Function: Use a common timer to output 2 complementary PWMs with adjustable frequency, adjustable duty cycle and adjustable dead zone.
Principle: As shown in the figure below, the counting mode is the center alignment mode
It can be seen that the CH3 high level interval is centered when the counter counts to 4 and extends to both sides.
As for CH4, since its output polarity is opposite, it extends to both sides with 0 as the center.
CCR3 becomes smaller and the duty cycle increases
CCR4 becomes larger, and the duty cycle increases
The sum of CCR3 and CCR4 is ARR
Note that in this mode the counter count period = ARR, which is different from the PWM mode: count period = ARR + 1
Refer to the following figure in the STM32 reference manual:
Therefore, the code and calculation formula are sorted out as follows:
void TIM3_PWMShiftInit(void)
{
float Duty = 0;
u16 ARR = 0, PSC = 0, CH3Ccr = 0, CH4Ccr = 0, DT = 0;
u32 Frequency = 0;
TIM_TimeBaseInitTypeDef TIM_TimeBaseInitStruct;
GPIO_InitTypeDef GPIO_InitStruct;
TIM_OCInitTypeDef TIM_OCInitStruct;
Frequence = 125000; //Frequency, unit: Hz
Duty = 0.5; //Duty cycle
DT = 1000; //Dead time, unit: ns
//Center alignment mode, only when CNT counts to CCR flips once, the frequency is halved compared to PWM mode, and ARR does not need to be -1
ARR = 36000000/(PSC+1)/Frequence; //Set to 125KHz
CH3Ccr = ARR*Duty - DT/2/((PSC+1)*13.89f); //TIM 72M, one cycle is about 13.89ns
CH4Ccr = ARR - CH3Ccr; //CH4 and CH3 are ARR
/************************TIM3 GPIO configuration************************ *****/
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_0|GPIO_Pin_1;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB,&GPIO_InitStruct);
/**********************Initialize TimBase structure****************************/
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM3,ENABLE);
TIM_TimeBaseInitStruct.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseInitStruct.TIM_CounterMode = TIM_CounterMode_CenterAligned1;
TIM_TimeBaseInitStruct.TIM_Period = ARR;
TIM_TimeBaseInitStruct.TIM_Prescaler = PSC;
TIM_TimeBaseInit(TIM3, &TIM_TimeBaseInitStruct);
TIM_TimeBaseInit(TIM1, &TIM_TimeBaseInitStruct);
/**********************Initialize TIM3 OC structure****************************/
TIM_OCInitStruct.TIM_OCMode = TIM_OCMode_PWM2;
TIM_OCInitStruct.TIM_OCPolarity = TIM_OCPolarity_Low;
TIM_OCInitStruct.TIM_OutputState = TIM_OutputState_Enable;
TIM_OCInitStruct.TIM_Pulse = CH3Ccr;
TIM_OC3Init(TIM3,&TIM_OCInitStruct);
TIM_OCInitStruct.TIM_Pulse = CH4Ccr;
TIM_OCInitStruct.TIM_OCPolarity = TIM_OCPolarity_High;
TIM_OC4Init(TIM3,&TIM_OCInitStruct);
TIM_Cmd(TIM3, ENABLE);
}
The oscilloscope waveform is as follows:
The program sets the frequency to 125K, the duty cycle to 50%, and the dead time to 1000ns. The waveform displayed by the oscilloscope is consistent with this.
Change the frequency to 125K, duty cycle to 25%, dead time to 500ns, and the waveform is as follows:
At 125K, the duration of high level with 25% duty cycle should be 2us, minus the dead zone of 0.5us = 1.5us,
From the figure above, we can see that the high level duration is about 1.5us, which is in line with the program setting.
Previous article:Advanced Timer 1 of STM32F103 with dead zone complementary output configuration
Next article:STM32F107 advanced timer TIM1 uses complementary PWM output
Recommended ReadingLatest update time:2024-11-22 20:30
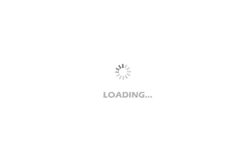
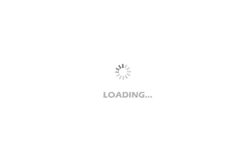
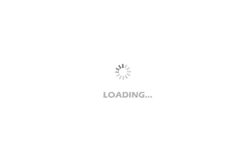
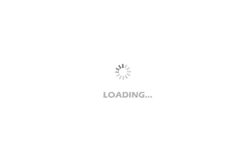
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
-
Intelligent Control Technology of Permanent Magnet Synchronous Motor (Written by Wang Jun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Another technical solution for power-type plug-in hybrid: A brief discussion on Volvo T8 plug-in hybrid technology
- Servo controller
- Design of linear array CCD driving timing circuit based on FPGA
- 【McQueen Trial】Power Supply Modification
- I made a module to exercise my fingers while slacking off, and it generates electricity by tapping
- [NXP Rapid IoT Evaluation] BLE Transmission Sensor Value Test
- 1
- [Hua Diao Experience] 15 Try to build the Arduino development environment of Beetle ESP32 C3
- Fully automatic contactless voltage regulator
- [GD32E231 DIY] DAP RTT, beats all printf formats, swo is no longer a thing
- Live Replay | Microchip Security Solutions Seminar Series (Continuously Updated)