1. Functions involved
serial,fopen,fclose,
instrfindall,instrhwinfo,
fprintf,fscanf,fwrite,fread,isempty
1.1 Serial port function
scom = serial('com6','BaudRate',115200,'BytesAvailableFcnMode','byte');
fopen(scom);
.
fclose(scom);
Or set the serial port properties separately as follows:
scom = serial(com);
scom.BaudRate = 115200;
scom.InputBufferSize = 512;
scom.BytesAvailableFcnMode = 'terminator'; % 'byte'
scom.terminator = CR/LF ;
scom.Timeout = 50; %read or write wait time
fopen(scom);
.
fclose(scom);
Usually the serial port is deleted after closing it, and the serial port data is cleared in the MATLAB workspace:
delete(scom);
clear scom;
Question 1.: After opening Matlab, the serial port can be opened successfully for the first time, but the following error will be reported when opening it for the second time:
>> scom = serial('com6','BaudRate',115200,'BytesAvailableFcnMode','byte');
>> fopen(scom)
Error using serial/fopen (line 72)
Open failed: Port: COM6 is not
available. Available ports: COM1.
Use INSTRFIND to determine if other
instrument objects are connected to the
requested device.
Solution: My personal understanding is that after closing the serial port, the serial port is not completely cleared, just like some software will fail to be installed again after being uninstalled, so you need to delete all previous settings of the serial port before reopening it, as follows:
>> scom = serial('com6','BaudRate',115200,'BytesAvailableFcnMode','byte');
>> fopen(scom);
Error using serial/fopen (line 72)
Open failed: Port: COM6 is not
available. Available ports: COM1.
Use INSTRFIND to determine if other
instrument objects are connected to the
requested device.
>> delete(instrfindall('Type','serial'));
>> scom = serial('com6','BaudRate',115200,'BytesAvailableFcnMode','byte');
>> fopen(scom);
>> fclose(scom)
>> help instrfindall
instrfindall Find all communication interface objects with specified
property values.
The instrfindall function can find all interfaces that communicate with MATLAB, and can also find interfaces that meet specific parameters.
1.2 Understanding serial parameters
All the parameters and current values of the serial port can be obtained through >> s=get(scom), the main settings of which are:
BaudRate, baud rate
ByteOrder, data big endian or little endian mode, default small segment
DataBits, data bits, usually 8 bits by default
Parity, check bit, default is none
StopBits, stop bit, default 1
Timeout, waiting time for MATLAB serial port to send or read data
ReadAsyncMode, asynchronous reading mode, continuous or manual, the default is continuous
----------
BytesAvailableFcnMode
BytesAvailableFcnCount
BytesAvailableFcn
Terminator
BytesAvailable
The callback function is entered when
Matlab searches for available serial port functions using instrhwinfo:
>> info = instrhwinfo('serial')
info =
HardwareInfo with properties:
AvailableSerialPorts: {2x1 cell}
JarFileVersion: 'Version 3.7'
ObjectConstructorName: {2x1 cell}
SerialPorts: {2x1 cell}
Access to your hardware may be provided by a support package. Go to the Support Package Installer to learn more.
>> info.SerialPorts
years =
'COM1'
'COM6'
>> info.AvailableSerialPorts
years =
'COM1'
'COM6'
>> str = char(info.SerialPorts(2))
str =
COM6
>> scom=serial(str);
I checked the computer device manager and found that the serial port used was 'COM6'. I don't know what 'COM1' is connected to, so the function of using MATLAB to automatically select the serial port has not been realized here.
1.3 Data reading and writing functions
matlab:
fprintf(scom,'%d\n', data,'async' );
data = fscanf(scom,'%d');
c:
scanf("%d",&data);
printf("%d\r\n",data);
Note1: The scanf and printf functions in C print and read data from the terminal by default, so the fputc and fgetc functions need to be redirected here.
Note2: The scanf function in C will continue to run and not exit until it reads valid data.
Question 2: Call the scanf function in the serial port interrupt function of stm32 to read the data sent by matlab. Use fprintf(scom,'%d\r\n', data,'async') in matlab to send data. When stm32 enters the interrupt to read the data, it always enters the interrupt again and enters the scanf function and cannot come out.
Processing method: The format of data sent in matlab is '%d\r\n', which is carriage return plus line feed. My personal understanding is that after the serial port sends a byte, it also sends out '\r', which is a carriage return character. Therefore, after the scanf function in the serial port receive buffer of stm32 reads the data, the carriage return character causes the receive interrupt again.
After changing the data format in the matlab send function to '%d\n', stm32 can read the data normally.
matlab:
fwrite(scom,data,'uint8','async');
cmd_ack = fread(scom,1,'uint8');
c:
rec = USART_ReceiveData( DEBUG_USART );
Usart_SendByte(DEBUG_USART,data);
Note1: fwrite and fread send data in binary format, while fprintf and fscanf above send data in ASCII format.
For example: data is the decimal number 123, its hexadecimal is 0x7b, the underlying binary data stream is 0111 1011, and it is sent as ASCII code as 0x31, 0x32, 0x33, and the underlying data stream is 0011 0001, 0011 0010, 0011 0011.
If you use the fwrite and fread functions in Matlab, the serial port parameters must also be changed to byte.
Previous article:STM32 combination device realizes USB to dual serial port
Next article:STM32 serial communication (buffer-based) programming and problems encountered
Recommended ReadingLatest update time:2024-11-15 11:25
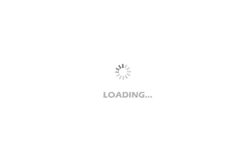
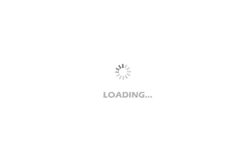
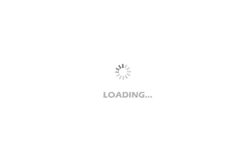
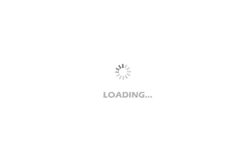
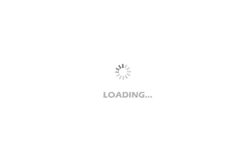
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Melexis launches ultra-low power automotive contactless micro-power switch chip
- Melexis launches ultra-low power automotive contactless micro-power switch chip
- Molex leverages SAP solutions to drive smart supply chain collaboration
- Pickering Launches New Future-Proof PXIe Single-Slot Controller for High-Performance Test and Measurement Applications
- Apple faces class action lawsuit from 40 million UK iCloud users, faces $27.6 billion in claims
- Apple faces class action lawsuit from 40 million UK iCloud users, faces $27.6 billion in claims
- The US asked TSMC to restrict the export of high-end chips, and the Ministry of Commerce responded
- The US asked TSMC to restrict the export of high-end chips, and the Ministry of Commerce responded
- ASML predicts that its revenue in 2030 will exceed 457 billion yuan! Gross profit margin 56-60%
- Detailed explanation of intelligent car body perception system
- Compensation Method for High-Precision Temperature Measurement Using MSP430 Microcontroller
- Machine A sends control command characters
- [Review of Arteli Development Board AT32F421] + Raising a Watchdog
- Let's talk about five cents and share your experience in voltage regulation circuit design
- Share: A brief analysis of the access control system using RFID technology
- MicropyCli - Micropython project management automation
- 【AIoT Smart Smoke Detection System】System Design Overview
- Doping of semiconductors from the perspective of energy levels
- EEWORLD University - HDMI 2.1 Design and Testing Update
- Design of breathing light based on FPGA