While designers place great emphasis on coding and simulation, they understandably have little understanding of the internal operation of the chip in the FPGA. As a result, incorrect logic synthesis and timing issues, rather than logic errors, are the source of most logic failures.
However, if designers take the right steps, it is easy to write FPGA code that creates predictable, reliable logic.
In the FPGA design process, logic synthesis and related timing closure need to be performed during the compilation phase. Many aspects, including I/O unit structure, asynchronous logic, and timing constraints, will have a huge impact on the compilation process, resulting in different results in each round of the tool chain. In order to complete timing closure better and faster, let's further explore how to eliminate these differences.
I/O unit structure
All FPGAs have I/O pins that can be highly customized . Customization affects timing, drive strength, termination, and many other aspects. If you do not explicitly define the I/O cell structure, your tool chain will often assume a default structure that you may or may not expect. The purpose of the following VHDL code is to create a bidirectional I/O buffer called sda using the statement "sda: inout ST d_logic;" .
tri_state_proc : PROCESS (sys_clk )
BEGIN
if rising_edge(sys_clk) then
if (enable_in = '1') then
sda <= data_in;
else
data_out <= sda;
sda <= 'Z';
end if;
end if;
END PROC ESS tri_state_proc;
Figure 1 – FPGA Editor view showing some bidirectional I/Os scattered outside the I/O buffers.
When the synthesis tool sees this code, there is no clear indication of how to implement the bidirectional buffer. Therefore, the tool makes its best guess.
One way to accomplish this is to use bidirectional buffers on the FPGA’s I/O ring (in fact, this is the ideal implementation). Another option is to use tri-state output buffers and input buffers, both implemented in look-up table (LUT) logic. The final possibility, and the approach most synthesizers choose, is to use tri-state output buffers on the I/O ring and input buffers in the LUT. All three methods can generate valid logic, but the latter two implementations incur longer routing delays when transferring signals between the I/O pins and the LUT. They also require additional timing constraints to ensure timing closure. The FPGA Editor clearly shows that in Figure 1, some of our bidirectional I/Os are scattered outside the I/O buffers.
The lesson is to remember not to let the synthesis tool guess how to implement critical parts of your code. Even if the synthesized logic happens to work as you expect, it is possible that things may change when the synthesis tool enters a new version. Your I/O logic and all critical logic should be explicitly defined. The following VHDL code shows how to implicitly define an I/O buffer using the Xilinx ? IOBUF primitive. Also note that all electrical characteristics of the buffer are explicitly defined in a similar manner.
sda_buff: IOBUF
generic map (IOSTANDARD => "LVC MOS 25",
IFD_DELAY_VALUE => "0", DRIVE => 12,
SLEW => "SLOW")
port map(o=> data_out, io=> sda,
i=> data_in, t=> enable_in);
Disadvantages of asynchronous logic
Asynchronous code creates logic that is difficult to constrain, simulate, and debug. Asynchronous logic often creates intermittent errors that are nearly impossible to reproduce. Additionally, it is impossible to generate testbenches to detect errors caused by asynchronous logic.
Although asynchronous logic may seem easy to detect, in reality it often goes undetected; therefore, designers must be careful of the many ways asynchronous logic can hide in their designs. All clocked logic requires a minimum setup and hold time, and this also applies to the reset input of a flip-flop . The following code uses an asynchronous reset. There is no way to apply timing constraints here to meet the setup and hold time requirements of the flip-flop.
data_proc: PROCESS (sys_clk,reset)
BEGIN
if (reset = '1') then
data_in <= '0';
elsif rising_edge(sys_clk) then
data_in <= serial_in;
end if;
END PROCESS data_proc;
The following code uses a synchronous reset. However, the reset signal for most systems may be a pushbutton switch or some other source that is not related to the system clock . Although the reset signal is mostly static and asserted or deasserted for long periods of time, its level can still vary. Reset deassertion, which corresponds to the rising edge of the system clock, can violate the setup time requirement of the flip-flop, which cannot be constrained.
data_proc : PROCESS (sys_clk)
BEGIN
if rising_edge(sys_clk) then
if (reset = '1') then
data_in <= '0';
else
data_in <= serial_in;
end if;
end if;
END PROCESS data_proc;
This problem is easy to fix once we understand that we cannot feed asynchronous signals directly into our synchronous logic. The following code creates a new reset signal called sys_reset that is synchronized to our system clock sys_clk. Metastability issues arise when sampling asynchronous logic. We can reduce the chance of this problem by sampling with a ladder that is ANDed with the previous stages of the ladder.
data_proc : PROCESS (sys_clk)
BEGIN
if rising_edge(sys_clk) then
reset_1 <= reset;
reset_2 <= reset_1 and reset;
sys_reset <= reset_2 and reset_1
and reset;
end if;
if rising_edge(sys_clk) then
if (sys_reset = '1') then
data_in <= '0';
else
data_in <= serial_in;
end if;
end if;
END PROCESS data_proc;
So far, it has been assumed that you have carefully synchronized all of your logic. However, if you are not careful, it is easy for your logic to become decoupled from the system clock. Do not allow your toolchain to use local routing resources used by the system clock. You will not be able to constrain your logic if you do so. Remember to clearly define all important logic.
The following VHDL code uses the Xilinx BUFG primitive to force sys_clk into a dedicated high fan-out buffer that drives a low-skew net .
gclk1: BUFG port map (I => sys_clk,O
=>sys_clk_bufg);
data_proc: PROCESS (sys_clk_bufg)
BEGIN
if rising_edge(sys_clk_bufg) then
reset_1 <= reset;
reset_2 <= reset_1 and reset;
sys_reset <= reset_2 and reset_1
and reset;
end if;
if rising_edge(sys_clk_bufg) then
if (sys_reset = '1') then
data_in <= '0';
else
data_in <= serial_in;
end if;
end if;
END PROCESS data_proc;
Some designs use split versions of a single master clock to process the deserialized data. The following VHDL code (nibble_proc process) illustrates data acquired at one-quarter the system clock frequency.
data_proc: PROCESS (sys_clk_bufg)
BEGIN
if rising_edge(sys_clk_bufg) then
reset_1 <= reset;
reset_2 <= reset_1 and reset;
sys_reset <= reset_2 and reset_1
and reset;
end if;
if rising_edge(sys_clk_bufg) then
if (sys_reset = '1') then
two_bit_counter <= "00";
divide_by_4 <= '0';
nibble_wide_data <= "0000";
else
two_bit_counter
<= two_bit_counter + 1;
divide_by_4 <= two_bit_counter(0) and
two_bit_counter(1);
nibble_wide_data(0)
<= serial_in;
nibble_wide_data(1)
<= nibble_wide_data(0);
nibble_wide_data(2)
<= nibble_wide_data(1);
nibble_wide_data(3)
<= nibble_wide_data(2);
end if;
end if;
END PROCESS data_proc;
nibble_proc : PROCESS (divide_by_4)
BEGIN
if rising_edge(divide_by_4) then
if (sys_reset = '1') then
nibble_data_in <= "0000";
else
nibble_data_in
<= nibble_wide_data;
end if;
end if;
END PROCESS nibble_proc;
It looks like everything is synchronized, but nibble_proc uses the product term divide_by_4 to sample nibble_wide_data from the clock domain sys_clk_bufg. Due to routing delays, there is no clear phase relationship between divide_by_4 and sys_clk_bufg. Moving divide_by_4 to BUFG does not help because this process introduces routing delays. The solution is to keep nibble_proc in the sys_clk_bufg domain and use divide_by_4 as the qualifier, as shown below.
nibble_proc: PROCESS (sys_clk_bufg)
BEGIN
if rising_edge(sys_clk_bufg) then
if (sys_reset = '1') then
nibble_data_in <= "0000";
elsif (divide_by_4 = '1') then
nibble_data_in
<= nibble_wide_data;
end if;
end if;
END PROCESS nibble_proc
Importance of Timing Constraints
If you want your logic to run correctly, you must use the correct timing constraints. If you have taken care to ensure that your code is all synchronous and all I/Os are registered, these steps can greatly simplify timing closure. Using the above code and assuming a 100MHz system clock , the timing constraint file can be easily completed with just four lines of code, as shown below:
NET sys_clk_bufg TNM_NET =
sys_clk_bufg;
TI MESPEC TS_sys_clk_bufg = PERIOD
sys_clk_bufg 10 ns HIGH 50%;
FFSET = IN 6 ns BEFORE sys_clk;
FFSET = OUT 6 ns AFTER sys_clk;
Please note: The setup and hold times for the I/O register logic in Xilinx FPGAs are very fixed and should not change much within a package. However, we still include them, primarily as a verification step to ensure that the design meets its system parameters.
Three simple steps
By following these three simple steps, designers can easily implement reliable code.
• Never let the synthesis tool guess what you expect. Explicitly define all I/O pins and critical logic using Xilinx primitives. Make sure to define the electrical characteristics of the I/O pins;
• Ensure that the logic is 100% synchronous and that all logic is referenced to the master clock domain;
• Apply timing constraints to ensure timing closure.
By following the three steps above, you can eliminate the variance caused by synthesis and timing. Removing these two major obstacles will give you code with 100% reliability.
Previous article:FPGAs are key to green search technology
Next article:High-speed data acquisition system based on AVR and CPLD
Recommended ReadingLatest update time:2024-11-16 21:50
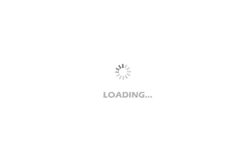
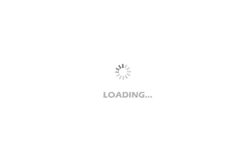
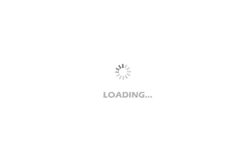
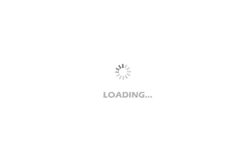
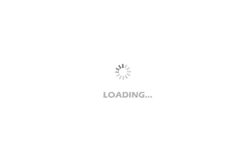
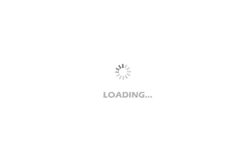
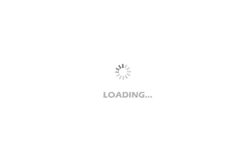
- Popular Resources
- Popular amplifiers
-
Analysis and Implementation of MAC Protocol for Wireless Sensor Networks (by Yang Zhijun, Xie Xianjie, and Ding Hongwei)
-
MATLAB and FPGA implementation of wireless communication
-
Intelligent computing systems (Chen Yunji, Li Ling, Li Wei, Guo Qi, Du Zidong)
-
Summary of non-synthesizable statements in FPGA
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- MicroPython - Python for microcontrollers
- Wireless transmission power quality monitoring system circuit
- 2020 Share my thoughts on Qian'an, a national healthy and civilized county-level city
- Current detection methods
- [CB5654 Intelligent Voice Development Board Review] Hardware Review
- FPGA Implementation of Floating-Point LMS Algorithm
- Selection of aluminum electrolytic capacitors
- EEWORLD University Hall ---- FanySkill Installation Instructions For Allegro 16.617.2 Version
- Yibite provides Bluetooth, 4G DTU, WiFi, lora and other modules for free. Come and play if you want to!
- [GD32L233C-START Review] 3. Software GPIO simulation + hardware SPI drive LCD screen