STM32F1xx official information:
"STM32 Chinese Reference Manual V10" - Chapter 8 General and Multiplexed Function IO (GPIO and AFIO)
"Cortex-M3 Definitive Guide (Chinese)" Chapter 5 Bit-band Operation
Hardware Hookup
Assume that the hardware connection of the marquee experiment is as shown in the figure above, LED0 is connected to PB5, and LED1 is connected to PE5. Since the other end of the LED is VCC3.3, when PB5 or PE5 is low, the LED will light up. At this time, the GPIO should adopt the push-pull output mode.
GPIO related configuration registers
Each group of GPIO ports of STM32 includes 7 registers. In other words, each register can control 16 GPIO ports of a group of GPIO. These 7 registers are:
GPIOx_CRL: Port Configuration Low Register (32 bits)
GPIOx_CRH: Port Configuration High Register (32 bits)
GPIOx_IDR: Port Input Register (32 bits)
GPIOx_ODR: Port Output Register (32 bits)
GPIOx_BSRR: Port Bit Set/Clear Register (32 bits)
GPIOx_BRR: Port Bit Clear Register (16 bits)
GPIOx_LCKR: Port Configuration Latch Register (32 bits)
Port Configuration Low Register (GPIOx_CRL)
Since each GPIO port requires 4 bits to configure the input and output mode (2 bits for MODE, 2 bits for CNF), each group of 16 GPIO ports requires 64 bits, which means two 32-bit registers are needed. Therefore, GPIOx_CRL is used to configure the input and output mode of GPIO0-GPIO7. Similarly, GPIOx_CRH is used to configure the input and output mode of GPIO8-GPIO15.
At the same time, the above table can summarize the port bit configuration information:
It should be noted that when MODE is selected as 00 and CNF is selected as 10, it represents the pull-up/pull-down input mode. Is it pull-up or pull-down? At this time, PxODR (port output register) is needed to determine, 0 is pull-down input, 1 is pull-up input.
Port Input Register (GPIOx_IDR)
The lower 16 bits of the IDR register control one IO port of the GPIO port group, corresponding to the input level of the IO port. In input mode, the level value of the I/O port can be read; in output mode, the level value of the I/O port can also be read (in open-drain output, the level value of the I/O port read is not necessarily the output level value).
Port Output Register (GPIOx_ODR)
The lower 16 bits of the ODR register, each bit controls an IO port of the GPIO port group, corresponding to the output level of the IO port. In output mode, the level output of a certain IO port can be achieved by writing the value of the register; in input mode, the pull-up or pull-down input mode can also be determined by writing the value.
Port Bit Set/Clear Register (GPIOx_BSRR)
In the open-drain output mode or push-pull output mode of GPIO, you can directly assign values to the ODR register to output the level of a certain IO port; at the same time, you can also assign values to the BSRR to control the ODR register to output the level of the IO port. In fact, the bottom layer of the BSRR register is also the control of the ODR register.
The lower 16 bits of the BSRR register can only set 1 to ODR, and the upper 16 bits can only set 0 to ODR. The advantage of this is that only certain specific bits of ODR can be affected without affecting other bits. In addition, many bits of ODR can be controlled at the same time.
Port Bit Clear Register (GPIOx_BRR)
The function of the BRR register is actually the same as that of the upper 16 bits of the BSRR register. Normally, the lower 16 bits of the BSRR register are used to assign 1, and the BRR register is used to assign 0.
Register version of GPIO
Enable IO port clock. Configuration register RCC_APB2ENR;
Initialize IO port mode. Configure register GPIOx_CRH/CRL;
Operate the IO port to output high or low level. Configure the register GPIOX_ODR or BSRR/BRR.
Specific program content:
void LED_Init(void){
RCC->APB2ENR|=1<<3;
RCC->APB2ENR|=1<<6;
GPIOB->CRL&=0xFF0FFFFF;
GPIOB->CRL|=0x00300000;
GPIOB->ODR|=1<<5;
GPIOE->CRL&=0xFF0FFFFF;
GPIOE->CRL|=0x00300000;
GPIOE->ODR|=1<<5;
}
int main(void)
{
LED_Init();
delay_init();
while(1){
GPIOB->ODR|=1<<5;
GPIOE->ODR|=1<<5;
delay_ms(500);
GPIOB->ODR&=~(1<<5);
GPIOE->ODR&=~(1<<5);
delay_ms(500);
}
}
GPIO library function version
Files to be referenced: stm32f10x_gpio.h, stm32f10x_rcc.h, misc.h
Files to be defined: led.h
Introduction to GPIO library functions
1 initialization function:
void GPIO_Init(GPIO_TypeDef* GPIOx, GPIO_InitTypeDef* GPIO_InitStruct);
Function: Initialize the working mode and speed of one or more IO ports (in the same group). This function mainly operates the GPIO_CRL (CRH) register, and sets the BSRR or BRR register when pulling up or down.
Note: Before using peripherals (including GPIO), you must enable the corresponding clock first.
2 functions to read input level:
uint8_t GPIO_ReadInputDataBit(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin);
uint16_t GPIO_ReadInputData(GPIO_TypeDef* GPIOx);
Function: Read the input level of a certain GPIO (a certain group of GPIOs). The actual operation is on the GPIOx_IDR register.
2 functions to read output level:
uint8_t GPIO_ReadOutputDataBit(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin);
uint16_t GPIO_ReadOutputData(GPIO_TypeDef* GPIOx);
Function: Read the output level of a certain GPIO (a certain group of GPIOs). The actual operation is on the GPIO_ODR register.
4 functions for setting output level:
void GPIO_SetBits(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin);
void GPIO_ResetBits(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin);
void GPIO_WriteBit(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin, BitAction BitVal);
void GPIO_Write(GPIO_TypeDef* GPIOx, uint16_t PortVal);
Function: Set the output of a certain IO port to high level (low level). Actually operate the BSRR register. The functions of the last two functions are similar.
GPIO library function version ticker
Enable the IO port clock. Call the function RCC_APB2PeriphColckCmd();
Initialize the IO port mode. Call the function GPIO_Init();
Operate the IO port to output high and low levels. Call the functions GPIO_SetBits(); GPIO_ResetBits().
Specific program content:
void LED_Init(void){
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB,ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOE,ENABLE);
GPIO_InitStructure.GPIO_Pin=GPIO_Pin_5;
GPIO_InitStructure.GPIO_Mode=GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed=GPIO_Speed_50MHz;
GPIO_Init(GPIOB,&GPIO_InitStructure);
GPIO_SetBits(GPIOB,GPIO_Pin_5);
GPIO_InitStructure.GPIO_Pin=GPIO_Pin_5;
GPIO_InitStructure.GPIO_Mode=GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed=GPIO_Speed_50MHz;
GPIO_Init(GPIOE,&GPIO_InitStructure);
GPIO_SetBits(GPIOE,GPIO_Pin_5);
}
int main(void)
{
LED_Init();
delay_init();
while(1){
GPIO_SetBits(GPIOB,GPIO_Pin_5);
GPIO_SetBits(GPIOE,GPIO_Pin_5);
delay_ms(500);
GPIO_ResetBits(GPIOB,GPIO_Pin_5);
GPIO_ResetBits(GPIOE,GPIO_Pin_5);
delay_ms(500);
}
}
Bit operation version of GPIO
The principle of bit operation
Each bit is expanded into a 32-bit address, and when accessing these addresses, the purpose of accessing the bit is achieved. For example, the BSRR register has 32 bits, so it can be mapped to 32 addresses. We access (read-modify-write) these 32 addresses to achieve the purpose of accessing 32 bits.
In other words, bit operation is to read and write a single bit. Since there is no sbit like 51 microcontroller to implement bit definition in STM32, it can be implemented through the bit band alias area.
Which regions support bit operations:
The lowest 1MB range of the SRAM area, 0x20000000 ‐ 0x200FFFFF (the lowest 1MB in the SRAM area);
The lowest 1MB range of the on-chip peripheral area, 0x40000000 ‐ 0x400FFFFF (the lowest 1MB in the on-chip peripheral area).
Bit-band area: address area that supports bit-band operations
Bitband alias: Access to the alias address ultimately affects the access to the bitband area (note: there is an address mapping process in the middle)
These two 1MB spaces can be operated like ordinary RAM (use read, modify, and write to modify the contents), and they also have their own bit-band alias area, which expands each bit of the 1MB space into a 32-bit word. To be precise, this word is an address, and when operating this address, the purpose of operating a certain bit in this bit-band area can be achieved.
In the bit-band region, each bit is mapped to an address in the alias address region. Note that this is a word with only the LSB being significant (least significant bit). When an alias address is accessed, the address is converted to a bit-band operation.
Mapping method
For a certain bit in the on-chip bit band area, let the address of the byte where it is located be A, and the bit number be n (0<=n<=7), then the address of the bit in the alias area is:
AliasAddr = 0x42000000 + ((A - 0x40000000) * 8 + n) * 4
= 0x42000000 + (A - 0x40000000) * 32 + n * 4
In the above formula, 4 means a word has 4 bytes, and 8 means a byte has 8 bits.
At first, I didn't understand n (0<=n<=7). Since n represents the bit number, why is it not 0<=n<=31? In fact, I ignored the word "byte", that is, in the bit band area, the units are not separated by registers, but by bytes.
For the mapping formula, let me explain it briefly:
A - 0x40000000 = the number of bytes that the current byte is offset from the peripheral base address;
Number of bytes offset * 8 = number of bits offset;
Because each bit in the bit-band area corresponds to an address in the bit-band alias area (32 bits and 4 bytes), and the address is calculated in bytes, so the address of the last offset in the bit-band alias area = the number of bits offset * 4;
n * 4 = The n bits following the offset correspond to the address of the aliased area.
Similarly, for a certain bit in the SRAM bit band area, let its byte address be A and its bit number be n (0<=n<=7), then the address of the bit in the alias area is:
AliasAddr = 0x22000000 + ((A - 0x20000000) * 8 + n) * 4
= 0x22000000 + (A - 0x20000000) * 32 + n * 4
Only the base address of the bit-band and the base address of the bit-band alias area are changed.
For ease of operation, we can combine these two formulas into one formula to convert "bit band address + bit sequence number" into an alias address:
//Convert the bitband address + bit number into a bitband alias macro
#define BITBAND(addr, bit_num) ((addr & 0xf0000000) + 0x02000000 + ((addr & 0x00ffffff) << 5) + (bit_num << 2))
Advantages of bit-band operation
Bit-banding operations can simplify jump determination. For example, if you need to jump to a certain bit before, you must do this:
Read the entire register;
Mask out the bits you don’t need;
Compare and jump.
Now using bit-banding operations only requires:
Read status bits from the bit-band alias area;
Compare and jump.
In the sys.h file in the SYSTEM folder, bit-band operations are implemented for some GPIO input and output functions. The specific implementation process is shown in the following program:
#define BITBAND(addr, bitnum) ((addr & 0xF0000000)+0x2000000+((addr &0xFFFFF)<<5)+(bitnum<<2))
#define MEM_ADDR(addr) *((volatile unsigned long *)(addr))
#define BIT_ADDR(addr, bitnum) MEM_ADDR(BITBAND(addr, bitnum))
//IO port address mapping
#define GPIOA_ODR_Addr (GPIOA_BASE+12) //0x4001080C
#define GPIOA_IDR_Addr (GPIOA_BASE+8) //0x40010808
//IO port operation, only for a single IO port!
// Make sure the value of n is less than 16!
#define PAout(n) BIT_ADDR(GPIOA_ODR_Addr,n) //Output
#define PAin(n) BIT_ADDR(GPIOA_IDR_Addr,n) //Input
The function of assigning a value to the nth bit of the GPIOA_ODR register is realized through PAout(n), and the function of assigning a value to the nth bit of the GPIOA_IDR register is realized through PAin(n). According to this writing method, the main program of the previous library function version can be rewritten as:
int main(void)
{
LED_Init();
delay_init();
while(1){
PBout(5)=1;
PEout(5)=1;
delay_ms(500);
PBout(5)=0;
PEout(5)=0;
delay_ms(500);
}
}
Previous article:【STM32】Basic principles of serial communication (super basic, detailed version)
Next article:【STM32】GPIO working principle (super detailed analysis of eight working modes)
Recommended ReadingLatest update time:2024-11-15 15:10
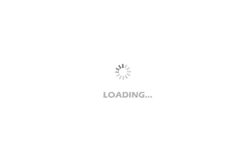
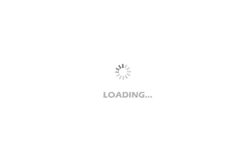
- Popular Resources
- Popular amplifiers
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
- What are the factors that affect the power consumption of microcontrollers?
- GigaDevice GD32 MCU Design Competition Selected Works Album (Limited Time Free Points Download)
- Share the production of a wireless remote control car
- Review of the 2019 National Electronic Design Competition - The small plane I made
- 【GD32F310G-START】USART indefinite length reception
- Discussion on simple polymer lithium battery charging and discharging circuit. Please give me your valuable advice.
- Difference between TMS320C6713 and TMS320C6713B
- [TI recommended course] #DC/DC switching regulator packaging innovation#
- Working principle of LM393 voltage comparator
- The difference between MCU interface and RGB interface