1. Query method, that is, the software keeps querying whether it is low (remote control signal) and then reads the header code and
data 0 or 1 code through precise delay.
2. When a signal input is read, turn on the timer counter, and then judge the header code and 0, 1 by reading the number of timer interrupts.
3 RB0 or RB4-RB7 plus timer (or software delay) The advantage of using input interrupts is that real-time control can be performed.
As follows:
;***The transmission is 6221, and the received code value is sent to the PORTC port of ICD for display******
;**************** ***************
org 0000h
nop
goto start
org 0004h
goto serv
;******************************************************
start
bsf status,rp0 ;body 1
movlw 0000h
movwf trisc ;C port output
movlw 0ffh ;B port RB0 input
movwf trisb
movlw B''00000100'' ;1:64
movwf option_reg ;TMR0 timer
bcf status,rp0 ;body 0
clrf intcon ;10010000
bsf intcon,7 ;Open INT falling edge interrupt
bsf intcon,4
bcf intcon,1
clrf portc
loop
sleep
nop
goto loop
;****************Scene protection********************************
serv
movwf w_temp
swapf status,0
clrf status
movwf status_temp
movf pclath,0
movwf pclath_temp
clrf pclath
;***************Remote control processing.******************************
bcf intcon,1
btfsc portb,0 ;Check if RB0 is 0.
goto zdhh ;RB0 is not 0, wrong interrupt. Return to
call delay8 ;Call 8MS delay. Header code detection.
btfsc portb,0 ;Detect header code
goto zdhh ;Not interrupt and return to
call delay5 ;Delay 5MS before data detection.
btfss portb,0 ;Detect if RB0 is high level.
goto zdhh ;Not continue detection.
;********************************************
clrf data1 ;20H
clrf data2 ;21H
clrf data3 ;22H
clrf data4 ;24H
clrf jsp2 ;32-bit counter
clrf sj ;Received data register
clrf jsp1 ;8-bit counter
movlw 0x20 ;20H register to W
movwf fsr ;Send 20H from W to FSR
jc btfsc portb,0 ;Detect if RB0 is low level.
goto jc ;Not continue detection.
;**************0 and 1 data detection********************************
js call delay1 ;delay 1.3MS
btfsc portb,0 ;check whether it is 0 or 1 after the header code,
goto s1 ;check data is not "1"
;*************0 data processing****************************
bcf sj,c ;data is "0"
rrf sj,1 ;0 data right shift to SJ register
goto j1
;**************1 data processing.****************************
s1 bsf sj,c
rrf sj,1
ddp btfsc portb,0
goto ddp
;**************RAM shift***************************
j1 incf jsp1 ;8-bit counting register
incf jsp2 ;32-bit number, counter register.
btfss jsp1,3 ;whether there is 8-bit
goto js
clrf jsp1
movf sj,0 ;shift 8-bit number to W
movwf indf ;W to DATA
incf fsr ;RAM address plus 1
clrf sj
;********************************
btfss jsp2,5 ;Check whether 32 bits are received.
goto js ;If not, check again.
movlw B''10001111'' ;Send IC high 8-bit code
xorwf data1,0 ;Subtraction (Z:1=result is 0;0=result is not 0)
btfss status,2 ;If the result is 0, go to the next step.
goto zdhh ;If the subtraction result is not 0, interrupt and return
movlw B''101010101'' ;Low 8-bit IC code detection
xorwf data2,0
btfss status,2
goto zdhh
;comf data4,0 ;Data inverse code detection. Not used for the time being.
;subwf data3,0
;btfss status,2
;goto zdhh
movf data3,0 ;Send digital data to port C
movwf portc
goto zdhh ;Return from interrupt after receiving 32 bits
;****************zdhh interrupt return procedure*********************
zdhh
movf pclath_temp,0
movwf pclath
swapf status_temp,0 ;Swap the contents of STATUS and W, and restore the body selection.
movwf status ;Send the contents of W to the STATUS register
swapf w_temp,1 ;Swap w_temp
swapf w_temp,0 ;Swap the contents of w_temp and w, and restore the contents of w_temp?
bcf intcon,1
retfie ;Return from interrupt,
;****************8ms************************************
delay8 bcf intcon,5
bcf intcon,2
movlw D''15''
movwf tmr0
loop1 btfss intcon,2
goto loop1
return
;****************5ms********************************
delay5 bcf intcon,5
bcf intcon,2
movlw D''120''
movwf tmr0
loop2 btfss intcon,2
goto loop2
return
;****************1.3ms*******************************
delay1 bcf intcon,5
bcf intcon,2
movlw D''240''
movwf tmr0
loop3 btfss intcon,2
goto loop3
return
;****************
end
There are two possible error codes.
One is that the header is not aligned properly.
The other is that there is an error in data transmission, especially a timing error. The
latter can
be verified by software. If the header is not aligned properly, it is best to send several consecutive sub-headers according to the software regulations and then use the shift method to determine whether there are multiple consecutive identical sub-headers. Align the headers. For example, 20 consecutive 1 0s are converted to indicate that data is ready to be sent and then wait for 1 0. After the transmission is completed, there is a header such as 11110000. In this way, data can be received later.
A I bought a universal remote control outside. It is made of pic16c57c, which means it has no interrupt. I really don't understand how it is made. It has no decoding chip. Does anyone know? I am also working on this. Can anyone give me some tips? Thank you.
B This is very easy.
Last time I used two PIC16C57C chips to make a small module for transmission and reception. It is effective control and no garbled code appears. However, there is a disadvantage that it will be interfered by other infrared products! !
C Infrared reception
uses external interrupts and timing to detect the code, which is very accurate. I use PIC16F72 chip.
D I use an infrared receiving tube with two-stage amplification to see the infrared output waveform. I use a Changhong remote control as the signal source. On the oscilloscope, I have to hit 10ms to see the complete signal waveform, like a waveform after voltage stabilization. I read a book about it and it says that the infrared signal is modulated on a 38khz signal. Why can't I see the waveform when I hit the oscilloscope on 50ns? In this case, there is no way to program. I hope some experts can help me. Thank you.
E You can use the input capture function in CCP. It is very useful. Read the relevant content of input capture carefully. It is very easy.
If an event occurs on the CCP pin (can be set to rising edge, falling edge or other methods), the content of TMR1 is recorded. This is very accurate. Even if the interrupt response is slow, there is no problem. This function is the most appropriate for infrared remote control! !
F Reply to the principle of infrared timing reception.
Use RB0 as interrupt (FIRST: RISING TRIG, SECOND: FALLING TRIG), and start TIMER2 timing (100US) counting at the same time. When the next interrupt comes, TIMER2 has 88 numbers, and 9MS is detected. The rest of the time,
and so on. Note that the interrupt edge of RBO should be changed according to the timing. Based on this principle, it is easy to compile the code.
There is another point worth noting; the attenuation of infrared signals is very strong, so you must consider the choice of its acceptance range.
In fact, any method can be used. Leave enough margin in time when receiving data, which is good for correct data reception.
Previous article:How to use PIC as infrared remote control receiver? (I)
Next article:How to use PIC as infrared remote control receiver? (Part 3)
Recommended ReadingLatest update time:2024-11-15 11:36
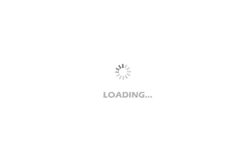
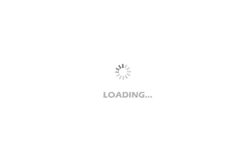
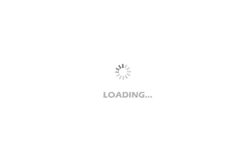
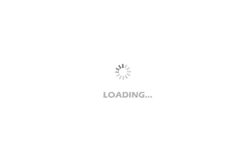
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Real-time driver monitoring system via modal and viewpoint analysis
-
Power Integrated Circuit Technology Theory and Design (Wen Jincai, Chen Keming, Hong Hui, Han Yan)
-
Small Compiler Design Practice (Compiled by Su Mengjin)
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Melexis launches ultra-low power automotive contactless micro-power switch chip
- Melexis launches ultra-low power automotive contactless micro-power switch chip
- Molex leverages SAP solutions to drive smart supply chain collaboration
- Pickering Launches New Future-Proof PXIe Single-Slot Controller for High-Performance Test and Measurement Applications
- Apple faces class action lawsuit from 40 million UK iCloud users, faces $27.6 billion in claims
- Apple faces class action lawsuit from 40 million UK iCloud users, faces $27.6 billion in claims
- The US asked TSMC to restrict the export of high-end chips, and the Ministry of Commerce responded
- The US asked TSMC to restrict the export of high-end chips, and the Ministry of Commerce responded
- ASML predicts that its revenue in 2030 will exceed 457 billion yuan! Gross profit margin 56-60%
- Detailed explanation of intelligent car body perception system
- Use GD32 to make a music spectrum to practice
- Energy Harvesting with MSP430 FRAM Microcontrollers
- SWD cannot be downloaded, what is the reason? ?
- A rather strange op amp, take a look
- Undergraduate entrance examination
- Bill of Materials Problem
- PADS PCB 3D component library
- C2000-GNAG Operation and Use
- HyperLynx High-Speed Circuit Design and Simulation (VI) Non-ideal Transmission Line Differential Pair Eye Diagram (Impedance Mismatch)
- [ESK32-360 Review] 6. Hello! Hello! Hello! Hello!