Interrupts and exceptions
definition
Except for User and System, all working modes in ARM are exception modes. The exceptions here are broad and include the following three situations:
External Interrupt (External Interrupt)
Changing the program execution flow due to reasons external to the CPU is an asynchronous event and can be blocked
Software interrupt (traps)
The processor has software instructions that can predictably change the execution flow of the program being executed to execute a specific program.
Explicit events, unconditional execution
It is a synchronous event and cannot be blocked.
For example, the Trap instruction in the Motorola 68000 series, the SWI in ARM, the INT in Intel 8086
abnormal
Events caused by internal CPU reasons (such as illegal instructions) or external reasons (such as memory access errors)
There is no corresponding processor instruction
When an exception occurs, the processor unconditionally suspends the currently running program to execute a specific handler.
It is a synchronous event and cannot be blocked.
ARM processors handle the above three events in exception mode and respond through exception vectors. Each exception vector of ARM stores an instruction (usually a jump instruction). When an exception occurs, the CPU automatically goes to the specified vector address to read the instruction and execute it.
Exception Vector Table
ARM's exception vector is generally the instruction that completes the program jump
In ARM720T, ARM9 series and later kernels, the exception vector table can be placed in the high address space starting at 0xFFFF0000
details
If the starting address of the exception handler is within the 32MB range, the jump can be completed directly using the branch instruction
If the starting address of the exception handler is a legal ARM instruction immediate value (which can be obtained by rotating a number between 0 and 255 by an even number of bits), the address can be loaded into the PC using a data transfer instruction.
In other cases, the LDR instruction is required to load the PC.
Exception event types caused by ARM
Abnormal response process
When an exception occurs, the processor core will
Copy the contents of CPSR to the SPSR_ Set the relevant bits of the CPSR (change to ARM state, change to exception mode, disable interrupts (if appropriate)) Save the return address in the LR_ Set PC to the exception vector table address To return from an exception, the exception handler needs to Restore CPSR from SPSR_ Set PC to the address pointed to by LR_ The above can only be done in ARM state Reset exception When Reset is triggered, the CPU enters Supervisor mode and disables FIQ and IRQ R14_svc = Unknown value SPSR_svc = CPSR CPSR[4:0] = 0b10011; Enter management mode CPSR[5] = 0; Execute in ARM state CPSR[6] = 1; Disable fast interrupt FIQ CPSR[7] = 1; Disable normal interrupt IRQ Undefined instruction exception There are two situations that trigger this exception. The CPU executes a coprocessor instruction without waiting for a response from any coprocessor. When the CPU executes an undefined instruction Once this exception occurs, the CPU will complete the following actions R14_und = the address of the next instruction of the undefined instruction SPSR_und = CPSR CPSR[4:0] = 0b11011 ; enter undefined mode CPSR[5] = 0; Execute in ARM state CPSR[7] = 1; Disable normal interrupt IRQ Commonly used exception return methods MOVS PC, R14 ; Skip the instruction where the exception occurred SUBS PC, R14, #4 ; Re-execute the instruction where the exception occurred Software interrupt exception Triggered when the SWI instruction is executed, mainly used to enter the OS system call R14_svc = the address of the next instruction after the SWI instruction SPSR_svc = CPSR CPSR[4:0] = 0b10011; Enter management mode CPSR[5] = 0; Execute in ARM state CPSR[7] = 1; Disable normal interrupt IRQ Commonly used exception return methods: MOVS PC, R14 Instruction prefetch exception When a memory read error occurs while reading an instruction, it is marked as aborted. This exception is triggered when the instruction is to be executed. If it is not executed, this exception is not triggered. That is, when the CPU attempts to execute an instruction that has been marked as invalid, this exception is triggered. R14_abt = the address of the instruction next to the abort instruction SPSR_abt = CPSR CPSR[4:0] = 0b10111 ; Enter the abort mode CPSR[5] = 0; Execute in ARM state CPSR[7] = 1; Disable normal interrupt IRQ Commonly used exception return methods: SUBS PC, R14, #4 Data Abort Exception When loading/storing data between the CPU and the storage system, if an error occurs, this exception is triggered. There are two cases: Internal Abort Exception Caused by the CPU core itself, such as an MMU/MPU error, which means that corrective actions need to be taken and the appropriate instructions need to be re-executed External abort exception Caused by the storage system, possibly a hardware error or the memory address does not exist R14_abt = the address of the instruction next to the abort instruction SPSR_abt = CPSR CPSR[4:0] = 0b10111 ; Enter the abort mode CPSR[5] = 0; Execute in ARM state CPSR[7] = 1; Disable normal interrupt IRQ Commonly used exception return methods SUBS PC, R14, #4 ; Skip the instruction where the exception occurred SUBS PC, R14, #8 ; Return to the instruction where the exception occurred IRQ This exception is triggered when an external IRQ input request occurs and the IRQ interrupt response has been enabled. R14_irq = next instruction address SPSR_irq = CPSR CPSR[4:0] = 0b10010; Enter IRQ mode CPSR[5] = 0; Execute in ARM state CPSR[7] = 1; Disable normal interrupt IRQ Commonly used exception return methods: SUBS PC, R14, #4 FIQ This exception is triggered when an external FIQ input request occurs and the FIQ interrupt response has been enabled. Usually used to transfer data quickly R14_fiq = next instruction address SPSR_fiq = CPSR CPSR[4:0] = 0b10001; Enter FIQ mode CPSR[5] = 0; Execute in ARM state CPSR[6] = 1; Disable fast interrupt FIQ CPSR[7] = 1; Disable normal interrupt IRQ Commonly used exception return methods: SUBS PC, R14, #4 Exception return Except for the reset exception, all other exceptions can be processed and returned to the program that was interrupted when the exception occurred to continue execution. Usually a data transfer instruction is used at the end of the exception handler to complete In exception mode, add the suffix 'S' to the instruction and use the PC as the destination register. This will not only update the value of the PC, but also load the contents of the SPSR register into the CPSR. One of the main reasons for correcting the return address is the pipeline of the ARM processor Notes on calculating return addresses Whether the PC value has been updated when an exception occurs Do the interrupted instructions need to be executed after the exception return? LR = PC - 4 Exception Priority Exceptions are assigned priorities, which determine the order in which they are responded to. At the entrance of all exceptions, the IRQ interrupt is masked and can only trigger kernel response (such as interrupt nesting processing) when it is re-enabled. At the entry of FIQ and Reset exceptions, FIQ interrupts are masked Register usage in exception handling The mode switch that accompanies an exception means that the exception handler that is called will access Its own stack pointer (SP_) Its own link register (LR_) Its own Backup Program Status Register (SPSR_) If it is FIQ exception handling, 5 other general status registers (r8_FIQ to r12_FIQ) The other registers are the same as the registers in the original mode On the external interface, the stack pointer (SP_) must be kept 8-byte aligned The exception handler must ensure that the other (corrupted registers) are reloaded before returning to restore the state before the exception occurred. This can be done by pushing the contents of the working (i.e. corrupted) registers onto the stack and popping them before returning. The application's initialization code will perform the necessary register initialization Interrupt handling ARM can respond to two external interrupt request signals, FIQ and IRQ FIQ interrupts have higher priority than IRQ interrupts When multiple interrupts occur, the FIQ is processed first When responding to a FIQ interrupt, mask the IRQ interrupt (and the FIQ interrupt). The IRQ interrupt will not be processed until the FIQ interrupt handler is completed. The following design enables IFQ to respond as quickly as possible The FIQ vector is at the end of the exception vector table, so that the FIQ handler can start directly from the FIQ vector, saving the time of jumping. There are five additional registers in FIQ mode (R8_FIQ to R12_FIQ) that do not need to be saved and restored when entering and exiting FIQ. FIQ interrupts are masked at the entry of the FIQ interrupt handler and need to be re-enabled (there can be multiple FIQ interrupt sources, but nesting of FIQ interrupts should be avoided for best system performance) Most ARM-based systems have more than two interrupt sources, so an interrupt controller (usually memory-mapped addressing) is needed to control how the interrupt signal enters the ARM chip.
Previous article:Interruption of Arm architecture exception handling process
Next article:ARM exception and interrupt handling
Recommended ReadingLatest update time:2024-11-15 07:51
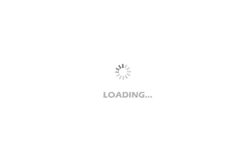
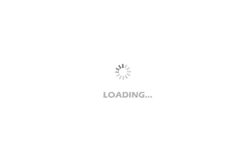
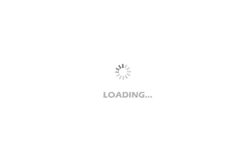
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- CGD and Qorvo to jointly revolutionize motor control solutions
- CGD and Qorvo to jointly revolutionize motor control solutions
- Keysight Technologies FieldFox handheld analyzer with VDI spread spectrum module to achieve millimeter wave analysis function
- Infineon's PASCO2V15 XENSIV PAS CO2 5V Sensor Now Available at Mouser for Accurate CO2 Level Measurement
- Advanced gameplay, Harting takes your PCB board connection to a new level!
- Advanced gameplay, Harting takes your PCB board connection to a new level!
- A new chapter in Great Wall Motors R&D: solid-state battery technology leads the future
- Naxin Micro provides full-scenario GaN driver IC solutions
- Interpreting Huawei’s new solid-state battery patent, will it challenge CATL in 2030?
- Are pure electric/plug-in hybrid vehicles going crazy? A Chinese company has launched the world's first -40℃ dischargeable hybrid battery that is not afraid of cold
- Design of frequency sweeper using digital frequency synthesis technology, FPGA and single chip microcomputer
- Bluetooth MESH technology makes up for the shortcomings of networking
- Some Problems with Differential Circuits
- Analog input
- [Sipeed LicheeRV 86 Panel Review] 10-Video Playback Test
- May Day event is online! Let's do a "labor" transformation of old things together!
- Software - Can I use the Breaking Master on my computer to install Allegro 16.6 and 17.4 at the same time?
- CC3120 Wireless Network Processor BoosterPack Plug-in Module
- Simulink and ModelSim co-simulation to realize BLDC six-step square wave closed-loop control system
- [NUCLEO-L552ZE review] + driving LCD19264 display