Since I got the stm32, I have always wanted to get started quickly, so I have been browsing various forums and learning from the blogs of various experts. As the saying goes, haste makes waste, and you can't eat hot tofu in a hurry! Is that right?
Finally, I decided to use the library on the st official website. According to the experts, using the library can help you get started quickly. It seems to be true. The tutorials are so well written that you can just use them. But there are so many libraries. Which one should I choose? My God! To be honest, I was tossed around by these libraries! Well, I finally admitted that I used the v3.4 library, which is very convenient!
Let's get down to business and light up the LED.
Hardware: Red Bull development board, STM32F103ZET6 (144 package).
Software: RealView MDK 4.12
stm32 firmware library: v3.4 Attached is the library I organized myself: V3.4_clean.rar
I sorted it out according to the official website library and created a new project template as shown below: (Main reference article "Using STM32 V3.4 library in Keil MDK+ environment.pdf") Using STM32V3.4 library in KeilMDK+ environment.pdf
As shown in the figure: Create a new directory 01_ProLed. It is recommended to put it in the English path to avoid unnecessary trouble. Unzip the above library v3.4 to this directory, and then create a new project directory to store the project.
Description:
CMSIS: The lowest level interface. StartUp: System startup file. StdPeriph_Lib: STM32 peripheral device driver file. Project: Project file. User: User file. New project steps: 300 words are omitted here.
Brief description:
1.core_cm3.c/core_cm3.h
This file is the source file and header file of the kernel access layer. Most of the code is written in assembly language. I don't know much about it online. --Excerpt from "Using STM32 V3.4 Library in Keil MDK+ Environment"
2. stm32f10x.h
is the header file of the peripheral access layer. It is one of the most important header files. Just like reg51.h in 51. For example, it defines what kind of CPU capacity the CPU is, interrupt vectors, etc. In addition to these, this header file also defines structures related to peripheral registers, such as:
typedef struct
{
__IO uint32_t CR;
__IO uint32_t CFGR;
__IO uint32_t CIR;
__IO uint32_t APB2RSTR;
__IO uint32_t APB1RSTR;
__IO uint32_t AHBENR;
__IO uint32_t APB2ENR;
__IO uint32_t APB1ENR;
__IO uint32_t BDCR;
__IO uint32_t CSR;
#ifdef STM32F10X_CL
__IO uint32_t AHBRSTR;
__IO uint32_t CFGR2;
#endif /* STM32F10X_CL */
#if defined (STM32F10X_LD_VL) || defined (STM32F10X_MD_VL) || defined (STM32F10X_HD_VL)
uint32_t RESERVED0;
__IO uint32_t CFGR2;
#endif /* STM32F10X_LD_VL || STM32F10X_MD_VL || STM32F10X_HD_VL */
}RCC_TypeDef;
Contains so many register definitions, so in the application file (such as the main source file you write yourself), you only need to include
stm32f10x.h, instead of the previous firmware library that needs to include the stm32f10x_conf.h header file. --Excerpt from "Using the STM32 V3.4 Library in the Keil MDK+ Environment"
3.system_stm32f10x.c/h
This header file can also be called the header file and source file of the peripheral access layer. In this file, you can define the system clock frequency,
the frequency of the low-speed clock bus and the high-speed clock bus. The most critical function is SystemInit(), which will be introduced in detail later. In short, these
two files are the focus of the new firmware library. With them, the initialization work of using stm32 is greatly simplified. --Excerpt from "Using STM32 V3.4 Library in Keil MDK+ Environment"
4. The contents of the stm32f10x_conf.h
file are the same as those of the V2 version of the library. Uncomment the peripherals that need to be used. For example, if you need to use the GPIO function but not the SPI function, you can do this. --Excerpt from "Using the STM32 V3.4 Library in Keil MDK+ Environment"
#include "stm32f10x_gpio.h"
/*#include "stm32f10x_spi.h"*/
5. There is no need to say more about the main.c
file, you can write it yourself. --Excerpt from "Using STM32 V3.4 Library in Keil MDK+ Environment" 6. The two files
stm32f10x_it.c/h
contain the stm32 interrupt function. Not all interrupt entry functions are written in the source file and header file, but
only a few abnormal interrupts of the ARM core are written. Other interrupt functions need to be written by the user. --Excerpt from "Using STM32 V3.4 Library in Keil MDK+ Environment"
OK, let’s start writing code.
Since the 3.4 library has already set the clock when it starts (which will be described later), we only need to set the corresponding GPIO.
Check the hardware connection:
Come light up PF6.
Create led.c and led.h and add them below User Code.
led.h
#ifndef _LED_H_
#define _LED_H_
void Delay(uint32_t times);
void LedInit(void);
#endif
led.c
#include "stm32f10x.h"
/****************************************************** *************************
* Function name: LedInit(void)
* describe :
* Input: None
* Output: None
* Return: None
*************************************************** ************************/
void LedInit(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
/*Initialize GPIOF's Pin_6 as push-pull output*/
GPIO_InitStructure.GPIO_Pin=GPIO_Pin_6;
GPIO_InitStructure.GPIO_Mode=GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed=GPIO_Speed_50MHz;
GPIO_Init(GPIOF,&GPIO_InitStructure);
}
/****************************************************** *************************
* Function name: Delay(uint32_t times)
* Description: Delay function
* Input: uint32_t times
* Output: None
* Return: None
*************************************************** ************************/
void Delay(uint32_t times)
{
while(times--)
{
uint32_t i;
for (i=0; i<0xffff; i++)
;
}
}
Just add LED initialization and lighting and closing in main.c.
/*!< At this stage the microcontroller clock setting is already configured,
this is done through SystemInit() function which is called from startup
file (startup_stm32f10x_xx.s) before to branch to application main.
To reconfigure the default setting of SystemInit() function, refer to
system_stm32f10x.c file
*/
/* Add your application code here
*/
/*Initialize GPIOF clock*/
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOF,ENABLE);
LedInit();
/* Infinite loop */
while (1)
{
/*Turn off LED1*/
GPIO_SetBits(GPIOF,GPIO_Pin_6);
/*Delay*/
Delay(50);
/*Turn on LED1*/
GPIO_ResetBits(GPIOF,GPIO_Pin_6);
/*Delay*/
Delay(50);
}
Study this code carefully, it is actually very simple, refer to "Using STM32 V3.4 Library in Keil MDK+ Environment". I would like to draw your attention to the previous English comment, what does this English comment mean. "Before running the main function, the system clock has completed the initialization work. Before the main function, the SystemInit function is run by calling the startup code, and this function is located in system_stm32f10x.c". According to the prompts in the text, we go back to system_stm32f10x.c to see how SystemInit initializes the system. The system clock frequency is defined at the beginning of system_stm32f10x.c. From the following code, we can see that the system frequency is
defined as 72MHZ, which is also the frequency of most STM32s when they are running.
#if defined (STM32F10X_LD_VL) || (defined STM32F10X_MD_VL) || (defined STM32F10X_HD_VL)
/* #define SYSCLK_FREQ_HSE HSE_VALUE */
#define SYSCLK_FREQ_24MHz 24000000
#else
/* #define SYSCLK_FREQ_HSE HSE_VALUE */
/* #define SYSCLK_FREQ_24MHz 24000000 */
/* #define SYSCLK_FREQ_36MHz 36000000 */
/* #define SYSCLK_FREQ_48MHz 48000000 */
/* #define SYSCLK_FREQ_56MHz 56000000 */
#define SYSCLK_FREQ_72MHz 72000000
#endif
Then, according to this macro definition, the program tries to initialize the system clock to 72MHz. The code is a bit lengthy, so I won't list it here. In
the SystemInit function, the SetSysClock function is called. If the clock frequency is set to 72MHZ, SetSysCloc calls
the SetSysClockTo72 function. This function is very similar to the RCC_Configuration in each example in the V2 firmware library. It mainly
completes the allocation of the external clock to the system clock after multiplying it by 9. The APB1 clock and APB2 clock are obtained by dividing the system clock. The key code is as follows:
/* HCLK = SYSCLK */
RCC->CFGR |= (uint32_t)RCC_CFGR_HPRE_DIV1;
/* PCLK2 = HCLK */
RCC->CFGR |= (uint32_t)RCC_CFGR_PPRE2_DIV1;
/* PCLK1 = HCLK */
RCC->CFGR |= (uint32_t)RCC_CFGR_PPRE1_DIV2;
From the above analysis, we can see that SystemInit does not need to be called by the user, and the startup code will be executed automatically, which is equivalent to missing most of the content of
the RCC_Configuration function. Please note that it is most of the content, not all of it. However, please pay special
attention to the peripherals used. You should still enable the clock of the peripherals as soon as possible. Don't forget a sentence like this.
/*Initialize GPIOF clock*/
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOF,ENABLE);
-------------------------------------------------- --------------------------------------------------
If not set correctly, compilation may fail:
1. Set up macros. Refer to another article: http://blog.chinaunix.net/space.php?uid=20788517&do=blog&id=296932
2. Set the header file directory: Add all the .h directories. One more thing to note is that you do not need to load header files in the project. The compiler will automatically search and link to the corresponding directory according to the settings.
Pictures speak louder than words:
Source code: ProLed.rar
In addition: Since the official website library is used, you often need to find files or keywords in the project. At this time, you can use the Source Insight tool to assist in reading the code. Its Lookup reference is very useful. You can create another directory Source Insight under the project directory. It is more troublesome, but it is practical!
Previous article:S3c2440 Hardware Part 2: SDRAM
Next article:Research on STM32 clock control RCC
Recommended ReadingLatest update time:2024-11-15 14:34
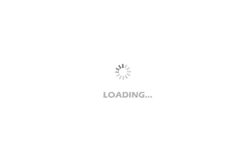
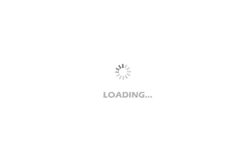
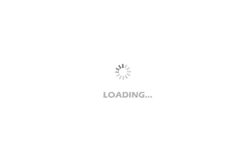
- Popular Resources
- Popular amplifiers
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
- Is it just simple impedance control? Answer with real examples!
- [NXP Rapid IoT Review] Hello Sensor
- What are the advantages of electronic load switches over MOS or transistors as output control? Now many domestic semiconductor...
- Lighter igniter electric shock/ignition principle
- This is the second time I haven't seen any replies in the past few days. The pattern is: there are replies on the 20th floor, but no more on the 21st floor; there are replies on the 40th floor, but no more on the 41st floor...
- Find TI, NXP, ALTERA, XILINX, ST, Microchip factory stock chips
- Record the use of TM1668 driver
- Newbie help, ESP8266 program problem
- GaN Applications in RF and Electronics
- CC2530 Basic Experiment 1 I/O Experiment Code