As shown in the figure below, taking Windows as an example, if we plug in a USB without a USB device driver, you will be prompted to install the driver
1 Why does a prompt message appear as soon as the device is plugged in?
This is because Windows comes with a USB bus driver.
2 The USB bus driver is responsible for:
Identify USB devices; find and install the corresponding driver for USB devices; provide USB read and write functions.
The default address (number) of a newly connected USB device is 0. Before a new number is assigned, the PC host uses address 0 to communicate with it.
Then the USB bus driver will assign it an address (number)
When a PC wants to access a USB device on the USB bus, the command it issues contains the corresponding address (number)
USB is a master-slave structure. The host is called Host and the slave is called Device. All USB transmissions are initiated by the USB host; USB devices do not have the ability to "actively" notify the USB host.
For example: A USB mouse generates data immediately when it is slid, but it does not have the ability to read the data through an OC machine and can only passively wait for the PC to read it.
The hardware principle of USB hot-swappable
A 15K ohm pull-down resistor is connected to the ground on the D+ and D- of each downstream port of the USB hub. In this way, when the port of the hub is left floating, it is pulled to a low level by these two pull-down resistors.
On the USB device side, a 1.5k ohm pull-up resistor is connected to D+ or D-. For full-speed and high-speed devices, the pull-up resistor is connected to D+; for low-speed devices, the pull-up resistor is connected to D-. In this way, when the device is inserted into the hub, the 1.5k pull-up resistor and the 15k pull-down resistor are used to pull one of the differential data lines high. After the hub detects this state, it reports to the USB host controller (or reports to the USB host controller through the hub above it), so that the insertion of the device is detected. The USB high-speed device is first identified as a full-speed device, and then switched to the high-speed mode after confirmation between the HOST and DEVICE. In the high-speed mode, it is the current transmission mode, and the pull-up resistor on D+ is disconnected at this time.
The four major transmission types of USB:
Control transfer
It is a must-have for every USB device and is usually used to obtain device descriptors, set device status, etc. The process of inserting a USB device and finally removing it will definitely generate control transfer (even if the USB device is not supported by the system).
Interrupt transfer
Typical devices that support interrupt transmission include USB mouse, USB keyboard, etc. Interrupt transmission does not mean that my device actually sends an interrupt, and then the host will read the data. It is actually a polling method to complete data communication. The USB device will set a parameter called interval in the device driver, which is a member of the endpoint. Interval means the interval time, which means how long my device wants the host to poll itself. Once this value is determined, my host will periodically check if there is any data to be processed.
Batch Processing
The most typical device that supports batch transfer is the USB flash drive, which can transfer large amounts of data and ensure the accuracy of the data, but the time is not fixed.
Real-time transmission
USB cameras are typical representatives of real-time transmission devices. They also transmit large amounts of data. The accuracy of the data cannot be guaranteed, but they are very sensitive to transmission delays, which means that they have high real-time requirements.
USB endpoint: (equivalent to us speaking through the "mouth")
The USB device and the host will have several communication "endpoints", each endpoint has an endpoint number. Except for endpoint 0, each endpoint can only work in one transfer type (control transfer, interrupt transfer, bulk transfer, real-time transfer) and one transfer direction.
The transmission direction is based on the standpoint of the USB host.
For example: the mouse data is transmitted from the mouse to the PC, and the corresponding endpoint is called the "interrupt input endpoint"
Endpoint 0 is the default control endpoint of the device, which can be used for both output and input and is used in the identification process of USB devices.
The Linux kernel also comes with its own USB bus driver, framework breast cancer:
To become a USB host, the hardware must have a USB host controller, which is divided into 4 types of interfaces:
OHCI (Open Host Controller Interface): Low-speed USB1.0 (1.5Mbps) and full-speed USB1.1 (12Mbps) led by Microsoft. The OHCI interface has simple software and complex hardware.
UHCI (Universal Host Controller Interface): Intel-led low-speed USB1.0 (1.5Mbps) and full-speed USB1.1 (12Mbps). The UHCI interface has complex software and simple hardware.
EHCI (Enhace Host Controller Interface): High-speed USB2.0 (480Mbps)
xHCI (eXtensible Host Controller Interface): USB3.0 (5.0Gbps), uses a 9-pin configuration, and also supports USB2.0, 1.1, etc.
Next, let's get into the topic and start analyzing the USB bus driver and how to identify the USB device.
Since the kernel comes with a USB driver, we first plug a USB keyboard into the development board to see the print information
The following fields were found:
As shown below, the first paragraph is located in line 2186 of drivers/usb/core/hub.c
This hub is actually the hub of our USB host controller, which is used to manage multiple USB interfaces.
1. Line 2186 of drivers/usb/core/hub.c is in the hub_port_init() function
Who calls it? As shown in the figure below, we searched and found that it was called by the hub_thread() function.
The hub_thread() function is as follows:
static int hub_thread(void *__unused)
{
do {
hub_events(); //Execute page hub time function
wait_event_interruptible(khubd_wait,
!list_empty(&hub_event_list) ||
kthread_should_stop());//Each time a hub event is executed, it will enter a waiting event interrupt function
try_to_freeze();
} while (!kthread_should_stop() || !list_empty(&hub_event_list));
pr_debug("%s: khubd exitingn", usbcore_name);
return 0;
}
From the above function, we can see that in order to execute hub_event(), we have to wait for the khubd_wait interrupt to wake up.
2. We search for "khubd_wait" to see who wakes it up.
Find the interrupt wakeup in the kick_khubd() function, the code is as follows:
static void kick_khubd(struct usb_hub *hub)
{
unsigned long flags;
/* Suppress autosuspend until khubd runs */
to_usb_interface(hub->intfdev)->pm_usage_cnt = 1;
spin_lock_irqsave(&hub_event_lock, flags);
if (list_empty(&hub->event_list)) {
list_add_tail(&hub->event_list, &hub_event_list);
wake_up(&khubd_wait); //Wake up khubd_wait interrupt
}
spin_unlock_irqrestore(&hub_event_lock, flags);
}
3. Continue searching for kick_khubd and find that it is called by the hub_irq() function
Obviously, when a USB device is plugged in, D+ or D- will be pulled high, and then the USB host controller will generate a hub_irq interrupt
4. Next, we will directly analyze the hub_port_connect_chage() function to see how to connect to the port.
static void hub_port_connect_change(struct usb_hub *hub, int port1,
u16 portstatus, u16 portchange)
{
... ...
udev = usb_alloc_dev(hdev, hdev->bus, port1); //(1)Register usb_device and then put it on the USB bus
usb_set_device_state(udev, USB_STATE_POWERED); //Set the status flag of the registered USB device
... ...
choose_address(udev); //(2) Give the new device an address number
status = hub_port_init(hub, udev, port1, i); //(3)Initialize the port and establish a connection with the USB device
... ...
status = usb_new_device(udev); //(4) Create a USB device and connect it to the USB device driver
}
(usb_device device structure reference: https://mp.csdn.net/postedit/81025137)
So the final flow chart is as follows:
5. Let's go to hub_port_connect_change()->usb_alloc_dev() to see how it sets up usb_device
struct usb_device * usb_alloc_dev(struct usb_device *parent, struct usb_bus *bus, unsigned port1)
{
struct usb_device *dev;
dev = kzalloc(sizeof(*dev), GFP_KERNEL); // allocate a usb_device device
... ...
device_initialize(&dev->dev);//initialize usb_device
dev->dev.bus = &usb_bus_type; //(1) Set the member device->bus of usb_device to be equal to the usb_bus_type bus
dev->dev.type = &usb_device_type; //(2) Set the member device->type of usb_device to be equal to usb_device_type
...
return dev; //Return a usb_device structure
}
(1) Set the device member bus, mainly twenty sections, to register the device table of the USB bus.
Among them, usb_bus_type is a global variable, which is similar to the platform bus we learned before. It belongs to the USB bus and is a type of bus in Linux.
As shown in the figure below, every time a USB device or a USB device driver is created, the USB bus will call the match member to match once to associate the USB device and the USB driver.
The usb_bus_type structure is as follows:
struct bus_type usb_bus_type = {
.name = "usb", // bus name, stored in /sys/bus
.match = usb_device_match, //Matching function, if the match is successful, the probe function member of the usb_driver driver will be called
.uevent = usb_uevent, // event function
.suspend = usb_suspend, // sleep function
.resume = usb_resume, //Wake-up function
};
6. Let's go to hub_port_connect_change()->choose_address() to see how it assigns address numbers
static void choose_address(struct usb_device *udev)
{
int devnum;
struct usb_bus *bus = udev->bus;
devnum = find_next_zero_bit(bus->devmap.devicemap, 128,bus->devnum_next); //In the range of bus->devnum_next~128, loop to find the next non-zero (no device) number
if (devnum >= 128) //If the address number is greater than or equal to 128, it means that no free address number is found, and the search starts from the beginning
Previous article:S3C2440 USB mouse driver (19)
Next article:S3C2440 touch screen driver (Seventeen)
Recommended ReadingLatest update time:2024-11-16 08:30
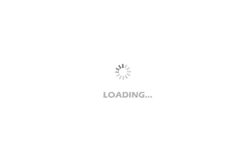
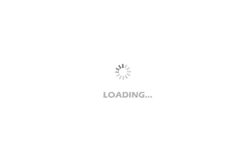
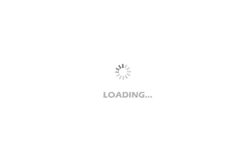
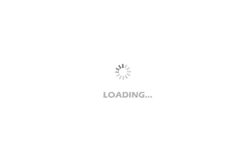
- Popular Resources
- Popular amplifiers
-
Virtualization Technology Practice Guide - High-efficiency and low-cost solutions for small and medium-sized enterprises (Wang Chunhai)
-
usb_host_device_code
-
Image acquisition and processing system for panoramic map applications
-
Teach you to learn 51 single chip microcomputer-C language version (Second Edition) (Song Xuefeng)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Introduction to Common PCB Microvia Technology
- Analysis of the design principle of single chip microcomputer music program
- Beetle_ESP32-C3 Arduino routine learning
- [BearPi-HM Nano, play Hongmeng's "touch and pay" function] Part 4: Combination of OLED and UDP!
- IPC video acquisition and AI issues
- FPGA key debounce
- Robot programming design and implementation
- SVPWM Vector Control System of Induction Motor Based on DSP
- 【RT-Thread Reading Notes】Virtual File System
- Read the good book "Electronic Engineer Self-study Handbook" + Reflection