Table of contents:
1. Program: Timer 2 interrupt configuration, high-precision delay function and LED light initialization
2. Basic knowledge:
1. Procedure:
#include #include "timer.h" #include "led.h" //unsigned int ms_count=0;//millisecond count //Timer 2 initialization void InitTIM2() { TIM2_PSCR=0x00; //1 division, timer clock equals system clock = 16m TIM2_ARRH=0X3e; //Be sure to install the upper eight bits first, then the lower eight bits TIM2_ARRL=0X80; //1ms reload value 16000, this is a pitfall, the technical manual says that tim2 counts up, but it actually counts down TIM2_CNTRH=0x00; TIM2_CNTRL=0x00; //It is necessary to clear the down counter TIM2_IER=0X01; //Enable tim2 update interrupt TIM2_SR1=0X01; //Clear tim2 update interrupt flag TIM2_CR1=0X81; //Allow reloading, enable timer } #pragma vector=0xF //Set the interrupt vector number of timer 2 reload = 0xF, this is the format of the interrupt, just call it directly __interrupt void TIM2_UPD_OVF_BRK_IRQHandler(void) { TIM2_SR1=0x00; ms_count++; if(ms_count>=1|ms_count<=600) { PB4_out = 0; /* PD0 pin output 0 */ PB5_out = 1; } if(ms_count>=600)//1ms*1000=1s { PB4_out = 1; /* PD0 pin output 0 */ PB5_out = 0; } if(ms_count>=1200) {ms_count=0;} } int main(void) { CLK_CKDIVR=0x00;/*Set the clock to the internal 16M high speed clock*/ asm("sim"); //Disable total interrupt InitTIM2(); //Timer 2 initialization LED_conf(); //LED initialization asm("rim"); // Enable total interrupt __enable_interrupt(); while(1) { // LED_Display_Bit(); } } /*LED light initialization*/ void LED_conf(void) { //PB5、PB4 PB_DDR|=0x30; /* Set the data direction register 1 to output and 0 to input -- see STM8 register.pdf P87 */ PB_CR1|=0x30; /* Set push-pull output--see STM8 register.pdf P88 */ PB_CR2|=0x00; /* Set the output frequency 1 to 10M, 0 to 2M--See STM8 register.pdf P89 */ } void LED_Display_Bit() { PB4_out = 0; /* PD0 pin output 0 */ PB5_out = 1; Delayms(500); PB4_out = 1; /* PD0 pin output 1 */ PB5_out = 0; Delayms(500); } /*Delay function*/ #include #include "timer.h" void ClkInit(void) { CLK_ECKR |=0x1; //Turn on external clock while(!(CLK_ECKR&0x2)); //Wait for external clock rdy CLK_CKDIVR &= 0xF8; //CPU has no frequency division 16M CLK_SWR = 0xB4; //Select external clock while(!(CLK_SWCR&0x8)); CLK_SWCR |= 0x2; // Enable external clock } //--- Microsecond delay-------------------------- void Delayus(void) { asm("nop"); //An asm("nop") function is tested by an oscilloscope and represents 100ns asm("nop"); asm("nop"); asm("nop"); } //----Millisecond delay program----------------------- void Delayms(unsigned int time) { unsigned int i; while(time--) for(i=900;i>0;i--) DelayUse(); } 2. Basic knowledge: 1. Timer 2 Introduction: General-purpose timers TIM2, TIM3, TIM5; general-purpose timers can choose 16 frequency divisions, and the timers all count down. 2. The registers used for basic interrupt timing are as follows; (1) Automatically load register high (TIMx_ARRH) and automatically load register low (TIMx_ARRL) The general timers TIM2, TIM3, and TIM5 are all 16-bit counting timers. When operating the register, you must first write the upper 8 bits and then the lower 8 bits. When operating the reload register, the updated value will not be written into the reload register immediately, but the value will be written into the register when an interrupt is generated. Of course, you can also use software methods to generate interrupts. (2) Counter high (TIMx_CNTRH) and counter low (TIMx_CNTRL) The general timers TIM2, TIM3, and TIM5 use these two counters. (3) Interrupt enable register (TIMx_IER) Address offset value: 0x04 Reset value: 0x00 7-0: BIE TIE COMIE CC4IE CC3IE CC2IE CC1IE UIE Bit 7 BIE: Enable brake interrupt 0: Disable brake interruption; 1: Allow brake interruption. Bit 6 TIE: Trigger interrupt enable 0: Disable triggering interrupt; 1: Enable trigger interrupt. Bit 5 COMIE: Enable COM interrupt 0: Disable COM interrupt; 1: Enable COM interrupt. Bit 4 CC4IE: Enable capture/compare 4 interrupt 0: Disable capture/compare 4 interrupt; 1: Enable capture/compare 4 interrupt. Bit 3 CC3IE: Enable capture/compare 3 interrupt 0: Disable capture/compare 3 interrupt; 1: Enable capture/compare 3 interrupt. Bit 2 CC2IE: Enable capture/compare 2 interrupt 0: Disable capture/compare 2 interrupt; 1: Enable capture/compare 2 interrupt. Bit 1 CC1IE: Enable capture/compare 1 interrupt 0: Disable capture/compare 1 interrupt; 1: Enable Capture/Compare 1 interrupt. Bit 0 UIE: Enable update interrupt 0: Disable update interrupt; 1: Allow update interruption. (4) Status register 1 (TIMx_SR1) Address offset value: 0x05 Reset value: 0x00 7-0: BIF TIF COMIF CC4IF CC3IF CC2IF CC1IF UIF Bit 7 BIF: Brake interrupt flag Once the brake input is valid, the hardware sets this bit to 1. If the brake input is invalid, the software can clear this bit to 0. 0: No braking event occurs; 1: A valid level is detected on the brake input. Bit 6 TIF: Trigger interrupt flag Set to 1 by hardware when a trigger event occurs (a valid edge detected at the TRGI input when the slave mode controller is in a mode other than gated mode, or any edge in gated mode). It is cleared to 0 by software. 0: No trigger event is generated; 1: Trigger interrupt and wait for response. Bit 5 COMIF: COM interrupt flag This bit is set to 1 by hardware once a COM event occurs (when the capture/compare control bits: CCiE, CCiNE, OCiM have been updated). Cleared by software. 0: No COM event is generated; 1: COM interrupt waiting for response. Bit 4 CC4IF: Capture/Compare 4 interrupt flag Refer to CC1IF description. Bit 3 CC3IF: Capture/Compare 3 interrupt flag Refer to CC1IF description. Bit 2 CC2IF: Capture/Compare 2 interrupt flag Refer to CC1IF description. Bit 1 CC1IF: Capture/Compare 1 interrupt flag If channel CC1 is configured as output mode: This bit is set to 1 by hardware when the counter value matches the compare value, except in centrosymmetric mode (refer to the CMS bit in the TIM1_CR1 register). It is cleared to 0 by software. 0: No match occurs; 1: The value of TIMx_CNT matches the value of TIMx_CCR1. Note: In centrosymmetric mode, when the counter value is 0, it counts up, and when the counter value is ARR, it counts down (it counts up from 0 to ARR-1, and then counts down from ARR to 1). Therefore, for all SMS bit values, neither value is marked. However, if CCR1>ARR, then CC1IF is set to 1 when CNT reaches the ARR value. If channel CC1 is configured as input mode: This bit is set by hardware when a capture event occurs and is cleared by software or by reading TIM1_CCR1L. 0: No input capture is generated; 1: The counter value has been captured (copied) to TIM1_CCR1 (an edge with the same polarity as selected was detected on IC1). Bit 0 UIF: Update interrupt flag This bit is set to 1 by hardware when an update event occurs. It is cleared to 0 by software. 0: No update event is generated; 1: Update event waiting for response. This bit is set by hardware when the register is updated: − If UDIS=0 in the TIM1_CR1 register, when the counter overflows or underflows; − If UDIS=0 and URS=0 in the TIM1_CR1 register, when the UG bit in the TIM1_EGR register is set, the software will When CNT is reinitialized; − If UDIS=0, URS=0 of the TIM1_CR1 register, when the counter CNT is reinitialized by a trigger event (refer to 0 Slave mode control register TIM1_SMCR). (5) Control register 1 (TIMx_CR1) Address offset value: 0x00 Reset value: 0x00 7-0: ARPE CMS[1:0] DIR OPM URS UDIS CEN Bit 7 ARPE: Automatic preload enable bit 0: TIM1_ARR register is not buffered, it can be written directly; 1: TIM1_ARR register is buffered by the preload buffer. Bit 6:5 CMS: Select center alignment mode 00: Edge-aligned mode. The counter counts up or down according to the direction bit (DIR). 01: Center alignment mode 1. The counter counts up and down alternately. The output compare interrupt flag of the channel configured as output (CCiS=00 in the TIM1_CCMRx register) is set to 1 only when the counter counts down. 10: Center-aligned mode 2. The counter counts up and down alternately. The output compare interrupt flag of the channel configured as output (CCiS=00 in the TIM1_CCMRx register) is set to 1 only when the counter counts up. 11: Center-aligned mode 3. The counter counts up and down alternately. The output compare interrupt flag of the channel configured as output (CCiS=00 in the TIM1_CCMRx register) is set to 1 when the counter counts up and down. Note 1: When the counter is enabled (CEN=1), transition from edge-aligned mode to center-aligned mode is not allowed. Note 2: In center-aligned mode, the encoder mode (SMS=001, 010, 011 in GPT_SMCR register) must Prohibited. Bit 4 DIR: Direction 0: counter counts up; 1: The counter counts down. Note: This bit is read-only when the counter is configured in center-aligned mode or encoder mode. Bit 3 OPM: Single pulse mode 0: The counter does not stop when an update event occurs; 1: The counter stops at the next update event (clearing the CEN bit). Bit 2 URS: Update request source 0: If UDIS allows update events to be generated, any of the following events will generate an update interrupt: − Registers are updated (counter overflow/underflow) − Software setting UG bit − Updates generated by the clock/trigger controller 1: If UDIS allows update events to be generated, an update interrupt is generated and UIF is set to 1 only when the following events occur: − Registers are updated (counter overflow/underflow) Bit 1 UDIS: Disable update 0: Generate an update (UEV) event once the following events occur: − Counter overflow/underflow − Generate software update events − Hardware reset generated by the clock/trigger mode controller The cached registers are loaded with their preload values. 1: No update event is generated, and the shadow registers (ARR, PSC, CCRx) retain their values. The counter and prescaler are reinitialized when the clock/trigger controller issues a hardware reset.
Previous article:STM8SF903K3T6 Timer 1 input capture
Next article:STM8S: Debugging is normal, but burning program is not running normally
Recommended ReadingLatest update time:2024-11-15 14:45
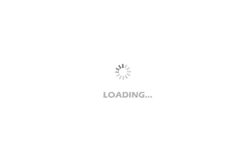
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
- Can vias be placed on pads?
- AM26LV32E Low Voltage High Speed Quadruple Differential Line Receiver
- Is a 12 bit oscilloscope really necessary?
- 5G Science (Comic Version, So Easy!)
- Nordic Bluetooth ICnRF52832 and 52840 Differences
- [SAMR21 New Gameplay] 3. Introduction to Graphical Programming
- Design of PCIJMC2000 Computer Data Encryption Card Based on DSP
- Review Weekly: New arrivals, Qinheng wireless charging kit, Beineng cost-effective ATSAMD51 board are waiting for you~20221107
- 12. [Learning LPC1768 library functions] PWM experiment
- How to Actually Build a Drone