Delay is a function that is often used in assembly. That is, the MCU does nothing but delays for a period of time. The MCU itself has timers and counters, which can be used to realize delay. However, a programmer naturally hopes that the function to be realized is easier to control. We use code and program to realize delay, that is, software delay. The specific method is: through A, H:X increase and decrease instructions, no operation instructions nop and brn and corresponding transfer instructions, and then use the loop structure to realize the delay function. Since it is a delay, it is best to know how long the delay is. What we know is that the MCU bus clock frequency is 4MHz, so a bus cycle takes 0.25us. In this way, as long as we know the bus cycle occupied by each instruction, we can calculate how long our program runs. The same is true for delay. Of course, these require mathematical knowledge. Don't worry, calculus is not needed. As long as you are patient, it is not difficult to accurately calculate the delay time.
Example: Design a delay subroutine with a delay of 10ms, given that the MCU bus clock frequency is 4MHz.
Analysis: Since the bus clock frequency is 4MHz, a bus cycle takes 0.25us, and a 10ms delay requires the execution of instructions equivalent to 40,000 bus cycles. We can first design a subroutine Re_cycle to achieve a smaller delay, and then call the subroutine multiple times to achieve a longer delay. The code is as follows:
org $0070
num ds.b 1
count1 ds.b 1
org $1860
re_cycle: ;4+7*70+6=500T=125us
mov #70T,num ;4T
dbnz num,* ;7T
rts ;6T
delay_10ms: ;[4+78*(5+7+500)]+4+7*7+6=39999T
mov #78T,count1 ;4T
re_call:
bsr re_cycle ;5T
dbnz count1,re_call ;7T
mov #07T,count1 ;4T
dbnz count1,* ;7T
rts ;6T
main:
bsr delay_10ms ;5~6T
again:
nop
jmp again
org $fffe
dc.w main
The time taken by each instruction has been marked. What is needed is delicate design and precise calculation. For example, the design of the Re_cycle subroutine takes exactly 500T for three instructions. Here we need to explain the DBNZ instruction. The function it implements is to compare the number in the previous variable with 0 by decrementing it by 1. If they are not equal, it will transfer to the following address and execute it. If they are equal, it will end the instruction (that is, if the decrement is not 0, the instruction will be transferred). Here we must ask what * represents. It represents the address of the instruction where it is located. The dbnz num,* instruction can be interpreted as num decrementing by 1 and not equal to 0, then it will come back and execute the statement again, and it will end when num decremented by 1 is equal to 0. From the comments, it can be seen that the total time taken by the delay_10ms subroutine to complete is 39999T, plus the call to the delay_10ms subroutine in the main program takes 5~6T. In this way, if delay_10ms is not called once, a delay of about 10ms for 40004~40005 bus cycles can be achieved. Of course, if the design is good enough, it can be even more precise, the closer to 40,000 T the better.
The above implements a delay of 10ms. What if we want to implement 100ms, 500ms, 1ms, and 0.1ms? The same method relies on sophisticated design and precise calculation. The following are subroutines for delays of 1ms and 500ms:
org $0070
num ds.b 1
count1 ds.b 1
count2 ds.b 1
org $1860
re_cycle: ;4+7*70+6=500T=125us
mov #70T,num
dbnz num,*
rts
delay_1ms: ;[4+(5+7+500)*8+6]=4106T
mov #08t,count
re_call:
bsr re_cycle
dbnz count,re_call
rts
delay_10ms: ;39999T
mov #78T,count1
re_call:
bsr re_cycle
dbnz count1,re_call
mov #07T,count1
dbnz count1,*
rts
delay_500ms: ;The idea is to repeat delay_10ms 50 times, so there will definitely be some inaccuracies, please forgive me.
mov #50T,count2
re:
bsr delay_10ms
dbnz count2,re
rts
main:
bsr delay_1ms
bsr delay_10ms
bsr delay_500ms
again:
nop
jmp again
org $fffe
dc.w main
Previous article:Freescale MC9S08AW60 Assembly Learning Notes (V)
Next article:Freescale MC9S08AW60 Assembly Learning Notes (VIII)
Recommended ReadingLatest update time:2024-11-23 09:27
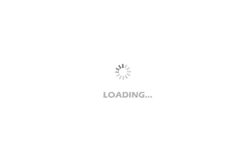
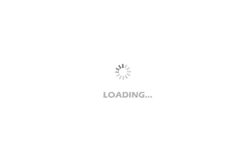
- Popular Resources
- Popular amplifiers
-
Practical Development of Automotive FlexRay Bus System (Written by Wu Baoxin, Guo Yonghong, Cao Yi, Zhao Dongyang, etc.)
-
Automotive Electronics KEA Series Microcontrollers——Based on ARM Cortex-M0+ Core
-
Automotive integrated circuits and their applications
-
ADS2008 RF Circuit Design and Simulation Examples (Edited by Xu Xingfu)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- PIC16C712 functions, features, and application areas
- "DSP Principles and C Programming Development Technology" related sample files
- Desktop Assistant Based on GD32F350——Supplementary Video
- ARM CORTEX-M3 core architecture understanding summary
- Introduction to TI's three-phase Vienna PFC solution
- A brief introduction to the Cache workflow of the M7 core, a summary of half a year of experience - transferred to Anfulai
- OBD output data
- EEWORLD University Hall----Live Replay: CC13X2/CC26X2 - TI SimpleLink Platform New Generation Wireless Product Solutions
- FPGA PLL Loss of Lock
- Altium Designer 17 complete set of novice zero-based entry PCB video training tutorial sharing