#define _syscall0(type,name) \
There are two '#' signs in one place. I don't understand what they mean. I found a forum post on the Internet:
Usage of "#" and "##" in macros
1. General usage
We use # to turn the macro parameter into a string, and use ## to paste two macro parameters together.
Usage:
#include
#include
using namespace std;
#define STR(s) #s
#define CONS(a,b) int(a##e##b)
int main()
{
printf(STR(vck)); // Output string "vck"
printf("%d
", CONS(2,3)); // 2e3 output: 2000
return 0;
}
2. When the macro parameter is another macro,
it should be noted that the macro parameter will not be expanded if there is '#' or '##' in the macro definition.
1. Non-'#' and '##' cases
#define TOW (2)
#define MUL(a,b) (a*b)
printf("%d*%d=%d
", TOW, TOW, MUL(TOW,TOW));
This line of macro will be expanded to:
printf("%d*%d=%d
", (2), (2), ((2)*(2)));
The parameter TOW in MUL will be expanded to (2).
2, when there is '#' or '##'
#define A (2)
#define STR(s) #s
#define CONS(a,b) int(a##e##b)
printf("int max: %s
", STR(INT_MAX)); // INT_MAX #include
This line will be expanded to:
printf("int max: %s
", "INT_MAX");
printf("%s
", CONS(A, A)); // compile error
This line is:
printf("%s
", int(AeA));
Neither INT_MAX nor A will be expanded, but the solution to this problem is very simple. Add an extra layer of intermediate conversion macro.
The purpose of adding this layer of macro is to expand all macro parameters in this layer, so that the macro (_STR) in the conversion macro can get the correct macro parameters.
#define A (2)
#define _STR(s) #s
#define STR(s) _STR(s) // Conversion macro
#define _CONS(a,b) int(a##e##b)
#define CONS(a,b) _CONS(a,b) // Conversion macroprintf
("int max: %s
", STR(INT_MAX)); // INT_MAX, the maximum value of int type, is a variable #include
Output: int max: 0x7fffffff
STR(INT_MAX) --> _STR(0x7fffffff) Then convert to a string;
printf("%d
", CONS(A, A));
Output: 200
CONS(A, A) --> _CONS((2), (2)) --> int((2)e(2))
III. Some special cases of '#' and '##'
1. Merge anonymous variable names
#define ___ANONYMOUS1(type, var, line) type var##line
#define __ANONYMOUS0(type, line) ___ANONYMOUS1(type, _anonymous, line)
#define ANONYMOUS(type) __ANONYMOUS0(type, __LINE__)
Example: ANONYMOUS(static int); That is: static int _anonymous70; 70 represents the line number;
First level: ANONYMOUS(static int); --> __ANONYMOUS0(static int, __LINE__);
Second level: --> ___ANONYMOUS1(static int, _anonymous, 70);
Third level: --> static int _anonymous70;
That is, only the macro of the current layer can be unlocked each time, so __LINE__ can be unlocked only in the second layer;
2. Filling structure
#define FILL(a) {a, #a}
enum IDD{OPEN, CLOSE};
typedef struct MSG{
IDD id;
const char * msg;
}MSG;
MSG _msg[] = {FILL(OPEN), FILL(CLOSE)};
is equivalent to:
MSG _msg[] = {{OPEN, "OPEN"},
{CLOSE, "CLOSE"}};
3. Record file name
#define _GET_FILE_NAME(f) #f
#define GET_FILE_NAME(f) _GET_FILE_NAME(f)
static char FILE_NAME[] = GET_FILE_NAME(__FILE__);
4. Get the string buffer size corresponding to a numerical type
#define _TYPE_BUF_SIZE(type) sizeof #type
#define TYPE_BUF_SIZE(type) _TYPE_BUF_SIZE(type)
char buf[TYPE_BUF_SIZE(INT_MAX)];
--> char buf[_TYPE_BUF_SIZE(0x7fffffff)];
--> char buf[sizeof "0x7fffffff"];
here is equivalent to:
char buf[11];
I wrote a program under Linux:
#include
#define transform 1##2##3
int main()
{
int result=transform*transform;
printf("the num of result is : %d",result);
return 0;
}
The function output is 15129 (=123*123) - the conclusion is: one of the functions of ## is to connect the two macro parameters together!
Previous article:Embedded problem (codewarrior compilation, download program)
Next article:KEILC51 compile ERROR L104
Recommended ReadingLatest update time:2024-11-15 23:36
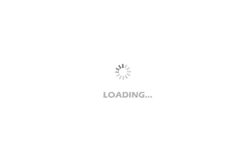
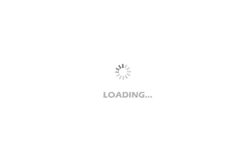
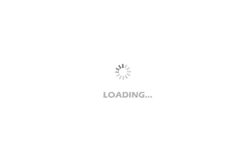
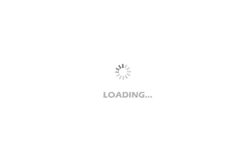
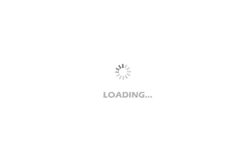
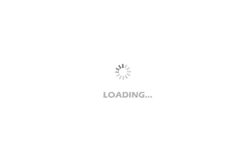
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- msp430f5529 interrupt notes--interrupt nesting
- Wearable ECG monitoring
- General test methods for wireless communication equipment
- I started to enter the Huada MCU again
- Research on Airport Runway Identification Algorithm and Implementation
- Regarding the clamping problem, discuss
- How to drive FT232+stm32?
- CBG201209U201T Product Specifications
- Keil compiles ADuC7029 and reports an error
- Technical Article: Optimizing 48V Mild Hybrid Electric Vehicle Motor Drive Design