Making a digital voltmeter (2 credit hours)
1. Experimental purpose:
1. Understand the working principle and communication protocol of I2C serial bus
2. Understand the interface design of ADC0804, DAC0832, PCF8591 and AT89S51
3. Master the programming methods of ADC0804, DAC0832, and PCF8591
4. Be able to skillfully use the digital-to-analog conversion module
2. Experimental requirements:
1. Digital voltmeter: PCF8591 chip is used in AT89C52 system to measure DC voltage in the range of 0-5V and display the voltage value on a 2-digit digital tube.
3. Experimental equipment: (PROTEUS component list)
4. Experimental report:
1. Describe the experimental procedure (with screenshots of important steps).
2. Give the circuit diagram of the single-chip microcomputer system designed in PROTEUS
3. Draw a program flow chart
4. Give the source program written in KEIL.
5. Describe the experimental phenomena of simulating the program in Proteus
5. Experimental Summary
The microcontroller source program is as follows:
/**********************BST-M51 experimental development board routine************************
* Platform: BST-M51 + Keil U4 + STC89C52
* Name: AD serial port reading experiment
* Company: Shenzhen Yabo Software Development Co., Ltd.
* Date: 2015-6
* Crystal oscillator: 11.0592MHZ
******************************************************************/
#include #include #define AddWr 0x90 //PCF8591 address // Variable definitions unsigned char AD_CHANNEL=0; unsigned char D[32]; unsigned char code table[10]={0xC0,0x79,0x24,0x30,0x19,0x12,0x02,0x78,0x00,0x10}; //numeric table of common anode digital tubes 0~9 unsigned int data dis[3]={0x00,0x00,0x00}; //3-element array used to calculate and store output voltage sbit scl=P2^0; //I2C clock sbit sda=P2^1; //I2C data bit ack; /*Response flag*/ sbit C1=P2^6; //digital tube bit selection sbit C2=P2^7; //digital tube bit selection sbit Dp=P2^5; //decimal point unsigned char date; /******************************************************************* Start bus function Function prototype: void Start_I2c(); Function: Start the I2C bus, that is, send the I2C start condition. ********************************************************************/ void Start_I2c() { sda=1; /*Send the data signal of the start condition*/ _nop_(); scl=1; _nop_(); /*Start condition establishment time is greater than 4.7us, delay*/ _nop_(); _nop_(); _nop_(); _nop_(); sda=0; /*Send start signal*/ _nop_(); /* Start condition lock time is greater than 4μs*/ _nop_(); _nop_(); _nop_(); _nop_(); scl=0; /*Clamp the I2C bus and prepare to send or receive data*/ _nop_(); _nop_(); } /******************************************************************* End bus function Function prototype: void Stop_I2c(); Function: End the I2C bus, that is, send the I2C end condition. ********************************************************************/ void Stop_I2c() { sda=0; /*Send data signal of end condition*/ _nop_(); /*Send the clock signal of the end condition*/ scl=1; /*End condition establishment time is greater than 4μs*/ _nop_(); _nop_(); _nop_(); _nop_(); _nop_(); sda=1; /*Send I2C bus end signal*/ _nop_(); _nop_(); _nop_(); _nop_(); } /******************************************************************* Byte data sending function Function prototype: void I2C_SendByte(UCHAR c); Function: Send data c, which can be an address or data, wait for a response after sending, and This status bit operates. (No response or non-response makes ack=0) If data is sent normally, ack=1; ack=0 means the controlled device has no response or is damaged. ********************************************************************/ void I2C_SendByte(unsigned char c) { unsigned char i; for(i=0;i<8;i++) /*The length of the data to be transmitted is 8 bits*/ { if((c< else sda=0; _nop_(); scl=1; /*Set the clock line high to notify the controlled device to start receiving data bits*/ _nop_(); _nop_(); /*Ensure that the clock high level period is greater than 4μs*/ _nop_(); _nop_(); _nop_(); scl=0; } _nop_(); _nop_(); sda=1; /*Release the data line after sending 8 bits and prepare to receive the response bit*/ _nop_(); _nop_(); scl=1; _nop_(); _nop_(); _nop_(); if(sda==1)ack=0; else ack=1; /*Judge whether the response signal is received*/ scl=0; _nop_(); _nop_(); } /******************************************************************* Byte data receiving function Function prototype: UCHAR I2C_RcvByte(); Function: Used to receive data from the device and determine bus errors (no response signal is sent). After sending, please use the response function to respond to the slave. ********************************************************************/ unsigned char I2C_RcvByte() { unsigned char retc=0,i; sda=1; /*Set the data line to input mode*/ for(i=0;i<8;i++) { _nop_(); scl=0; /*Set the clock line low and prepare to receive data bits*/ _nop_(); _nop_(); /*Clock low level period is greater than 4.7μs*/ _nop_(); _nop_(); _nop_(); scl=1; /*Set the clock line high to make the data on the data line valid*/ _nop_(); _nop_(); retc=retc<<1; if(sda==1)retc=retc+1; /*Read data bit, and put the received data bit into retc*/ _nop_(); _nop_(); } scl=0; _nop_(); _nop_(); return(retc); } /******************************************************************** Response subfunction Function prototype: void Ack_I2c(bit a); Function: The master controller responds to the signal (can be a response or non-response signal, determined by bit parameter a) ********************************************************************/ void Ack_I2c(bit a) { if(a==0)sda=0; /*Send a response or non-response signal here*/ else sda=1; /*0 is a response, 1 is a non-response signal*/ _nop_(); _nop_(); _nop_(); scl=1; _nop_(); _nop_(); /*Clock low level period is greater than 4μs*/ _nop_(); _nop_(); _nop_(); scl=0; /*Clear the clock line and hold the I2C bus to continue receiving*/ _nop_(); _nop_(); } /************************************************************ * Function name: Pcf8591_DaConversion * Function: Output analog value of PCF8591 * Input: addr (device address), channel (conversion channel), value (conversion value) * Output: None ******************* *****************************************/ bit Pcf8591_DaConversion(unsigned char addr,unsigned char channel, unsigned char Val) { Start_I2c(); //Start the bus I2C_SendByte(addr); //Send device address if(ack==0)return(0); I2C_SendByte(0x40|channel); //Send control byte if(ack==0)return(0); I2C_SendByte(Val); //Send DAC value if(ack==0)return(0); Stop_I2c(); //End the bus return(1); } /************************************************************ * Function name: Pcf8591_SendByte * Function: Write a control command * Input: addr (device address), channel (conversion channel) * Output: None ************************************************************/ bit PCF8591_SendByte(unsigned char addr,unsigned char channel) { Start_I2c(); //Start the bus I2C_SendByte(addr); //Send device address if(ack==0)return(0); I2C_SendByte(0x40|channel); //Send control byte if(ack==0)return(0); Stop_I2c(); //End the bus return(1); } /************************************************************ * Function name: PCF8591_RcvByte * Function: Read a conversion value * Input : * Output: dat ************************************************************/ unsigned char PCF8591_RcvByte(unsigned char addr) { unsigned char dat; Start_I2c(); //Start the bus I2C_SendByte(addr+1); //Send device address if(ack==0)return(0); dat=I2C_RcvByte(); //Read data 0 Ack_I2c(1); //Send non-acknowledge signal Stop_I2c(); //End the bus return(that); } /*------------------------------------------------ Serial port initialization function ------------------------------------------------*/ void init_com(void) { EA=1; //Open the general interrupt ES=1; //Enable serial port interrupt ET1=1; //Enable timer T1 interrupt TMOD=0x20; //Timer T1, generates baud rate in mode 2 interrupt PCON=0x00; //SMOD=0 SCON=0x50; // Mode 1 controlled by timer TH1=0xfd; //Set the baud rate to 9600 TL1=0xfd; TR1=1; //Start timer T1 running control bit } /*------------------------------------------------ Delay function ------------------------------------------------*/ void delay(unsigned char i) { unsigned char j,k; for(j=i;j>0;j--) for(k=125;k>0;k--); } /*------------------------------------------------ Convert the read value into characters one by one and display them on the serial port ------------------------------------------------*/ void To_ascii(unsigned char num) { SBUF=num/100+'0'; delay(200); SBUF=num/10%10+'0'; delay(200); SBUF=num%10+'0'; delay(200); } /*------------------------------------------------ Main function ------------------------------------------------*/ main() { init_com(); while(1) { /********The following AD-DA processing*************/ PCF8591_SendByte(AddWr,0); //Start conversion D[0]=PCF8591_RcvByte(AddWr); //Read the converted digital signal, ADC0 analog-to-digital conversion 1 photoresistor /********The following will send the AD value through the serial port*************/
Previous article:51 single chip simple electronic scale program
Next article:Electronic password lock based on AT89S52 single chip microcomputer
Recommended ReadingLatest update time:2024-11-15 15:12
![[51 MCU Quick Start Guide] 9: Power saving mode (low power consumption)](https://6.eewimg.cn/news/statics/images/loading.gif)
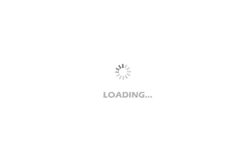
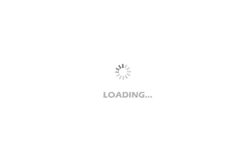
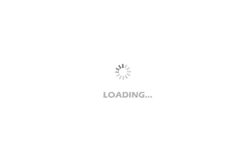
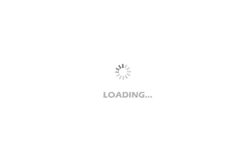
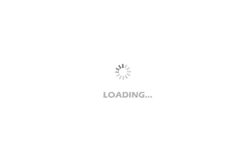
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
- EEWORLD University Hall----Design considerations for a robust interface between J6 and vehicle displays via FPD-Link
- [Analog Electronics Course Selection Test] + User Experience
- [Sipeed LicheeRV 86 Panel Review] IX. lvgl Reuse
- PWM pulses generated by ecap
- 【Goodbye 2021, Hello 2022】Busy and fulfilling
- Derivation of the voltage transfer function of a circuit containing capacitors T(S) = Vo(S)/Vi(S)
- Answer the question to win a prize | Using GaN technology to meet power adapter design challenges
- Question about the schematic diagram of switching power supply
- Application of NGI DC Power Supply in Current Sensor Testing
- It is said that Lichuang's server was hacked