipulation. Since this has already been explained in the AVR series, we will only look at some very useful macros.
These very useful macros help get rid of unreadable bitmasks:
#define setBit(reg, bit)(reg = reg | (1 <
#define clearBit(reg, bit)(reg = reg & ~(1 <
#define toggleBit(reg, bit)(reg = reg ^(1 <
Copy and paste this code at the top of your code, and then, you can use them like functions without having to write bit manipulation code. So let's look at some examples of how to use them in your code!
setBit(GPIOB→DDR,3); //Set bit 3 on port B to output
clearBit(GPIOA→ODR,4); //turn off output bit 4 on port a
toggleBit(GPIOC→ODR), 5); // toggle bit 5 on port c
However, reading the pins uses a simple bitwise operation involving using AND to mask out all the bits we don't need, then testing to see if the result is 0.
if (GPIOB→IDR & 0b00000001) )
{
// Code here executes IF bit 0 is on
}
if (!(GPIOB→IDR & 0b00000001))
{
// Code here executes IF bit 0 is off
}
Basic Configuration Example
In this example, we have configured pin A1 as input and B5 as output, and whenever the switch (connected to A1) is pressed, the LED connected to B5 will toggle. Here we are also taking advantage of the internal pull-ups, so our button does not require a pull-up resistor to work (which is done by setting a bit in CR1).
/* MAIN.C file
*
* Copyright (c) 2002-2005 STMicroelectronics
*/
#include "stm8s.h"
#define setBit(reg, bit) (reg = reg | (1 << bit))
#define clearBit(reg, bit) (reg = reg & ~(1 << bit))
#define toggleBit(reg, bit) (reg = reg ^ (1 << bit))
void simpleDelay(void);
main()
{
GPIOA->DDR = 0x00; // Make all pins on PORT A inputs
GPIOA-》CR1 = 0xFF; // Ensure that internal pull up is on
GPIOA-》CR2 = 0x00; // Ensure that interrupts are turned off
GPIOB->DDR = 0xFF;
while (1)
{
// Testing bit 1 (bit 0 would be 1)
if (! (GPIOA->IDR & 0x02))
{
toggleBit(GPIOB->ODR, 5);
simpleDelay();
}
}
}
// Simple delay used for debouncing
void simpleDelay(void)
{
unsigned int i, j;
for (i = 0; i < 1000; i++)
{
for (j=0; j < 10; j++)
{
}
}
}
Previous article:About Atomthreads, a real-time operating system that can run on STM8
Next article:Introduction to the five major members of the STM8 series
Recommended ReadingLatest update time:2024-11-22 21:51
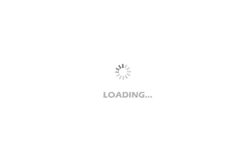
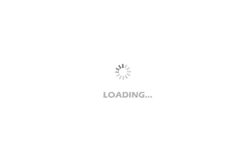
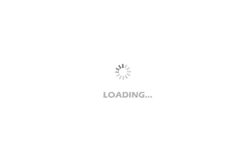
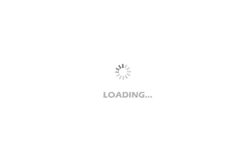
![[STM32 motor square wave] Record 1——GPIO basic configuration](https://6.eewimg.cn/news/statics/images/loading.gif)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- [Raspberry Pi Pico Review] Development Environment Construction and Simple Testing
- [GD32E503 Review] +sdio reads tf card and timeout occurs
- Application Tips/A Software Method to Remove Key Jitter
- Let’s talk about what kind of DuPont wire everyone chooses and the landmines they have stepped on.
- The microcontroller application architecture summarized by experts is recommended for collection
- LIS25BA Bone Vibration Sensor
- Do you think UWB technology will have a bright future?
- Play with EK-TM4C1294XL——by 54chenjq
- Lattice, Altera, Xilinx three-in-one image to ROM, MIF software
- How to design the CC2530 antenna? What is the size or package of the dual-ended antenna?