annotation:
The SWI number is as I wrote it. Under RISC OS, it is the number given to Econet_DoImmediate. Don't take it literally, it's just an example!
You may not have seen LDMFD before, it loads multiple registers from the stack. In this example, we load R0 through R12 and R14 from a fully functional stack. See str.html for more information on register loading and storage.
I said to load R14. So why put it into PC? The reason is that the value stored in R14 at this time contains the return address. We can also use: LDMFD R13!, {R0-R12,R14} MOV PC, R14, but the MOV statement can be omitted by directly restoring to PC.
Finally, these registers may very well be occupied by a SWI call (depending on the code executed during the call), so you'd better push your important registers on the stack and restore them later.
SWI Instructions
SWI soft interrupt
(Software Interrupt)
SWI{condition} <24-bit number>
Instruction Format
This is a simple facility, but probably the most commonly used. Most operating system facilities are provided using SWI. RISC OS without SWI is unthinkable.
Nava Whiteford explains how SWI works (originally in Frobnicate issue 12?)...
What is SWI?
SWI stands for Software Interrupt. SWI is used in RISC OS to access operating system routines or modules produced by third parties. Many applications use modules to provide low-level external access to other applications.
Examples of SWIs are:
File manager SWI, which assists in reading and writing disks, setting properties, etc.
Printer driver SWI is used to assist in using the printing parallel port.
FreeNet/Acorn TCP/IP protocol stack SWI, using TCP/IP protocol to send and receive data on the Internet.
When used in this way, SWI allows the operating system to have a modular structure, which means that the code required to build a complete operating system can be divided into many small parts (modules) and a module handler.
When the SWI handler gets a request for a specific routine number, it finds the location of that routine and executes it, passing any data (as appropriate).
How does it work?
First let's look at how to use it. A SWI instruction (in assembly language) looks like this:
SWI &02
or
SWI "OS_Write0"
These instructions are actually the same and will assemble to the same instructions. The only difference is that the second instruction uses a string to represent the SWI number &02.
When using a program that uses a string number, the string is searched before execution.
We don't want to deal with strings here, because they don't give a true representation of what it is going to do. They are often used to improve the clarity of a program, but are not actual instructions to be executed.
Let's look at the first instruction again:
SWI &02
What does this mean? It literally means entering the SWI handler and passing the value &02. In RISC OS this means executing the routine numbered &02.
How does it do this? How does it pass the SWI number and enter the SWI handler?
If you look at the first 32 bytes of memory (at 0-&1C) and disassemble them (looking at the actual ARM instructions) you will see something like this:
Address Content Disassembly 00000000 : 0..? : E5000030 : STR R0,[R0,#-48] 00000004 : .ó?? : E59FF31C : LDR PC,&00000328 00000008 : .ó?? : E59FF31C : LDR PC,&0000032C 0000000C : .ó?? : E59FF31C : LDR PC,&00000330 00000010 : .ó?? : E59FF31C : LDR PC,&00000334 00000014 : .ó?? : E59FF31C : LDR PC,&00000338 00000018 : .ó?? : E59FF31C : LDR PC,&0000033C 0000001C : 2?? : E3A0A632 : MOV R10,#&3200000
Let’s take a closer look.
With the exception of the first and last instructions (which are special cases) all you see are instructions that load a new value into the PC (Program Counter), which tells the computer where to execute the next instruction.
It also shows that the value is being received from an address in memory. (You can use the "Read Memory" option on the !Zap main menu to see this for yourself.)
This may seem to have little to do with SWI, but is explained further below.
All a SWI does is change the mode to supervisor and set the PC to execute the next instruction at address &08!
Putting the processor into supervisor mode switches out the two registers r13 and r14 and replaces them with r13_svc and r14_svc.
When entering supervisor mode, r14_svc is also set to the address after this SWI instruction.
This is effectively like a branch instruction (BL &08) connected to address &08, but with space for some data (the SWI number).
As I said, address &08 contains an instruction to jump to another address, the address of the actual SWI routine!
At this point you might be thinking "wait a minute! What about the SWI number?". Actually the processor ignores the value itself. The SWI handler uses the value passed in r14_svc to get it.
Here are the steps to do it (after storing registers r0-r12):
It subtracts 4 from r14 to obtain the address of the SWI instruction.
Load this instruction into a register.
Clearing the high-order 8 bits of the instruction removes the OpCode and leaves only the SWI number.
Use this value to find the address of the routine where the code is to be executed (using a lookup table etc).
Restore registers r0-r12.
Takes the processor out of supervisor mode.
Jump to the address of this routine.
Here is an example, from the ARM610 datasheet:
0x08 B Supervisor EntryTable DCD ZeroRtn DCD ReadCRtn DCD WriteIRtn ... Zero EQU 0 ReadC EQU 256 WriteI EQU 512 ; SWI contains the required routine in bits 8-23 and data (if any) in bits 0-7. ; Assume R13_svc points to a suitable stack STMFD R13, {r0-r2 , R14} ; Save working registers and return address. LDR R0,[R14,#-4] ; Get SWI instruction. BIC R0,R0, #0xFF000000 ; Clear the upper 8 bits. MOV R1, R0, LSR #8 ; Get routine offset. ADR R2, EntryTable ; Get the start address of the EntryTable. LDR R15,[R2,R1,LSL #2] ; Branch to correct routine WriteIRtn ; Write character in bits 0 - 7 of R0. ......... LDMFD R13, {r0-r2 , R15}^ ; Restore workspace and return, restoring processor mode and flags.
These are the basic processing steps of the SWI instruction.
Previous article:Overall Architecture Design of Embedded Firewall Based on ARM Processor
Next article:Brief Analysis of Intelligent Lighting Control System Based on ARM
Recommended ReadingLatest update time:2024-11-17 10:28
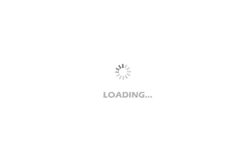
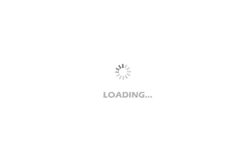
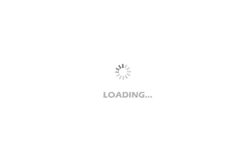
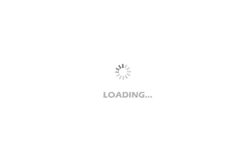
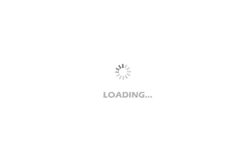
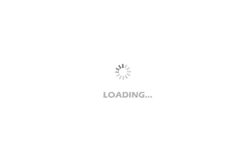
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- New breakthrough! Ultra-fast memory accelerates Intel Xeon 6-core processors
- New breakthrough! Ultra-fast memory accelerates Intel Xeon 6-core processors
- Consolidating vRAN sites onto a single server helps operators reduce total cost of ownership
- Consolidating vRAN sites onto a single server helps operators reduce total cost of ownership
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Use GD32 to make a music spectrum to practice
- Zhou Ligong ZDS1104 and Macosin Oscilloscope STO1104C Operation Video (Transferred)
- Sharing on the design rules of switching power supply layout
- Energy Harvesting with MSP430 FRAM Microcontrollers
- Live FAQ|Maxim IO-Link Solution
- How to solve the problem that a pin of the HuaDa MCU always outputs a high-level pulse after power-on reset/hardware reset?
- RF Antenna Specifications Diagram
- SWD cannot be downloaded, what is the reason? ?
- Waveform selection output
- Analog/digital mixed circuit acceleration simulation technology