*************************************************** *************************************************** *****
FileName:dsp_asm.h
**************************************** *************************************************** ***************
*/
#ifndef __DSP_ASM_H__
#define __DSP_ASM_H__
*************************** *************************************************** ****************************
* FUNCTION PROTOTYPES
******************* *************************************************** ************************************
*/
void dsp_asm_test(void);
void dsp_asm_init(void);
#endif /* End of module include. */
/
*
8
...
dsp library in mdk project.
* Use trigonometric functions to generate sampling points for FFT calculation
* When performing FFT test, call the functions in the following order:
* dsp_asm_init();
* dsp_asm_test();
*/
#include "stm32f10x.h"
#include "dsp_asm.h"
#include "stm32_dsp.h"
#include "table_fft.h"
#include
// 1024 point FFt, sampling rate 5120Hz, frequency resolution 5Hz, maximum effective frequency measurement 2560Hz
#ifdef NPT_1024
#define NPT 1024
#define Fs 5120
#endif
/*
*************************************************************************************************************
* LOCAL GLOBAL VARIABLES
*********************************************************************************************************
*/
extern uint16_t TableFFT[];
long lBUFIN[NPT]; /* Complex input vector */
long lBUFOUT[NPT]; /* Complex output vector */
long lBUFMAG[NPT];/* Magnitude vector */
/*
*********************************************************************************************************
* LOCAL FUNCTION PROTOTYPES
*************************************************************************************************************
*/
void dsp_asm_powerMag(void);
/*
*****************************************************************************************************
* Initialize data tables for lBUFIN
* Simulate sampling data. The sampling data contains 3 frequency sine waves: 50Hz, 2500Hz, 2550Hz
* In the lBUFIN array, the real part of the sampling data is stored in the high word (high 16 bits) of each unit data, and the imaginary part of the sampling data (always 0) is stored in the low word (low 16 bits)
**************************************************************************************************
*/
void dsp_asm_init()
{
u16 i=0;
float fx;
for(i=0;i
fx = 4000 * sin(PI2*i*50.0/Fs) + 4000 * sin(PI2*i*2500.0/Fs) + 4000*sin(PI2*i*2550.0/Fs);
lBUFIN[i] = ((s16)fx)<<16;
}
}
/*
******************************************************************************************************
* Test FFT,calculate powermag
* Perform FFT transformation and calculate the amplitude of each harmonic
******************************************************************************************************
*/
void dsp_asm_test()
{
// Select the appropriate FFT function according to the predefined
#ifdef NPT_64
cr4_fft_64_stm32(lBUFOUT, lBUFIN, NPT);
#endif
#ifdef NPT_256
cr4_fft_256_stm32(lBUFOUT, lBUFIN, NPT);
#endif
#ifdef NPT_1024
cr4_fft_1024_stm32(lBUFOUT, lBUFIN, NPT);
#endif
// Calculate the amplitude
dsp_asm_powerMag();
// printf("No. Freq Power\n");
// for(i=0;i
// printf("%4d,%4d,%10d,%1 0d,%10d\n",i,(u16)((float)i*Fs/NPT),lBUFMAG[i],(lBUFOUT[i]>>16),(lBUFOUT[i]&0xffff));
// }
// printf("*********END**********\r\n");
}
/*
**************************************************************************************************
* Calculate powermag
* Calculate the amplitude of each harmonic
* First decompose lBUFOUT into the real part (X) and the imaginary part (Y), then calculate the assignment (sqrt(X*X+Y*Y)
*********************************************************************************************************
*/
void dsp_asm_powerMag(void)
{
s16 lX,lY;
u32 i;
for(i=0;i
lX = (lBUFOUT[i] << 16) >> 16;
lY = (lBUFOUT[i] >> 16);
{
float X = NPT * ((float)lX) /32768;
float Y = NPT * ((float)lY) /32768;
float Mag = sqrt(X*X + Y*Y)/NPT;
lBUFMAG[i] = (u32)(Mag * 65536);
}
}
}
// The author uses the Taurus development board, the CPU is STM32F107VC; JLink V8, MDK-ARM 4.10
// Note the symmetry of the FFT calculation results, that is, for the 256-point calculation results, only the first 128 points of data are valid.
// 64-point FFT calculation result diagram (partial):

In the figure above, the harmonic frequency corresponding to the array subscript X is: N×Fs/64=N×3200/64=N*50Hz.
lBUFMAG[1] corresponds to the 50Hz harmonic amplitude
In the above figure, due to the FFT resolution of 50HZ, only 1600Hz harmonics can be recognized at most, resulting in incorrect data in the result.
// 256-point FFT calculation result diagram (partial):
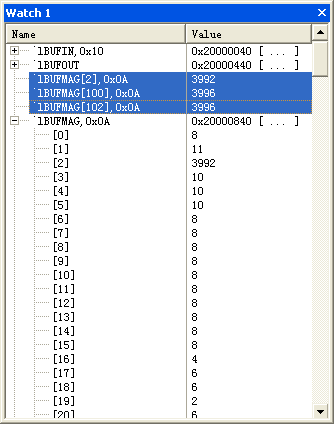
In the figure above, the harmonic frequency corresponding to the array subscript X is: N×Fs/256=N×6400/256=N*25Hz.
lBUFMAG[2] corresponds to 2×25 =50Hz harmonic amplitude
lBUFMAG[100] corresponds to 100×25=2500Hz harmonic amplitude
lBUFMAG[102] corresponds to 102×25=2550Hz harmonic amplitude
// 1024-point FFT calculation result diagram (partial):
In the figure above, the harmonic frequency corresponding to the array subscript X is: N×Fs/1024=N×5120/1024=N*5Hz.
lBUFMAG[10] corresponds to 10×5 =50Hz harmonic amplitude
lBUFMAG[500] corresponds to 500×5=2500Hz harmonic amplitude
lBUFMAG[510] corresponds to 510×5=2550Hz harmonic amplitude
The analog signal source in this project is: 4000 * sin(PI2*i*50.0/Fs) + 4000 * sin(PI2*i*2500.0/Fs) + 4000*sin(PI2*i*2550.0/Fs)
The signal is a mixed signal of 1 50Hz, 1 2500Hz, and 1 2550Hz sine waves, all with an amplitude of 4000.
Previous article:Multifunctional electronic clock based on LPC2103 of ARM7 TDMI-S CPU
Next article:STM32 AD dual channel DMA mode
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [Zero-knowledge ESP8266 tutorial] Quick Start 14-Three IOs control 8 LED lights
- LTE Cat 1 STM32 4G EC200S
- Single-ended to balanced conversion, the actual situation is so different from the simulation, why?
- [NXP Rapid IoT Review] + Preliminary Study on Power Consumption of Some Devices
- Embedded Linux Learning Route Planning
- Today is the summer solstice. I heard that those who went out at noon had no shadow.
- Panta STM32H750 Part 6 (Using timer synchronization to generate a specified number of PWM pulses)
- The TI Cup is coming soon. Which editions have you participated in?
- The topology of the switching power supply in the electric competition must be solved according to the problem-solving ideas
- I encountered a problem. Before the program was burned, the voltage obtained by AIO4 was half of the battery voltage. After the program was burned...