1. Description
PART 3 SPI (SCLK, SDO, SDI) full duplex
SCLK clock, SDO (MOSI) data output, SDI (MISO) data input
8051SPI and I2C also need to use IO simulation
There are 4 types of SPI bus transmission:
CPOL bit, high level: 1, low level: 0
CPHA bit, edge, read first then write: 0, write first then read: 1
When transmitting, transmit the high bit first and then the low bit
The experimental program uses SPI serial bus EEPROM, 25AA040A
process
Read data: Write the read command first, then write the read address
Write data: first latch the write address, then use the write command, write the address, and then write the data
A8 If it is a 9-bit register, valid 1, 8-bit is 0
2. Demonstration
3. Timing
Command word
Read and write timing
Write Enable
Code:
/**
* 8051/2 DEMO 3
* Commonly used local communication methods
* 1. Serial port UART, baud rate: 9600
* When connecting a device, generally only GND RX TX is connected, and Vcc is not connected to avoid power supply conflicts with the target device.
* 1.1 RS485 standard (+2V ~ +6V: 1 / -6V ~ -2V: 0)
* 1.2 RS232 standard (-15V ~ -3V: 1 / +3V ~ +15V: 0), MAX232 needs to perform level inversion before communication with MCU
* 1.3 TTL standard (2.4V--5V:1 / 0V--0.5V:0)
*
* 2.I2C(SCL,SDA)
* 3. SPI (SCLK, SDO, SDI) full duplex
*/
#include "REG52.H"
#include typedef unsigned char U8; /** * PART 3 SPI (SCLK, SDO, SDI) full duplex * SCLK clock * SDO (MOSI) data output * SDI (MISO) data input * 8051SPI and I2C also need to use IO simulation * There are 4 types of SPI bus transmission: * CPOL bit, high level: 1, low level: 0 * CPHA bit, edge, read first then write: 0, write first then read: 1 * When transmitting, transmit the high bit first and then the low bit * * The experimental program uses SPI serial bus EEPROM, 25AA040A * process * Read data: Write the read command first, then write the read address * Write data: first latch the write address, then use the write command, write the address, and then write the data * * A8 If it is a 9-bit register, valid 1, 8-bit is 0 **/ #define READ 0x03 #define WRITE 0x02 #define WREN 0x06 sbit SCK = P2^4; sbit SI = P2^5; sbit SO = P2^6; sbit CS = P2^7; //Serial port initialization - for printing data void uart_init() { SCON = 0x50; TMOD = 0x20; //Select timer 1 //Install the high 8 bits and low 8 bits of the baud rate, 9600 TH1 = 0xFD; TL1 = 0xFD; ES = 1; //Open serial port interrupt TR1 = 1; //Turn on timer 1 EA = 1; // Enable general interrupt } //Send a character void uart_send_char(char str) { SBUF = str; //Put the string to be sent into the buffer while(TI==0); //Wait for sending to complete TI=0; // Clear the send interrupt flag } //Serial port interrupt service processing void uart_handle() interrupt 4 { } //Write 1 byte void spi_write(U8 dat) { U8 i=0; for(i=0;i<8;i++) { SCK = 0; //Read SI data when a rising edge occurs // Take the data bits in sequence and give them to SI SI=given>>7; that=that<<1; SCK = 1; } } //After reading the rising edge, read the data U8 spi_read() { U8 i=0; U8 that=0; for(i=0;i<8;i++) { SCK = 0; SCK = 1; //Put the data bits into SO in sequence dat <<= 1; that|=SO; } return that; } //Read eeprom operation U8 spi_device_read(U8 addr) { U8 that=0; CS = 0; spi_write(READ); spi_write(addr); that = spi_read(); CS = 1; // Pull CS back to high level after reading return that; } void delay5ms() //Error -0.000000000001us { unsigned char a,b; for(b=15;b>0;b--) for(a=152;a>0;a--); } //Write eeprom operation void spi_device_write(U8 addr,U8 dat){ //Write ready CS = 0; spi_write(WREN); CS = 1; //pull high CS = 0; spi_write(WRITE); //Use write command spi_write(addr); spi_write(dat); //Whether the write is successful or not, delay 5ms delay5ms(); CS = 1; } void delay1s() //error 0us { unsigned char a,b,c; for(c=167;c>0;c--) for(b=171;b>0;b--) for(a=16;a>0;a--); } void main() { U8 res1; //Initialize the serial port to display the data to the serial port uart_init(); //Write data, address 1, write value 2 delay1s(); spi_device_write(1,0x11); delay1s(); res1 = spi_device_read(1); //Send to the serial port uart_send_char(res1); while (1){ } }
Previous article:8051/2 single chip microcomputer DHT11 air temperature and humidity sensor value
Next article:Common local communication methods for 8051/2 microcontrollers: UART, RS485, I2C, SPI, I2C E2PROM 3
Recommended ReadingLatest update time:2024-11-22 20:05
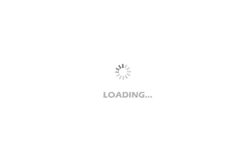
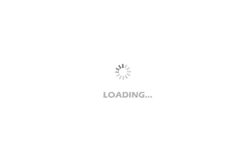
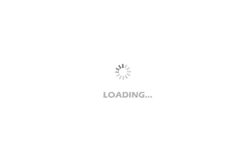
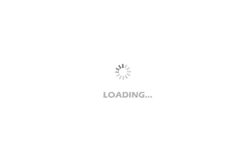
- Popular Resources
- Popular amplifiers
-
Follow me Season 2 Episode 3 Mission Code
-
Microgrid Stability Analysis and Control Microgrid Modeling Stability Analysis and Control to Improve Power Distribution and Power Flow Control (
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
MATLAB and FPGA implementation of wireless communication
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Another technical solution for power-type plug-in hybrid: A brief discussion on Volvo T8 plug-in hybrid technology
- Bridge isolation and pin tube real part to reflective type
- It’s dangerous to make phone calls at a gas station, so is it safe to pay by scanning a QR code with your mobile phone?
- MSP430 MCU's own hardware SPI communication
- Is this description correct for the three working conditions of a transistor? To achieve these conditions, how should the peripheral circuit...
- Microstrip transmission line impedance matching engineering example
- 《Warehouse temperature alarm system based on GD32》
- 5-minute quick talk - Nichicon small lithium-ion rechargeable battery
- Biological test paper discharge current peak detection circuit
- What methods can be used to generate a ±0.2V rectangular wave?
- Four common ideas and techniques for FPGA design