This article mainly introduces the basic characteristics of PIC's C language based on HiTech PICC .
1 Features of HiTech PICC language
PICC basically complies with the ANSI standard, but does not support recursive function calls. The main reason is the special stack structure of the PIC microcontroller. The stack in the PIC microcontroller is implemented in hardware, and its depth is fixed with the chip, so it is impossible to implement recursive algorithms that require a large number of stack operations; in addition, the efficiency of implementing software stacks in PIC microcontrollers is not very high. For this reason, the PICC compiler uses a "static overlay" technology to allocate a fixed address space to local variables in C language functions. The machine code generated after this treatment is very efficient. When the code size exceeds 4KB, the difference between the length of the code compiled by C language and the code implemented entirely in assembly code is not very large (<10%). Of course, the premise is that you need to pay attention to the efficiency of the statements written during the entire C code writing process.
2 Variables in PICC
The variable types in PICC are the same as those in standard C language, so they will not be repeated here. In order to make the compiler generate the most efficient machine code, PICC lets the programmer manage the banks of data registers in the microcontroller. Therefore, when defining user variables, you must decide which bank to put these variables in. If not specified, the defined variables will be located in bank0. The corresponding bank number must be added in front of the variables defined in other banks, for example:
bank1 unsigned char temp; // variable is located in bank1
The data register bank size of the mid-range PIC microcontroller is 128 bytes. Except for the special function register area of the first few bytes, the total number of variable bytes defined in a bank in C language cannot exceed the number of available RAM bytes. If the bank capacity is exceeded, an error will be reported at the last connection, and the general information is as follows:
Error[000]:Can't find 0x12C words for psect rbss_1 in segmentBANK1
The linker prompts that there are a total of 0x12c (300) bytes to be placed in bank 1, but the capacity of bank 1 is insufficient. Although the bank location of the variable must be determined by the programmer, there is no need to specifically write instructions to set the bank before performing variable access operations when writing the source program. The C compiler will automatically generate assembly instructions for the corresponding bank settings based on the object being operated. In order to avoid frequent bank switching and improve code efficiency, try to locate variables that implement the same task in the same bank; when reading and writing variables in different banks, try to merge variables in the same bank together for continuous operations.
Bit variables can only be global or static. PICC will merge 8 bit variables located in the same bank into one byte and store it at a fixed address. PICC implements bit addressing for the entire data storage space. The address of the 0th bit of the 0x000 unit is 0x0000, and so on. Each byte has 8 bit addresses. If a bit variable flag1 is addressed as 0x123, the actual storage space is located at:
Byte address = 0x123/8 = 0x24
Bit offset = 0x123%8 = 3
That is, the flag1 bit variable is located at the third bit of the byte at address 0x24. When debugging the program, if you want to observe the change of flag1, you must observe the byte at address 0x24 instead of 0x123. When PICC compiles the original code, whenever possible, the operation of ordinary variables will be implemented with the simplest bit operation instructions. Assuming that a byte variable tmp is finally located at address 0x20, then
tmp | =0x80=>bsf 0x20.7
In addition, the function can return a bit variable, and the returned bit variable will be stored in the carry bit of the microcontroller and returned.
3 Pointers in PICC
3.1 Pointers to RAM
When PICC compiles the C source program, the pointer operation pointing to RAM is finally implemented with FSR for indirect addressing. The range that FSR can directly and continuously address is 256B, so a pointer can cover the storage area of 2 banks at the same time (bank0/1 or bank2/3, one bank area is 128B). To cover the maximum 512B of internal data storage space, the addressing area applicable to the pointer must be clearly specified when defining the pointer. For example:
unsigned char *pointer0; //Define the pointer that overwrites bank0/1
bank2 char *pointer1; //Define the pointer that covers bank2/3
Since the defined pointer has a clear bank applicable area, type matching must be achieved when assigning values to pointer variables, otherwise an error will occur, for example:
unsigned char *pointer0; //define the pointer to bank0/1
bank2 unsigned char buff; //define a buffer in bank2/3
Program statements:
pointer() = buff; //Error! Trying to assign the address of a variable in bank2 to a pointer pointing to bank0/1
If such incorrect pointer operation occurs, the PICC will notify you of the following information at the last link:
Fixup overflow in expression (…)
3.2 Pointers to ROM constants
If a set of variables are constants that have been defined in the ROM area, then the pointer to them can be defined like this:
const unsigned char company[]="software"
3.3 Pointers to functions
Because the efficiency of implementing function pointer calls on the specific architecture of PIC microcontrollers is not high, it is recommended that you try not to use function pointers unless required by special algorithms.
4 Subroutines and functions in PICC
The program space of the mid-range PIC microcontroller has the concept of paging, but you don't need to worry too much about the paging of the code when programming in C language, because the page setting when calling all functions or subroutines (if the code exceeds one page) is implemented by the instructions automatically generated by the compiler.
4.1 Function code length limit
PICC determines that the machine code generated by a function in a C source program after compilation must be placed in the same program page. The length of a program page of a mid-range PIC microcontroller is 2KB, and the code generated by any function written in C language cannot exceed 2KB. If you really need to write a long program continuously to achieve a specific function, then you must split these continuous codes into several functions to ensure that the code compiled by each function does not exceed one page space.
4.2 Call hierarchy control
PIC microcontrollers use hardware stacks, so the function call level will be subject to certain restrictions during programming. Generally, the hardware stack depth of the mid-range microcontrollers in the PIC series is 8 levels. The programmer must control the nesting depth of the subroutine call to meet this restriction. After the final compilation and linking of PICC is successful, a link positioning mapping file (*.map) can be generated. In this file, there is a detailed function call nesting indicator graph "call graph". Some function calls are library functions automatically added during compilation. These function calls cannot be directly seen from the C source program, but they are clear at a glance on the nesting indicator graph.
5 Mixed programming of C language and assembly language
Some special instructions of the microcontroller have no direct corresponding description in the standard C language syntax, such as the clear watchdog instruction "clrwdt" and the sleep instruction "sleep" of the PIC microcontroller; the microcontroller system emphasizes the real-time control. In order to achieve this requirement, sometimes it is necessary to use assembly instructions to implement part of the code to improve the efficiency of program operation. There are two ways to embed assembly instructions in C programs.
① If only a few assembly instructions need to be embedded, PICC provides a function-like statement:
asm("clrwdt");
This is the most direct and easiest way to embed assembly instructions directly in a C source program.
② If you need to write a continuous assembly instruction, PICC supports another syntax description: use "#asm" to start the assembly instruction segment and "#endasm" to end it. For example:
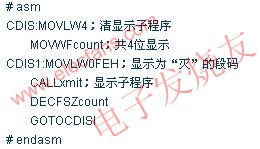
5.1 Assembly instructions addressing global variables defined in C language
All symbols defined in C language will be automatically preceded by an underscore "_" after compilation. Therefore, if you want to address various variables defined in C language in assembly instructions, you must add the "_" symbol in front of the variable. For example, count in the above example is an unsigned global variable defined in C language. In assembly language, you only need to add the "_" symbol in front of it to access it. In addition, for multi-byte global variables defined in C language, such as the following definition in C language:
int advalue;
In assembly language, access is done by byte, for example:
asm("movf_advalue+0.0"); //Send the number in the low byte of advalue to w
asm("rrf_advalue+1") //Shift the number in the high byte of advalue one bit to the left
5.2 Assembly instructions addressing local variables of C functions
As mentioned above, PICC uses a "static overwrite" technology for automatic local variables (including entry parameters when calling functions), and determines a fixed address (located in bank0) for each variable. When the embedded assembly instructions address it, they only need to use the direct addressing method of the data register. Therefore, the key is to know the addressing symbols of these local variables. It is recommended that readers first write a short C code with the simplest local variable operation instructions, and compile this source code into the corresponding PICC assembly instructions; check how the assembly instructions generated by the C compiler address these local variables, and use the same addressing method for the inline assembly instructions written by yourself.
Compared with assembly language, the advantages of programming in C language are unquestionable: development efficiency is greatly improved, humanized statement instructions and modular programs are easy to manage and maintain, and programs are easy to port between different platforms. Therefore, since you use C language for programming, you should try to avoid embedding assembly instructions or writing assembly instruction module files. For example:
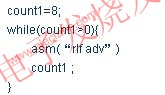
It is very inconvenient to implement the variable right-shift operation in C language. The PIC microcontroller has the corresponding shift operation assembly instruction, so it is most efficient to implement it in the form of embedded assembly. In fact, the decrement of the variable count1 can also be directly implemented with assembly instructions, which can save code, but it is more intuitive and easier to maintain to describe it in standard C language.
6. Notes
① Since all local variables will occupy the storage space of bank0, the number of bytes of variables that users can locate in bank0 will be subject to certain restrictions. Please pay attention to this in actual use.
② When a non-bit variable is forcibly converted into a bit variable in the program, please note that the compiler only judges the lowest bit of the ordinary variable: if the lowest bit is 0, it is converted into bit variable 0; if the lowest bit is 1, it is converted into bit variable 1.
③ Since the internal resources of the PIC series microcontrollers are very limited, unsigned character type variables should be used as much as possible under permitted conditions to save space.
④ PICC does not reserve address space for absolutely positioned variables, for example:
unsigned char advalue @ 0x20; //advalue is located at address 0x20, which is equivalent to a pseudo-instruction in assembly language
advalue EQU 20H
So please use it with caution.
⑤ Try to use global variables for parameter passing. The biggest advantage of using global variables is that addressing is intuitive. You only need to add an underscore character before the variable name defined in C language to address it in the assembly statement. The efficiency of using global variables for parameter passing is also higher than that of formal parameters.
⑥ For multi-byte variables (such as int type, float type variables, etc.), PICC follows the Little Endian standard, that is, the low byte is placed at the low address of the storage space, and the high byte is placed at the high address. Please pay attention when programming.
7 Conclusion
Generally, the codes generated by C language are relatively cumbersome, so to write high-quality and practical C language programs, you must have a detailed understanding of the microcontroller architecture and hardware resources. Using C language to develop PIC series microcontroller system software has the advantages of high code writing efficiency, intuitive software debugging, convenient maintenance and upgrading, high code reuse rate, and convenient cross-platform code transplantation. Therefore, the application of C language programming in microcontroller system design will become more and more extensive.
Previous article:Adjustable countdown reminder made with PIC16F627
Next article:Design of hands-free passive keyless access control based on PIC16F639
Recommended ReadingLatest update time:2024-11-16 19:57
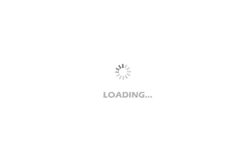
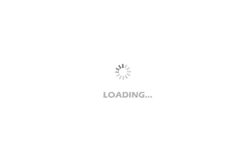
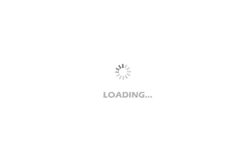
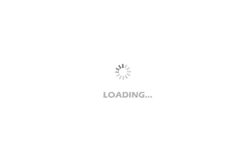
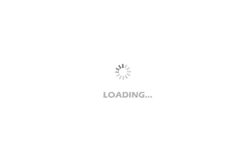
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Modern Compiler Principles C Language Description (Ampel)
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Power Integrated Circuit Technology Theory and Design (Wen Jincai, Chen Keming, Hong Hui, Han Yan)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Working waveform problem of single-phase bridge uncontrolled rectifier circuit with capacitor filtering
- Abnormal output of op amp
- Guys, please help me find out what the problem is.
- I would like to ask about the interrupt problem of msp430f5529
- Three questions on embedded ARM basics
- [Rawpixel RVB2601 development board trial experience] GRB breathing light
- Dancing flame pendant
- Do you purchase samples or apply for them for free?
- EEWorld interviews Toshiba PCIM booth engineer: 2-minute short video shows you key products and solutions
- Is it necessary to learn TI's KeystoneII series multi-core heterogeneous processors?