{
SetTimeBcdByte(6);
}
else if (irdCode[2] == 0x42)
{
SetTimeBcdByte(7);
}
else if (irdCode[2] == 0x52)
{
SetTimeBcdByte(8);
}
else if (irdCode[2] == 0x4A)
{
SetTimeBcdByte(9);
}
else
{
}
}
/*T0 interrupt service*/
void Timer0_ISP() interrupt 1
{
static unsigned char counter = 0;
TH0 = thr0;
TL0 = tlr0; //1ms
counter++;
KeyScan(); //Key scan
if (counter >= 200)
{
counter = 0;
flag200ms = 1;//200ms
}
}
#ifndef _MAIN_H_
#define _MAIN_H_
sbit ADDR0 = P1^0;
sbit ADDR1 = P1^1;
sbit ADDR2 = P1^2;
sbit ADDR3 = P1^3;
sbit ENLED = P1^4;
#endif //_MAIN_H_
/**
************************************************************************
* @file : Lcd1602.c
* @author : xr
* @date : April 21, 2014 22:23:12
* @version : V1.2.3
* @brief : LCD1602 bottom-level driver microcontroller STC89C52RC MCU crystal oscillator 11.0592MHZ
************************************************************************
*/
#include //LCD1602 sbit LCD1602_RS = P1^0; sbit LCD1602_RW = P1^1; sbit LCD1602_EN = P1^5; #define LCD1602_DB P0 /*LCD1602 busy waiting*/ void LCD1602Wait() { unsigned char sta; //Read LCD1602 status word /*P0 ports must be pulled high before reading the LCD status word*/ LCD1602_DB = 0xFF; LCD1602_RS = 0; LCD1602_RW = 1; LCD1602_EN = 0; do { LCD1602_EN = 1; sta = LCD1602_DB; //Read status word LCD1602_EN = 0; } while (sta & 0x80); //Check if the highest bit is 1, 1 is busy, 0 is idle } /*LCD1602 write command*/ void LCD1602WriteCmd(unsigned char cmd) { //Before reading and writing, the LCD needs to be busy waiting LCD1602Wait(); LCD1602_RS = 0; LCD1602_RW = 0; LCD1602_EN = 0; LCD1602_DB = cmd; LCD1602_EN = 1; //High pulse LCD1602_EN = 0; // Turn off LCD output } /*LCD1602 write data*/ void LCD1602WriteData(unsigned char dat) { LCD1602Wait(); LCD1602_RS = 1; LCD1602_RW = 0; LCD1602_EN = 0; LCD1602_DB = dat; // input data LCD1602_EN = 1; //High pulse LCD1602_EN = 0; // Turn off LCD output } /*LCD initialization*/ void InitLCD1602() { LCD1602WriteCmd(0x38); //Write command 38H LCD1602WriteCmd(0x0C); //Turn on the display without displaying the cursor LCD1602WriteCmd(0x06); //Character pointer++ and cursor++ when writing characters LCD1602WriteCmd(0x01); //Clear the screen } /*Display str at the coordinate (x, y) position of LCD1602*/ void LcdShowStr(unsigned char x, unsigned char y, unsigned char * str) { unsigned char addr; if (y == 0) { addr = 0x00 + x; //x position display of the first line } else { addr = 0x40 + x; //The position of x in the second line is displayed } LCD1602WriteCmd(addr + 0x80); while (*str != '') { LCD1602WriteData(*str++); } } /*Set the cursor position to (x, y)*/ void LcdSetCoursor(unsigned char x, unsigned char y) { unsigned char addr; if (y == 0) { addr = 0x00 + x; } else { addr = 0x40 + x; } LCD1602WriteCmd(addr | 0x80); //Write cursor blinking address } /*Turn on cursor display*/ void LcdOpenCoursor() { LCD1602WriteCmd(0x0F); //Display cursor disease makes the cursor flash } /*Turn off cursor display*/ void LcdCloseCoursor() { LCD1602WriteCmd(0x0C); //Turn on the display but don't display the cursor } /** ************************************************************************ * @file : Ds1302.c * @author : xr * @date : April 21, 2014 22:23:12 * @version : V1.2.3 * @brief : DS1302 bottom-level driver microcontroller STC89C52RC MCU crystal oscillator 11.0592MHZ ************************************************************************ */ #include //DS1302 sbit DS1302_SIO = P3^4; //Data IO sbit DS1302_SCK = P3^5; //clock line sbit DS1302_CE = P1^7; //Chip select enable /*When using external data types, you must declare them again in this file*/ struct Time { unsigned char year; //The year is stored in the DS1302 in the lower two digits, so here we use unsigned char type unsigned char month; unsigned char day; unsigned char hour; unsigned char min; unsigned char sec; unsigned char week; }; /*Send a byte of data to SIO*/ void DS1302WriteByte(unsigned char byte) { unsigned char mask = 0x01; //low bit first, send bit by bit /*When initializing DS1302, pull SIO and SCK pins low and idle*/ for (mask = 0x01; mask != 0; mask <<= 1) { if ((byte & mask) != 0) // DS1302 samples and latches data on the rising edge { DS1302_NOT = 1; } else { DS1302_NOT = 0; } DS1302_SCK = 1; DS1302_SCK = 0; // falling edge host outputs data } //After the host finishes sending data, it needs to release the SIO data line DS1302_NOT = 1; } /*Read data on the SIO data line*/ unsigned char DS1302ReadByte() { unsigned char mask = 0x01; // low bit first, receive bit by bit unsigned char byte = 0; for (mask = 0x01; mask != 0; mask <<= 1) { if (DS1302_SIO != 0) //Rising edge host performs data sampling and latching { byte |= mask; //corresponding position 1 } else { byte &= (~mask); // Clear the corresponding bit } DS1302_SCK = 1; DS1302_SCK = 0; //DS1302 outputs data on the falling edge } return (byte); } /*Write a single byte of data to the reg address register of DS1302*/ void DS1302WriteSingleByte(unsigned char reg, unsigned char dat) { /*First open the DS1302 chip select terminal, then write the register address reg, and then write the data dat*/ DS1302_CE = 1; DS1302WriteByte((reg << 1) | 0x80); //Write register address, reg<<1 leaves out the read/write direction bit, the high bit of reg is 1, the second high bit is 0 DS1302WriteByte(dat); //Write data DS1302_CE = 0; DS1302_SIO = 0; //Because there is no SIO port on the board and no pull-up resistor is added, the open-drain output is unstable. Set SIO=0, and the MCU IO port will output a stable 0 state } /*Read a byte of data from the reg address register of DS1302*/ unsigned char DS1302ReadSingleByte(unsigned char reg) { unsigned char byte = 0; DS1302_CE = 1; DS1302WriteByte((reg << 1) | 0x81); //Write register address reg and select read in read/write direction byte = DS1302ReadByte(); DS1302_CE = 0; DS1302_SIO = 0; //SIO output stable 0 state return (byte); } /*Burst mode writes eight bytes to eight registers of DS1302*/ void DS1302BurstWrite(unsigned char * date) { unsigned char i = 0; DS1302_CE = 1; DS1302WriteByte(0xBE); //Write burst mode command (Burst mode) for (i = 0; i < 8; i++) { DS1302WriteByte(date[i]); } DS1302_CE = 0; DS1302_NOT = 0; } /*Burst mode reads eight bytes of data from the DS1302 register continuously*/ void DS1302BurstRead(unsigned char * date) { unsigned char i = 0; DS1302_CE = 1; //Chip select enable DS1302WriteByte(0xBF); //Burst read instruction for (i = 0; i < 8; i++) { date[i] = DS1302ReadByte(); } DS1302_CE = 0; DS1302_NOT = 0; } /*Get the current time from DS1302*/ void GetTimeFromDS1302(struct Time * time) { unsigned char buff[8]; //Save the data in eight registers DS1302BurstRead(buff); //Read the data in the DS1302 register into the buff array time->year = buff[6]; // Take out the bcd code in the year register and store it in the structure time->year time->month = buff[4]; time->week = buff[5]; time->day = buff[3]; time->hour = buff[2]; time->min = buff[1]; time->sec = buff[0]; } /*Set the time, set the current modified time value to DS1302*/ void SetTimeToDS1302(struct Time * time) { unsigned char buff[8]; //Save the value of the current modified time structure member buff[0] = time->sec; //Store the value in the seconds register into buff[0 buff[1] = time->min; buff[2] = time->hour; buff[3] = time->day; buff[4] = time->month; buff[5] = time->week; buff[6] = time->year; buff[7] = 0x00; //WP write protection register DS1302BurstWrite(buff); //Write the value in buff to DS1302 in Burst mode } /* Initialize DS1302 */ void InitDS1302() { struct Time code InitTime[] = {0x14, 0x04, 0x22, 0x23, 0x59, 0x59, 0x02}; //April 22, 2014 23:59:59 unsigned char psec; //Detect the stop bit CH of DS1302 /*Initialize the communication pins of DS1302*/ DS1302_SCK = 0; DS1302_CE = 0; psec = DS1302ReadSingleByte(0x00); //0x00<<1 | 0x81=0x81, read the second register if ((psec & 0x80) != 0) //highest bit CH=1, clock stops { DS1302WriteSingleByte(7, 0x00); //Remove write protection SetTimeToDS1302(InitTime); //Set the initial time to DS1302 } } /** ************************************************************************** * @file : Infrared.c * @author : xr * @date : April 23, 2014 22:01:12 * @version : V1.2.3 * @brief : Infrared communication decoding-NEC protocol decoding ************************************************************************** */ #include //Infrared communication pin sbit IRD = P3^3; // infrared communication pin, external interrupt 1 pin bit flagIrd = 0; // Flag bit for infrared data code value reception completion unsigned char irdCode[4]; //Save the four-byte data code decoded by the NEC protocol (user code + user inverse code, key code + key code inverse code) /*Infrared communication - Timer T1 counts and obtains the time for infrared receiving data judgment*/ void ConfigIrdByTimer1() { TMOD &= 0x0F; // Clear T1 control bit TMOD |= 0x10; //Use T1 mode 1, 16-bit configurable mode TH1 = 0; TL1 = 0; //No infrared signal at the beginning, clear T1 count TR1 = 0; //Close T1 count at the beginning ET1 = 0; //Disable T1 interrupt, only use T1 counting function /*External interrupt 1 placement*/ IE1 = 0; // External interrupt 1 flag cleared IT1 = 1; //Set external interrupt 1 to falling edge trigger EX1 = 1; // Enable external interrupt EA = 1; // Enable general interrupt } /*Get the IRD pin, which is the infrared pin high level T1 count value*/ unsigned int GetHighTimers() { IRD = 1; //Before detecting the infrared pin, pull the IRD pin high to release it TH1 = 0; TL1 = 0; // Clear the last T1 count value first TR1 = 1; //Start T1 counting while (IRD) { //High level T1 counts if (TH1 > 0x40) //0x40 * 256 * (12/11059200) * 1000 = 17.7ms { break; //When the time > 17.7ms, we consider it a bit error and force exit } } TR1 = 0; //Turn off T1 counting return (TH1 * 256 + TL1); //Return the count value of T1 when the IRD pin is high } /*Get low level carrier time*/ unsigned int GetLowTimers() { IRD = 1; // Before detecting the infrared pin, release the IRD pin first TH1 = 0; TL1 = 0; // Clear T1 count value TR1 = 1; //Start T1 counting while (!IRD) { //Timeout judgment if (TH1 > 0x40) //Exit if it exceeds 17.7ms { break; } } TR1 = 0; // Turn off T1 counting
Previous article:Design of emergency power inverter circuit using single chip microcomputer SPWM
Next article:Circuit structure of P0 port of 51 single chip microcomputer
Recommended ReadingLatest update time:2024-11-16 19:46
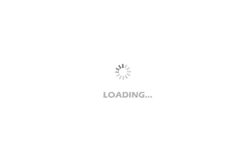
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Signal Integrity and Power Integrity Analysis (Eric Bogatin)
-
Traffic Light Control System Based on Fuzzy Control (Single-Chip Microcomputer Implementation)
-
Principles and Applications of Single Chip Microcomputers (Second Edition) (Wanlong)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- The clock signal is followed by an inverter.
- EEWORLD University Hall----Live Replay: TI Wireless Product Update: Wi-Sun Standard Helps Smart City Construction
- TI automotive solution puzzle, do you dare to challenge it?
- [Fifth Batch of Shortlist] GigaDevice GD32L233 Review Event
- Mobile station update development board, welcome to borrow it!!
- 3. "Wanli" Raspberry Pi car - Python learning (timing task)
- This is a camera development board, but where is the camera?
- Sharing the principle of the adapter solution for charging while listening to music
- Make announces its return
- The mysterious EMC, how did it come about?