UART, I2C and SPI are the three most commonly used communication protocols in microcontroller systems.
1. Introduction
SPI is a high-speed, full-duplex, synchronous communication bus. The standard SPI uses only 4 pins and is commonly used for communication between microcontrollers and EEPROM, FLASH, real-time clocks, digital signal processors and other devices. The SPI communication principle is simpler than I2C. It is mainly a master-slave communication mode. This mode usually has only one host and one or more slaves. The standard SPI has 4 wires, namely SSEL (chip select, also written as SCS), SCLK (clock, also written as SCK), MOSI (master output slave input Master
Output/Slave Input) and MISO (Master Input/Slave Output).
SSEL: Slave chip select enable signal. If the slave device is low level enabled, when this pin is pulled low, the slave device will be selected, and the host will communicate with the selected slave.
SCLK: Clock signal, generated by the host, somewhat similar to the SCL of I2C communication.
MOSI: The channel through which the host sends instructions or data to the slave. MISO: The channel through which the host reads the status or data of the slave.
2. Working mode
The host of SPI communication is also our microcontroller. There are four modes in the process of reading and writing data timing;
CPOL: Clock Polarity, which is the polarity of the clock. The entire communication process is divided into idle time and communication time. If the idle state of SCLK before and after data transmission is high, then CPOL=1, if the idle state SCLK is low, then CPOL=0.
CPHA: Clock Phase, which is the phase of the clock.
#include typedef unsigned char uchar; sbit DS1302_CE = P1^7; sbit DS1302_CK = P3^5; sbit DS1302_IO = P3^4; struct sTime //Date and time structure definition { unsigned int year; //year unsigned char mon; //month unsigned char day; //day unsigned char hour; //hour unsigned char min; // points unsigned char sec; //seconds unsigned char week; //week }; /* Send a byte to the DS1302 communication bus */ void DS1302ByteWrite(uchar dat) { uchar mask; for (mask = 0x01; mask != 0; mask <<= 1) // Low bit first, shift out one by one { if ((mask & dat) != 0) // first output the data bit { DS1302_IO = 1; } else { DS1302_IO = 0; } DS1302_CK = 1; //Then pull the clock high DS1302_CK = 0; //Pull the clock down again to complete a bit operation } DS1302_IO = 1; //Finally make sure to release the IO pin } /* Read a byte from the DS1302 communication bus */ uchar DS1302ByteRead() { uchar mask; uchar dat = 0; for (mask = 0x01; mask != 0; mask <<= 1) //low bit first, read bit by bit { if (DS1302_IO != 0) //First read the current IO pin and set the corresponding bit in dat { dat |= mask; } DS1302_CK = 1; //Then pull the clock high DS1302_CK = 0; //Pull the clock down again to complete a bit operation } return dat; //Finally return the read byte data } /* Use a single write operation to write a byte to a register, reg-register address, dat-byte to be written*/ void DS1302SingleWrite(uchar reg, uchar dat) { DS1302_CE = 1; // Enable chip select signal DS1302ByteWrite((reg << 1) | 0x80); //Send write register instruction DS1302ByteWrite(dat); //Write byte data DS1302_CE = 0; //Disable chip select signal } /* Use a single read operation to read a byte from a register, reg-register address, return value-read byte*/ uchar DS1302SingleRead(uchar reg) { uchar dat; DS1302_CE = 1; // Enable chip select signal DS1302ByteWrite((reg << 1) | 0x81); //Send the read register instruction dat = DS1302ByteRead(); //Read byte data DS1302_CE = 0; //Disable chip select signal return dat; } /* Use burst mode to continuously write 8 register data, dat-data pointer to be written*/ void DS1302BurstWrite(uchar *dat) { uchar i; DS1302_CE = 1; DS1302ByteWrite(0xBE); //Send burst write register instruction for (i = 0; i < 8; i++) //Continuously write 8 bytes of data { DS1302ByteWrite(dat[i]); } DS1302_CE = 0; } /* Use burst mode to continuously read the data of 8 registers, dat-read data receiving pointer*/ void DS1302BurstRead(uchar *dat) { uchar i; DS1302_CE = 1; DS1302ByteWrite(0xBF); //Send burst read register instruction for (i = 0; i < 8; i++) //Read 8 bytes continuously { dat[i] = DS1302ByteRead(); } DS1302_CE = 0; } /* Get real-time time, that is, read the current time of DS1302 and convert it into time structure format*/ void GetRealTime(struct sTime *time) { uchar buf[8]; DS1302BurstRead(buf); time->year = buf[6] + 0x2000; time->mon = buf[4]; time->day = buf[3]; time->hour = buf[2]; time->min = buf[1]; time->sec = buf[0]; time->week = buf[5]; } /* Set the real time, convert the set time in the time structure format into an array and write it into DS1302*/ void SetRealTime(struct sTime *time) { uchar buf[8]; buf[7] = 0; buf[6] = time->year; buf[5] = time->week; buf[4] = time->mon; buf[3] = time->day; buf[2] = time->hour; buf[1] = time->min; buf[0] = time->sec; DS1302BurstWrite(buf); } /* DS1302 initialization, if power failure occurs, reset the initial time*/ void InitDS1302() { uchar dat; struct sTime code InitTime[] = //Tuesday, May 18, 2016, 9:00:00 { 0x2016, 0x05, 0x18, 0x09, 0x00, 0x00, 0x02 }; DS1302_CE = 0; //Initialize DS1302 communication pin DS1302_CK = 0; dat = DS1302SingleRead(0); //Read the seconds register if ((dat & 0x80) != 0) //Judge whether DS1302 has stopped by the value of the highest bit CH of the seconds register { DS1302SingleWrite(7, 0x00); //Remove write protection to allow data to be written SetRealTime(&InitTime); //Set DS1302 to the default initial time } }
Previous article:51 MCU Introduction - DS18B20 Temperature Sensor
Next article:51 MCU Introduction - UART Serial Port
Recommended ReadingLatest update time:2024-11-24 19:20
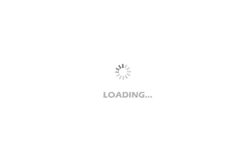
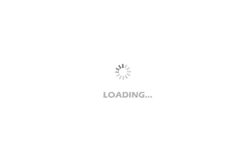
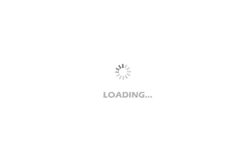
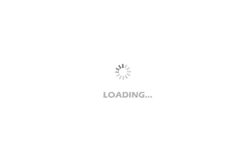
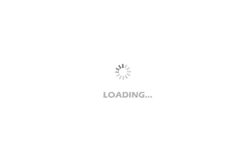
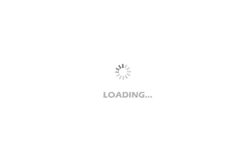
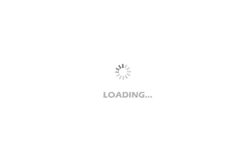
Recommended posts
- Several questions about MmwaveStudio
- Question1:WhataretheparametersfortheSensorconfigurationoptioninMmwaveStudioandwhatareitsmeanings? Thereissomeconfusionwhenconfiguringthemmwavestudiosensorconfigurationoptions.Thecompletesensorconfigurationtabissho
-
qwqwqw2088
Analogue and Mixed Signal
- C6678 on-chip storage space allocation mechanism
- ThestackspaceonembeddeddevicessuchasDSPisattheKblevel.Whendefininganarrayorapplyingforspacewithinafunction,youcannotdefineandapplyforitdirectlyasinLinux.Youmusteitherdefineitgloballyorpointtoawell-allo
-
Jacktang
Microcontroller MCU
- A question about understanding electric current again
- Ionceansweredaquestionfromanetizen:"Ifaninsulatingobjectwithastaticelectricitycoremovesathighspeed,eitherinastraightlineorinacircle,willamagneticfieldbeformedaroundit?" Myansweratthetimewas:"Itshouldbe
-
bigbat
Power technology
- DIY Wireless Charging Cool Light
- Whileonvacationathome,IusedoldcomponentsandmaterialstoDIYasimpleandcoollampwithwirelesscharging. Materialsused: Fakefruitearphoneswirelesschargingbox 3VLEDLightBoard 20ohmresistor Toggle
-
dcexpert
MicroPython Open Source section
- Selection Tips | How to choose a suitable power amplifier for the experiment? What parameters should be paid attention to?
- SelectionTips|Howtochooseasuitablepoweramplifierfortheexperiment?Whatparametersshouldbepaidattentionto? Whatindicatorparametersshouldwepayattentionto? Didn'tsayanything Haha~Sincethevideoisshort,Imay
-
aigtekatdz
Test/Measurement
- How to prevent the phase-shifted full-bridge DCDC from crashing when starting or increasing or decreasing power?
- Purpose:Duetomanufacturingprocessandotherreasons,wewanttoreducethepossibilityofpowersupplycrashingtozerobytestingandaddingprotection.Evenifthepowersupplyitselfhasabnormalcomponents,itwillnotcrash.Currently,only
-
DKandDK
Switching Power Supply Study Group
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Fundamentals and Applications of Single Chip Microcomputers (Edited by Zhang Liguang and Chen Zhongxiao)
-
Single chip microcomputer control technology (Li Shuping, Wang Yan, Zhu Yu, Zhang Xiaoyun)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- Xsens Sirius Series Inertial Sensors Enable 3D Inertial Navigation in Harsh Environments
- Infineon's Automotive Landscape: From Hardware to Systems
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Initial debugging of NXP beacon camera (avoid detours)
- [HPM-DIY] openmv for hpm6750 progress 1
- Indian engineer sues SpaceX, alleging discrimination at work
- Urgent! The device just came out yesterday, and today my teammates ran away!
- Notice of the State Council on Issuing Several Policies to Encourage the Development of the Software Industry and the Integrated Circuit Industry
- "Notifying employees of slacking off at work", Gome responds
- Uncover the real reason behind the explosive development of TWS headphones
- Introduction to the new Mutianyu scenic area in Huairou, Beijing (Photos)
- Byte alignment for 32-bit embedded systems
- Layman asks questions about ZigBee