Key interruption experiment, experiment 2 is key query
1. Experimental Principle
1. Use the buttons in query and interrupt mode
For buttons, use query and interrupt modes. Query mode is to put a piece of code in a loop, for example, in the main function, you can query in real time and process it when you find a change, while interrupt mode uses hardware to query the change status and then respond. The difference between the two is that one needs to write the query software code by yourself, and the other is to configure the interrupt mode during initialization, and then directly write your processing code in the interrupt.
2. Implementation steps
① Initialize the IO port as input (in key.c!)
void KEY_Init(void) //IO initialization
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA|RCC_APB2Periph_GPIOE,ENABLE); //Enable PORTA, PORTE clock
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_4|GPIO_Pin_3;//KEY0-KEY1
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU; //Set to pull-up input
GPIO_Init(GPIOE, &GPIO_InitStructure); //Initialize GPIOE4,3
//Initialize WK_UP-->GPIOA.0 pull-down input
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPD; //PA0 is set to input, pull-down by default
GPIO_Init(GPIOA, &GPIO_InitStructure); //Initialize GPIOA.0
}
② Turn on AFIO clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_AFIO,ENABLE);
③Set the mapping relationship between IO port and interrupt line.
void GPIO_EXTILineConfig();
④Initialize online interrupts, set trigger conditions, etc.
EXIT_Init();
⑤Configure the interrupt group NVIC and enable interrupt.
NVIC_Init();
⑥Write interrupt service function.
EXTIx_IRQHandler();
⑦ Clear the interrupt flag
EXTI_ClearITPendingBit();
2. Experimental Code
**exit.c**
#include "exti.h"
#include "led.h"
#include "key.h"
#include "delay.h"
#include "usart.h"
#include "beep.h"
void EXTIX_Init(void)
{
EXTI_InitTypeDef EXTI_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
KEY_Init(); //Key port initialization
RCC_APB2PeriphClockCmd(RCC_APB2Periph_AFIO,ENABLE); //Enable multiplexing function clock
//GPIOE.3 interrupt line and interrupt initialization configuration falling edge trigger //KEY1
GPIO_EXTILineConfig(GPIO_PortSourceGPIOE,GPIO_PinSource3);
EXTI_InitStructure.EXTI_Line=EXTI_Line3;
EXTI_InitStructure.EXTI_Mode = EXTI_Mode_Interrupt;
EXTI_InitStructure.EXTI_Trigger = EXTI_Trigger_Falling;
EXTI_Init(&EXTI_InitStructure); //Initialize the peripheral EXTI registers according to the parameters specified in EXTI_InitStruct
//GPIOE.4 interrupt line and interrupt initialization configuration falling edge trigger //KEY0
GPIO_EXTILineConfig(GPIO_PortSourceGPIOE,GPIO_PinSource4);
EXTI_InitStructure.EXTI_Line=EXTI_Line4;
EXTI_InitStructure.EXTI_Mode = EXTI_Mode_Interrupt;
EXTI_InitStructure.EXTI_Trigger = EXTI_Trigger_Falling;
EXTI_Init(&EXTI_InitStructure); //Initialize the peripheral EXTI registers according to the parameters specified in EXTI_InitStruct
//GPIOA.0 interrupt line and interrupt initialization configuration rising edge trigger PA0 WK_UP
GPIO_EXTILineConfig(GPIO_PortSourceGPIOA,GPIO_PinSource0);
EXTI_InitStructure.EXTI_Line=EXTI_Line0;
EXTI_InitStructure.EXTI_Trigger = EXTI_Trigger_Rising;
EXTI_Init(&EXTI_InitStructure); //Initialize the peripheral EXTI registers according to the parameters specified in EXTI_InitStruct
NVIC_InitStructure.NVIC_IRQChannel = EXTI0_IRQn; // Enable the external interrupt channel where the WK_UP button is located
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0x02; //Preemption priority 2,
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0x03; //Subpriority 3
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //Enable external interrupt channel
NVIC_Init(&NVIC_InitStructure);
NVIC_InitStructure.NVIC_IRQChannel = EXTI3_IRQn; // Enable the external interrupt channel where the key KEY1 is located
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0x02; //Preemption priority 2
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0x01; //Subpriority 1
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //Enable external interrupt channel
NVIC_Init(&NVIC_InitStructure); //Initialize peripheral NVIC registers according to the parameters specified in NVIC_InitStruct
NVIC_InitStructure.NVIC_IRQChannel = EXTI4_IRQn; // Enable the external interrupt channel where the key KEY0 is located
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0x02; //Preemption priority 2
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0x00; //Subpriority 0
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //Enable external interrupt channel
NVIC_Init(&NVIC_InitStructure); //Initialize peripheral NVIC registers according to the parameters specified in NVIC_InitStruct
}
//External interrupt 0 service routine
void EXTI0_IRQHandler(void)
{
delay_ms(10); //debounce
if(WK_UP==1) //WK_UP button
{
BEEP=!BEEP;
}
EXTI_ClearITPendingBit(EXTI_Line0); //Clear the interrupt flag on LINE0
}
//External interrupt 3 service routine
void EXTI3_IRQHandler(void)
{
delay_ms(10); //debounce
if(KEY1==0) //key KEY1
{
LED1=!LED1;
}
EXTI_ClearITPendingBit(EXTI_Line3); //Clear the interrupt flag on LINE3
}
void EXTI4_IRQHandler(void)
{
delay_ms(10); //debounce
if(KEY0==0) //key KEY0
{
LED0=!LED0;
LED1=!LED1;
}
EXTI_ClearITPendingBit(EXTI_Line4); //Clear the interrupt flag on LINE4
}
Previous article:STM32 serial communication uses interrupt mode to send and receive
Next article:STM32 Basic Experiment 3 (Serial Communication)
Recommended ReadingLatest update time:2024-11-23 07:57
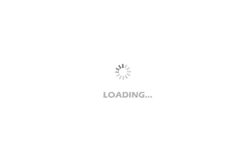
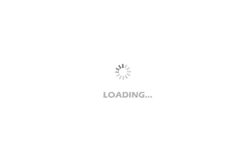
- Popular Resources
- Popular amplifiers
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- A great popular science article on electromagnetic compatibility principles, methods and design!
- What is it like to run high-speed lines on the signal layer of ultra-thick copper?
- ADC conversion method and main parameters
- GaN: Key Technologies for Solving 5G Challenges
- MicroPython driver porting for LIS2DW12 motion sensor
- The 3.3V square wave signal output by the microcontroller can be measured by the multimeter as 1.5V voltage
- Introduction to SPI NAND flash
- Microcomputer Principles and Interface Design
- Inductor Current Measurement in Switching Power Supplies
- [HC32F460 Development Board Review] 07. Hardware I2C to implement EEPROM read and write operations