DS18B20——Temperature sensor. The microcontroller can communicate with DS18B20 through 1-Wire and finally read the temperature. The hardware interface of 1-Wire bus is very simple. You only need to connect the data pin of 18B20 to an IO port of the microcontroller to communicate. The temperature storage value with a maximum value of 12 is stored in the form of complement.
2 bytes, LSB low byte, MSB high byte, -55~125
1. Initialization
Detect the existence of pulses: If there is a DS18B20 on the bus, the bus will return a low-level pulse according to the timing requirements. The microcontroller needs to pull down this pin for about 480us to 960us, and it lasts for 500us in our program. Then, the microcontroller releases the bus, that is, gives a high level. After waiting for about 15 to 60us, the DS18B20 will actively pull down this pin for about 60 to 240us, and then the DS18B20 will actively release the bus, so that the IO port will be automatically pulled up by the pull-up resistor.
2. ROM operation instructions
Skip ROM: 0xCC. When there is only one device on the bus, ROM can be skipped and no ROM detection is performed.
3. RAM memory operation instructions
Read Scratchpad: 0xBE - The temperature data of DS18B20 is 2 bytes. When we read the data, we first read the low bit of the low byte. After reading the first byte, we read the low bit of the high byte until both bytes are read.
Convert Temperature (start temperature conversion): 0x44 - 12-bit maximum conversion time is 750ms
4. DS18B20 bit write timing
When writing '0' to DS18B20, the microcontroller directly pulls the pin low for a duration greater than 60us and less than 120us. The figure shows that after the microcontroller pulls the pin low for 15us, DS18B20 will read this bit between 15us and 60us. DS18B20 will read at the earliest 15us, the typical value is 30us, and it will not exceed 60us at most. DS18B20 will definitely finish reading, so the duration can be more than 60us.
When writing '1' to DS18B20, the microcontroller first pulls the pin low for more than 1us, then immediately releases the bus, i.e. pulls the pin high for more than 60us. Similar to writing '0', DS18B20 will read the '1' between 15 and 60us.
5. DS18B20 bit read timing
The MCU must first pull down this pin and keep it for at least 1us, then release the pin and read it as soon as possible after release. From pulling down this pin to reading the pin status, it cannot exceed 15us. As you can see from Figure 16-17, the master sampling time, that is, MASTER SAMPLES, must be completed within 15us.
#include #include typedef unsigned char uchar; sbit IO_18B20 = P3 ^ 2; //DS18B20 communication pin /* Software delay function, delay time (t*10)us */ void DelayX10us(volatile t) { do { _nop_(); _nop_(); _nop_(); _nop_(); _nop_(); _nop_(); _nop_(); _nop_(); } while (--t); } /* Reset the bus and get a presence pulse to start a read or write operation */ bit Get18B20Ack() { bit ack; EA = 0; //Disable general interrupt IO_18B20 = 0; //Generate 500us reset pulse DelayX10us(50); IO_18B20 = 1; DelayX10us(6); //delay 60us ack = IO_18B20; //Read the existence pulse while(!IO_18B20); //Wait for the pulse to end EA = 1; //Re-enable the general interrupt return ack; } /* Write a byte to DS18B20, dat-byte to be written*/ void Write18B20(uchar dat) { flying mask; EA = 0; //Disable general interrupt for (mask = 0x01; mask != 0; mask <<= 1) // Low bit first, shift out 8 bits in sequence { IO_18B20 = 0; //Generate 2us low level pulse _nop_(); _nop_(); if ((mask & dat) == 0) //output the bit value { IO_18B20 = 0; } else { IO_18B20 = 1; } DelayX10us(6); //delay 60us IO_18B20 = 1; //Pull up the communication pin } EA = 1; //Re-enable the general interrupt } /* Read a byte from DS18B20, return value - the byte read*/ fly Read18B20() { flying dat; flying mask; EA = 0; //Disable general interrupt for (mask = 0x01; mask != 0; mask <<= 1) //Low bit first, collect 8 bits in sequence { IO_18B20 = 0; //Generate 2us low level pulse _nop_(); _nop_(); IO_18B20 = 1; //End low level pulse, wait for 18B20 to output data _nop_(); //Delay 2us _nop_(); if (!IO_18B20) //Read the value on the communication pin { dat &= ~mask; } else { that |= mask; } DelayX10us(6); //Delay another 60us } EA = 1; //Re-enable the general interrupt return that; } /* Start a 18B20 temperature conversion, return value - indicates whether the start is successful*/ bit Start18B20() { bit ack; ack = Get18B20Ack(); //Execute bus reset and get 18B20 response if (ack == 0) //If 18B20 responds correctly, a conversion is started { Write18B20(0xCC); //Skip ROM operation Write18B20(0x44); //Start a temperature conversion } return ~ack; //ack==0 means the operation is successful, so the return value is negated } /* Read the temperature value converted by DS18B20, and return value - indicates whether the reading is successful*/ bit Get18B20Temp(int *temp) { bit ack; uchar LSB, MSB; //16bit temperature value low byte and high byte ack = Get18B20Ack(); //Execute bus reset and get 18B20 response if (ack == 0) //If 18B20 responds correctly, read the temperature value { Write18B20(0xCC); //Skip ROM operation Write18B20(0xBE); //Send read command LSB = Read18B20(); //Read the low byte of the temperature value MSB = Read18B20(); //Read the high byte of the temperature value *temp = ((int)MSB << 8) + LSB; //synthesize into 16-bit integer } return ~ack; //ack==0 indicates an operation response, so the return value is its inverse value }
Previous article:51 MCU Introduction - IIC (I2C) Bus
Next article:51 MCU Introduction - SPI Bus
Recommended ReadingLatest update time:2024-11-15 09:12
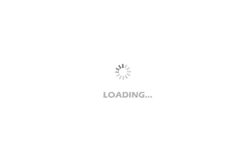
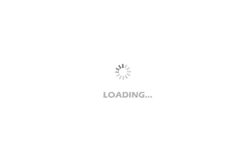
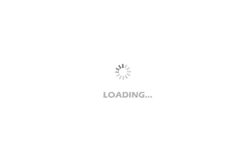
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Single-chip microcomputer technology and application - electronic circuit design, simulation and production (edited by Zhou Runjing)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Detailed explanation of intelligent car body perception system
- How to solve the problem that the servo drive is not enabled
- Why does the servo drive not power on?
- What point should I connect to when the servo is turned on?
- How to turn on the internal enable of Panasonic servo drive?
- What is the rigidity setting of Panasonic servo drive?
- How to change the inertia ratio of Panasonic servo drive
- What is the inertia ratio of the servo motor?
- Is it better for the motor to have a large or small moment of inertia?
- What is the difference between low inertia and high inertia of servo motors?
- MSP432UART Test
- ESP32, only one timer is turned on, the power consumption is very high, what should I do
- [TI recommended course] #Power Tips — Power management design tips series#
- STM32 allows pyb, uos, utime, machine and onewire modules to be configurable
- FAQ Sharing: Frequently Asked Questions about TI Wireless Product RF Hardware
- 【TGF4042 Signal Generator】+ Noise Test
- Authoritative sharing of Lingdong Microelectronics MM32 MCU information
- ESP8266WiFi module (IoT switch) test (AP mode)
- CAN communication design, please help
- How to make component packages with multiple pins connected internally in Allegro