My roommate always forgets to bring his keys, so I made a simple fingerprint lock. Six people in the dormitory use it. This is the first time I upload it. It's quite crude. I wrote a very detailed comment (I think).
If it can help you, it will be great.
The microcontroller source program is as follows:
#include #include sbit zhen=P2^0; //The motor rotates forward, pull the handle to open the door sbit fan=P2^1; //motor reverses, resets door handle sbit led_work=P2^2; //MCU has completed initialization, indicating LED and detecting door handle position indicator sbit check=P2^3; //Reed switch position detection, door open is 1 sbit pressed=P2^6; //Fingerprint module touch detection sbit wakeup=P2^7; //Fingerprint module power-on control, 1 means the module is powered on, 0 means it is powered off sbit test=P3^3; //Test door opening ////////////////////////////////////////////////////////////////////////// volatile unsigned char FPM10A_RECEICE_BUFFER[32]; //define the receive buffer code unsigned char FPM10A_Pack_Head[6] = {0xEF,0x01,0xFF,0xFF,0xFF,0xFF}; //Protocol header code unsigned char FPM10A_Get_Img[6] = {0x01,0x00,0x03,0x01,0x00,0x05}; //Get fingerprint image code unsigned char FPM10A_Img_To_Buffer1[7]={0x01,0x00,0x04,0x02,0x01,0x00,0x08}; //Put the image into BUFFER1 code unsigned char FPM10A_Search[11]={0x01,0x00,0x08,0x04,0x01,0x00,0x00,0x00,0x64,0x00,0x72}; //Search fingerprint search range 0 - 999, use the signature in BUFFER1 to search ////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////// // Timing // ///////////////////////////////////////////// void delay1s(void) //timing 1S, crystal oscillator 11.0592MHZ { unsigned char a,b,c; for(c=13;c>0;c--) for(b=247;b>0;b--) for(a=142;a>0;a--); _nop_(); //if Keil,require use intrins.h } void delay100ms(void) //timing 100MS, crystal 11.0592MHZ { unsigned char a,b; for(b=221;b>0;b--) for(a=207;a>0;a--); } void delay500ms(void) //500ms { unsigned char a,b,c; for(c=98;c>0;c--) for(b=127;b>0;b--) for(a=17;a>0;a--); _nop_(); } void delay1_6_f(void) //error 0us { unsigned char a,b,c; for(c=218;c>0;c--) for(b=131;b>0;b--) for(a=23;a>0;a--); _nop_(); //if Keil,require use intrins.h } void delay50ms(void) //error 0us { unsigned char a,b; for(b=173;b>0;b--) for(a=143;a>0;a--); } void delay10ms(void) //error 0us { unsigned char a,b,c; for(c=1;c>0;c--) for(b=38;b>0;b--) for(a=130;a>0;a--); } ///////////////////////////////////////////// void kaisuo(void) { int t; zhen=fan=0; zhen=1; //Motor starts forward for(t=0;t<45;t++) //50ms is one segment, totaling 2.25s, to control the maximum pulling time to prevent the handle from being damaged by accidental forward rotation for too long { if(check==0) { delay10ms(); //Wait for 10ms, the reed switch will debounce if(check==0) //check again { led_work=0; // detect that the handle is in place, the indicator light turns off to indicate zhen=0; //motor stop delay100ms(); //Wait 100ms, prepare for reversal //delay500ms(); //Wait for 500ms, prepare for reversal fan=1; delay1_6_f(); //Reverse 1.6s fan=0; led_work=1; //status indicator light reset check=1; return; //Break out of the loop } } else { delay50ms(); //50ms per segment continue; } } zhen=0; //Time exceeded, shutdown delay100ms(); //Wait 100ms, prepare for reversal //delay500ms(); //Wait 500ms, prepare for reversal fan=1; delay1_6_f(); fan=0; } void Uart_Init(void) //initialization { //zhen=1; //fan=1; SCON=0x50; //UART mode 1: 8-bit UART; REN=1: Allow receiving PCON=0x00; //SMOD=0: baud rate is not doubled TMOD=0x20; //T1 mode 2, used for UART baud rate TH1=0xFD; //UART baud rate setting: FDFD(9600) TL1=0xFD; //UART baud rate setting: FDFD(9600) TR1=1; //Allow T1 counting EA=1; // } ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// //UART sending and receiving part ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// void Uart_Send_Byte(unsigned char c)//uart sends a byte { SBUF = c; while(!TI); //1 after sending TI = 0; } unsigned char Uart_Receive_Byte() //UART receives a byte { unsigned char dat; while(!RI); //1 after receiving RI = 0; dat = SBUF; return (dat); } /////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// //////////////////////////////////////////// //AS608/FPM10A fingerprint module command // //////////////////////////////////////////// void FPM10A_Cmd_Send_Pack_Head(void) //Send communication protocol packet header { int i; for(i=0;i<6;i++) { Uart_Send_Byte(FPM10A_Pack_Head[i]); } } void FPM10A_Receive_Data(unsigned char ucLength) //Receive fingerprint module feedback data buffer { unsigned char i; for (i=0;i } void FPM10A_Cmd_Get_Img(void) ////FINGERPRINT_Get fingerprint image command (detect whether there is a fingerprint) { unsigned char i; FPM10A_Cmd_Send_Pack_Head(); //Send communication protocol packet header for(i=0;i<6;i++) { Uart_Send_Byte(FPM10A_Get_Img[i]); } } //Convert the image into a feature code and store it in Buffer1 void FINGERPRINT_Cmd_Img_To_Buffer1(void) { unsigned char i; FPM10A_Cmd_Send_Pack_Head(); //Send communication protocol packet header for(i=0;i<7;i++) //Send command to convert image into feature code and store it in CHAR_buffer1 { Uart_Send_Byte(FPM10A_Img_To_Buffer1[i]); } } //Search the first 100 fingerprints in the database (you can change the number in the DATA area up to 999) void FPM10A_Cmd_Search_Finger(void) { unsigned char i; FPM10A_Cmd_Send_Pack_Head(); //Send communication protocol packet header for(i=0;i<11;i++) { Uart_Send_Byte(FPM10A_Search[i]); //Receive the data sent back by the fingerprint module } } //Search for fingerprints, and authenticate if there are any void FPM10A_Find_Fingerprint() { FPM10A_Cmd_Get_Img(); //Send command to get fingerprint image FPM10A_Receive_Data(12); //Receive feedback data buffer if(FPM10A_RECEICE_BUFFER[9]==0&&pressed==1) //Based on the 9th bit of data fed back, determine whether there is a fingerprint on the module. If yes, continue to execute. If no, exit { FINGERPRINT_Cmd_Img_To_Buffer1(); //Convert the image into feature code and store it in Buffer1 FPM10A_Receive_Data(12); FPM10A_Cmd_Search_Finger(); //Search all users 100 pieces FPM10A_Receive_Data(16); if(FPM10A_RECEICE_BUFFER[9] == 0) //If the corresponding fingerprint is found { kaisuo(); //Unlock //delay500ms(); //delay 0.5s delay1s(); wakeup=0; //Module SOC power off } else { wakeup=0; //Module SOC power off //delay100ms(); //delay 100ms, jump out } } else { wakeup=0; //Module SOC power off } } ////////////////////////////////////////////// // Main program // ////////////////////////////////////////////// void main() { //delay1s(); //MCU powers on and waits for 1s to stabilize Uart_Init(); //Initialize the serial port led_work=0; zhen=0; fan=0; wakeup=0; pressed=0; test=1; check=1; //Detection position reed switch led_work=1; //The working indicator light is on, reminding the system that the initialization has been completed (test is 0) while(1) { if(pressed==1) //Is the fingerprint module pressed? If pressed, it is 1; otherwise, it is 0 { //Uart_Init(); wakeup=1; //Module SOC power on delay500ms(); //delay 0.5s //wait for SOC initialization to complete Uart_Init(); //Reinitialize the serial port FPM10A_Find_Fingerprint(); //Search and compare fingerprints } else { if(test==0) //test unlock { kaisuo(); //Unlock delay500ms(); //delay 0.5s } else { delay100ms(); //The fingerprint module is not pressed and the delay is 100ms } } } }
Previous article:HX711 electronic scale weighing module distribution information (MCU source code with median filter)
Next article:Design of automatic watering greenhouse control system based on single chip microcomputer
Recommended ReadingLatest update time:2024-11-16 13:59
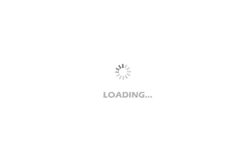
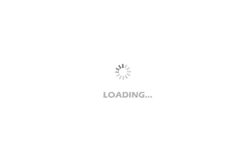
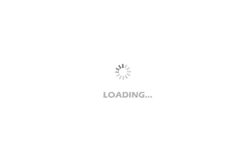
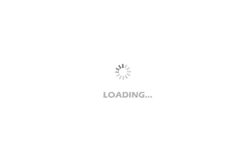
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Fundamentals and Applications of Single Chip Microcomputers (Edited by Zhang Liguang and Chen Zhongxiao)
-
Single chip microcomputer control technology (Li Shuping, Wang Yan, Zhu Yu, Zhang Xiaoyun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Importing an old MPLAB IDE V8.9 project into MPLAB X IDE shows 'other' has different root
- [Smartwatch for environmental experts] Part 8: Code migration for personnel going down the mine
- Post count 1234, mark it here
- Open Source ESP32 Color Screen WIFI/BLE Smart Multimeter Production Process (1. Shell and Screen Selection)
- TI Automotive IC Products and Application Solutions (Free Viewing)
- B station hands-on expert shares: How to "trick" USB charger
- Differentiated innovative product-LED transparent screen
- TI's affordable Li-ion battery pack reference design for electric motorcycle batteries
- EEWORLD University Hall----Live Replay: Manufacturing Logistics Challenges of Microchip Key Security Configuration- Advantages of Discrete Security Elements
- Please tell me what is the reason for this, the transistor is connected to the single chip