This is my first time using a PIC microcontroller. I don't know the programming specifications for PICC, and I always have problems when compiling programs. Now I will introduce two of the most common problems to you to see if you have encountered the same problems as me.
Error 1: The order of variable definition and assignment is very important
The development environment is MPLAB IDE V7.43 + PICC. Which of the following two code writing methods will compile incorrectly after completion? (flag_sendKeyByte is a global bit variable, which has been defined)
A:
flag_sendKeyByte = 0;
unsigned char mbit = 0;
B:
unsigned char mbit = 0;
flag_sendKeyByte = 0;
From the perspective of normal C language habits, it seems that there is no problem, they are all assignments, just the order is different. However, in the compilation environment of PIC microcontroller, A is wrong and B is correct.
When this compilation error occurred, I searched for a long time. Since it was my first time to use PIC and I didn't know much about it, I took a lot of detours. Finally, I deleted the code line by line and modified it line by line, and finally found that the order was wrong.
The compilation environment of PIC microcontrollers requires that variable assignment must be after variable definition. It does not allow defining another variable and assigning a value after assigning a value to a variable. It seems that the compilation environment processes all variable definitions before assigning a value. If a variable assignment statement is detected, the definition of the function or subroutine will not be checked, resulting in some variables being undefined during the final compilation.
(Note: The above analysis is my own thinking, and may be wrong. Please correct me if I am wrong.)
The funny thing is that the compilation error message is a mess, instead of saying that there are undefined variables. The following prompts are what I prompted when compiling. For a beginner, can you find the source of the error based on these prompts?
Error[000] D:documentsPICtestkeyboardps2.c 390 : missing basic type: int assumed Error
[000] D:documentsPICtestkeyboardps2.c 390 : type redeclared
Error[000] D:documentsPICtestkeyboardps2.c 390 : identifier redefined: calc_parity (from line 64) Error[000] D:documentsPICtestkeyboardps2.c 390 : constant expression required
Warning[000] D:documentsPICtestkeyboardps2.c 390 : missing basic type: int assumed
Error[000] D:documentsPICtestkeyboardps2.c 390 : type redeclared
Error[000] D:documentsPICtestkeyboardps2.c 390 : identifier redefined: calc_parity (from line 64)
Error[000] D:documentsPICtestkeyboardps2.c 390 : constant expression required
....
There are many more to come, and the program will prompt an error at the end. Replace the writing in A with that in B, and the compilation will succeed.
I feel that this compilation environment is too difficult to use. I have used the development tools of the C8051F series before, which are much easier to use. I raise this question to remind beginners not to make the same mistake as me.
Error 2, could not find file 'c018i.o'
When using MPIDE +MCC18 to compile, the following prompt often appears: "could not find file 'c018i.o'"
Solution: Because the default path of the library is not specified. Click project->build option->project. In the Directories tab, click the drop-down menu on the right of show directories for:, find the library search path item, select new, and specify the path as c:mcc18lib in the newly created project. Click OK. Compile again and it will be successful.
————————————————————————————————
In C language, variable declarations must be at the beginning of a block.
A block refers to the part enclosed by a pair of {} braces.
The variable declaration must follow "{" immediately, and no other code can be included in between.
For example, for(char i=0; i< 20 ; i++){ do something...}, which works in some compilers but not in others.
This way of writing is also incorrect and will report a syntax error, an error of undeclared variable.
If you want to use it but don’t want to declare the temporary variable i at the beginning of the block, you can do it like this:
{ //Note the curly braces
char i;
for(i=0; i< 20 ; i++){ /*do something...*/}
} //Note the curly braces.
This ensures that the declaration is at the beginning of the block and that the local variable can be used without declaring it at the beginning
Previous article:MOS tube makes PIC microcontroller unable to operate normally
Next article:PIC16F877A Watchdog Timer (WDT)
Recommended ReadingLatest update time:2024-11-23 08:18
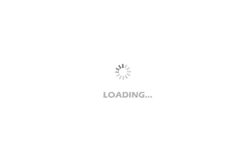
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- RISC-V Manual Chinese Version
- AUTOSEA--Medium and high frequency noise and vibration simulation analysis solution
- Ethernet plays an important role in the manufacturing industry
- Switching Power Supply Interest Group Task 02
- Say goodbye to 2018 and welcome 2019. See how the three pillars of China Mobile IoT dominate the field
- Using MakeCode Driver on BrainPad Development Board
- Car Radio Audio Signal Processor (Front End)
- Please tell me the function of STM32 WWDG window value
- How does positioning technology change the world?
- 2. Sensors used in previous "Control" competitions