/*-------------------------
*First of all, I am a novice and just started to write driver programs. I will explain the development environment (host system redhat6.3, development board ARM-s3c6410)
Take watchdog (commonly known as dog interrupt) as an example to write a simple interrupt (driver)
-------------------------*/
#include
#include
#include
#include
#include
#include
#include
MODULE_LICENSE("GPL");
MODULE_AUTHOR("cheng");
typedef struct mydev{
unsigned long gpio_virt;
unsigned long wdt_virt;
unsigned long *gpmcon, *gpmdat;
unsigned long *wtcon, *wtdat, *wtcnt, *wtclrint;
void (*init_nydev)(struct mydev *this);
void (*exit_mydev)(struct mydev *this);
irqreturn_t (*do_irq)(int irq, struct mydev *this);
void (*led_on)(struct mydev *this);
void (*led_off)(struct mydev *this);
void (*wdt_on)(struct mydev *this);
void (*wdt_off)(struct mydev *this);
}MYDEV;
void my_init_mydev(struct mydev *this);
void my_exit_mydev(struct mydev *this);
irqreturn_t my_do_irq(int irq, struct mydev *this);
void my_led_on(struct mydev *this);
void my_led_off(struct mydev *this);
void my_wdt_on(struct mydev *this);
void my_wdt_off(struct mydev *this);
MYDEV my ;
static int test_init(void)
{
printk("hello,in my test_initn");
my.init_mydev = my_init_mydev;
my.exit_mydev = my_exit_mydev;
my.init_mydev(&my);
return 0;
}
void test_exit()
{
my.exit_mydev(&my);
printk("this is test exitn");
}
module_init(test_init);
module_exit(test_exit);
void my_init_mydev(struct mydev *this)
{
this->do_irq = my_do_irq;
this->led_on = my_led_on;
this->led_off = my_led_off;
this->wdt_on = my_wdt_on;
this->wdt_off = my_wdt_off;
int ret = request_irq(IRQ_WDT, this->do_irq, IRQF_SHARED,"hello", this);
if(ret < 0){
printk("request_irq errorn");
return ;
}
this->gpio_virt = ioremap(0x7f008000, SZ_4K);
this->wdt_virt = ioremap(0x7e004000, SZ_4K);
this->gpmcon = this->gpio_virt + 0x820;
this->gpmdat = this->gpio_virt + 0x824;
this->wtcon = this->wdt_virt + 0x00;
this->wtdat = this->wdt_virt + 0x04;
this->wtcnt = this->wdt_virt + 0x08;
this->wtclrint = this->wdt_virt + 0x0c;
this->wdt_on(this);
}
void my_exit_mydev(struct mydev *this)
{
this->my_wtd_off(this);
iounmap(this->wdt_virt);
iounmap(this->gpio_virt);
free_irq(IRQ_WDT,this);
}
irqreturn_t my_do_irq(int irq, struct mydev *this)
{
if(irq == IRQ_WDT){
*this->wtclrint = 0;
printk("wang wang wangn");
static int flag = 1;
if(flag)
this->led_on(this);
else
this->led_off(this);
flag ^= 1;
}
return IRQ_HANDLED;
}
void my_led_on(struct mydev *this)
{
*this->gpmcon = 1;
*this->gpmdat = 0;
}
void my_led_off(struct mydev *this)
{
*this->gpmcon = 1;
*this->gpmdat = 1;
}
void my_wdt_on(struct mydev *this)
{
*this->wtcon = (1 << 2) | (1 << 3) | (1 << 5) | (31 << 8);
*this->wtdat = 0x4000;
*this->wtcnt = 0x8000;
}
void my_wdt_off(struct mydev *this)
{
*this->wtcon = 0;
}
/*
Here is the Makefile content:
*/
all:
make -C linux-2.6.28_smdk6410 M=`pwd` modules
clean:
make -C /linux-2.6.28_smdk6410 M=`pwd` clean
rm -rf modules.order
obj-m += test.o
Previous article:S3C6410 development board development environment construction
Next article:【Embedded Development】Burn the Linux system into the development board - Model S3C6410
Recommended ReadingLatest update time:2024-11-22 23:23
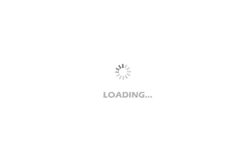
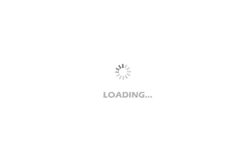
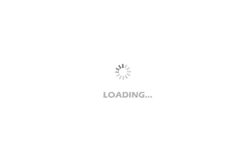
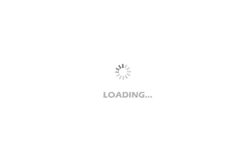
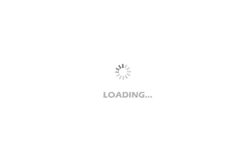
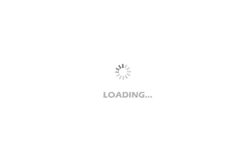
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Application skills/Development of intelligent telephone alarm
- 1000 posts, commemorating
- This is a circuit for detecting the grid voltage. I would like to ask how the differential circuit works.
- BMP library management based on FPGA.pdf
- I need help with the simplest program to control 12864 with keys
- TUSB9261 -- USB3.0 to SATA interface bridge chip programming guide
- 【ST NUCLEO-H743ZI Review】1. Unboxing
- Embedded Technology and Application Development Project Tutorial: STM32 Edition
- AM335x Evaluation Board Quick Test (2)
- TMS320C55x assembly language knowledge--linker relocation of program